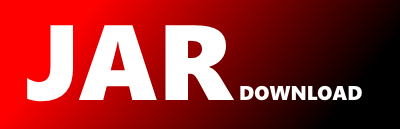
org.qas.qtest.api.services.project.model.Permissions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.project.model;
import com.fasterxml.jackson.annotation.JsonIgnore;
import org.qas.qtest.api.internal.model.QTestBaseModel;
import java.util.Map;
/**
* Permissions
*
* @author Dzung Nguyen
* @version $Id Permissions 2014-10-30 12:00:30z dungvnguyen $
* @since 1.0
*/
public final class Permissions extends QTestBaseModel {
@JsonIgnore
private PermissionType type;
//~ class members ===========================================================
/**
* Creates the permission instance.
*/
public Permissions() {
}
/**
* @param permissions permissions
*/
public Permissions(Map permissions) {
if (null != permissions) {
this.properties.putAll(permissions);
}
}
/**
* If the given permission name is permitted
*
* @param name the given permission name.
* @return {@code true} if permitted, otherwise {@code false}
*/
public boolean hasPermission(String name) {
Object value = getProperty(name);
if (value == null || !(value instanceof Boolean)) return false;
return ((Boolean) value).booleanValue();
}
/**
* Sets the permission.
*
* @param name the given permission name.
* @param value the value of the given permission.
* @return current instance.
*/
public Permissions setPermission(String name, Boolean value) {
if (value == null) {
removeProperty(name);
} else {
setProperty(name, value);
}
return this;
}
/**
* Sets the permission.
*
* @param name the given permission name.
* @param value the value of the given permission.
* @return the {@link Permissions} object.
*/
public Permissions withPermission(String name, Boolean value) {
setPermission(name, value);
return this;
}
/**
* Sets the permission type.
*
* @param type the given permission type to set.
*/
public void setType(PermissionType type) {
this.type = type;
}
/**
* Sets the permission type.
*
* @param type the given permission type to set.
* @return the current {@link Permissions} object.
*/
public Permissions withType(PermissionType type) {
setType(type);
return this;
}
/**
* @return the permission type.
*/
public PermissionType getType() {
return type;
}
@Override
public Permissions clone() {
Permissions that = new Permissions();
that.withType(type).setPropertiesFrom(this);
return that;
}
public String getTypeName(){
return type.typeName();
}
@Override
public String elementName() {
return type.typeName();
}
//~ helper enum =============================================================
/**
* PermissionType
*
* @author Dzung Nguyen
* @version $Id PermissionType 2014-10-30 16:29:30z dungvnguyen $
* @since 1.0
*/
public enum PermissionType {
//~ enum values =============================================================
DEFECT("defect", 3),
MODULE("module", 9),
REQUIREMENT("requirement", 2),
TEST_CASE("test_case", 1),
TEST_RUN("test_run", 14),
RELEASE("release", 8),
BUILD("build", 7),
TEST_CYCLE("test_cycle", 17),
TEST_SUITE("test_suite", 12),
PROJECT("project", 61),
PROJECT_SETTING("project_setting", 62),
QMAP("qmap", 63),
SESSION("session", 64),
UNKNOWN("unknown", 0);
//~ class properties ========================================================
private String typeName;
private int typeValue;
//~ class members ===========================================================
/**
* Create the permission type.
*
* @param typeName the given object type name.
* @param typeValue the given object type value.
*/
PermissionType(String typeName, int typeValue) {
this.typeName = typeName;
this.typeValue = typeValue;
}
/**
* @param typeName typeName
* @return the {@link PermissionType} corresponding with the given type name.
*/
public static PermissionType resolve(String typeName) {
String lcType = (typeName == null ? "" : typeName.toLowerCase());
for (PermissionType value : values()) {
if (value.typeName.equals(lcType)) return value;
}
return UNKNOWN;
}
/**
* @param typeValue typeValue
* @return the {@link PermissionType} corresponding with the given object type value.
*/
public static PermissionType resolve(int typeValue) {
for (PermissionType value : values()) {
if (value.typeValue == typeValue) return value;
}
return UNKNOWN;
}
/**
* @return the type name.
*/
public String typeName() {
return typeName;
}
/**
* @return the type value.
*/
public int typeValue() {
return typeValue;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy