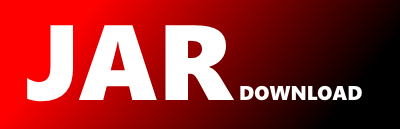
org.qas.qtest.api.services.project.model.Module Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.project.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import org.qas.qtest.api.internal.model.QTestBaseModel;
import java.util.List;
/**
* Module
*
* @author Dzung Nguyen
* @version $Id Module 2014-05-17 10:14:30z dungvnguyen $
* @since 1.0
*/
public final class Module extends QTestBaseModel {
@JsonProperty("id")
private Long id;
@JsonProperty("parent_id")
private Long parentId;
@JsonProperty("name")
private String name;
@JsonProperty("shared")
private Boolean shared;
@JsonProperty("pid")
private String pid;
@JsonProperty("order")
private Integer order;
@JsonProperty("description")
private String description;
@JsonProperty("children")
private List children;
/**
* Creates module instance.
*/
public Module() {
setParentId(0L);
}
/**
* @return the module identifier.
*/
public Long getId() {
return id;
}
/**
* Sets module identifier.
*
* @param id the given module identifier to set.
* @return current instance.
*/
public Module setId(Long id) {
this.id = id;
return this;
}
/**
* Sets module identifier and return the current module instance.
*
* @param id the given module identifier to set.
* @return the current module instance.
*/
public Module withId(Long id) {
setId(id);
return this;
}
/**
* @return the parent identifier of module.
*/
public Long getParentId() {
return parentId;
}
/**
* Sets the parent identifier of module.
*
* @param parentId the given parent identifier of module to set.
* @return current instance.
*/
public Module setParentId(Long parentId) {
this.parentId = parentId;
return this;
}
/**
* Sets the parent identifier of module and return the current module
* instance.
*
* @param parentId the given parent identifier of module to set.
* @return the current module instance.
*/
public Module withParentId(Long parentId) {
setParentId(parentId);
return this;
}
/**
* @return the module name.
*/
public String getName() {
return name;
}
/**
* Sets the module name.
*
* @param name the given module name to set.
* @return current instance.
*/
public Module setName(String name) {
this.name = name;
return this;
}
/**
* Sets the module name.
*
* @param name the given module name to set.
* @return the current module instance.
*/
public Module withName(String name) {
setName(name);
return this;
}
/**
* @return the shared flag.
*/
public Boolean getShared() {
return shared;
}
/**
* Sets the module shared flag.
*
* @param shared the given module shared flag.
* @return current instance.
*/
public Module setShared(Boolean shared) {
this.shared = shared;
return this;
}
/**
* Sets the module shared flag.
*
* @param shared the given shared flag.
* @return the current module instance.
*/
public Module withShared(Boolean shared) {
setShared(shared);
return this;
}
/**
* @return the display identifier of module.
*/
public String getPid() {
return pid;
}
/**
* Sets the display identifier of module.
*
* @param pid the given display identifier of module to set.
* @return current instance.
*/
public Module setPid(String pid) {
this.pid = pid;
return this;
}
/**
* Sets the display identifier of module and return itself.
*
* @param pid the given display identifier of module to set.
* @return the current module instance.
*/
public Module withPid(String pid) {
setPid(pid);
return this;
}
/**
* @return the module order.
*/
public Integer getOrder() {
return order;
}
/**
* Sets the module order.
*
* @param order the given module order to set.
* @return current instance.
*/
public Module setOrder(Integer order) {
this.order = order;
return this;
}
/**
* Sets the module order and return itself.
*
* @param order the given module order to set.
* @return the module instance.
*/
public Module withOrder(Integer order) {
setOrder(order);
return this;
}
/**
* @return the module description.
*/
public String getDescription() {
return description;
}
/**
* Sets module description.
*
* @param description the given module description to set.
* @return current instance.
*/
public Module setDescription(String description) {
this.description = description;
return this;
}
/**
* Sets module description.
*
* @param description the given module description to set.
* @return current instance.
*/
public Module withDescription(String description) {
setDescription(description);
return this;
}
/**
* @return the list of module's children.
*/
public List getChildren() {
return children;
}
/**
* Sets the list of module's children.
*
* @param modules the given list of module's children.
* @return current instance.
*/
public Module setChildren(List modules) {
this.children = modules;
return this;
}
/**
* Sets the list of module's children.
*
* @param modules the given list of module's children.
* @return the current module.
*/
public Module withChildren(List modules) {
setChildren(modules);
return this;
}
@Override
public Module clone() {
Module that = new Module();
that.setPropertiesFrom(this);
return that;
}
@Override
public String elementName() {
return "module";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy