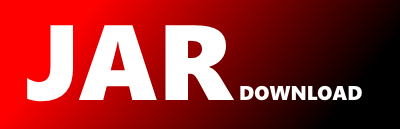
org.qas.qtest.api.services.project.model.Project Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.project.model;
import com.fasterxml.jackson.annotation.JsonIgnore;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.databind.annotation.JsonDeserialize;
import com.fasterxml.jackson.databind.annotation.JsonSerialize;
import org.qas.api.DateTimeDeserializer;
import org.qas.api.DateTimeSerializer;
import org.qas.api.JsonMapper;
import org.qas.qtest.api.internal.model.QTestBaseModel;
import java.util.Collections;
import java.util.Date;
import java.util.LinkedList;
import java.util.List;
/**
* Project
*
* @author Dzung Nguyen
* @version $Id Project 2014-03-27 16:22:30z dungvnguyen $
* @since 1.0
*/
public final class Project extends QTestBaseModel {
@JsonProperty("id")
private Long id;
@JsonProperty("name")
private String name;
@JsonProperty("description")
private String description;
@JsonProperty("sample")
private Boolean sample;
@JsonProperty("start_date")
private Date startDate;
@JsonProperty("end_date")
private Date endDate;
@JsonProperty("defect_tracking_systems")
private List defectTrackingSystems;
public Project() {
setSample(Boolean.FALSE);
}
/**
* @return the project identifier.
*/
public Long getId() {
return id;
}
/**
* Sets the project identifier.
*
* @param id the given project identifier.
* @return the current module.
*/
public Project setId(Long id) {
this.id = id;
return this;
}
/**
* Sets the project identifier and return itself.
*
* @param id the project identifier.
* @return the current {@link Project} object.
*/
public Project withId(Long id) {
setId(id);
return this;
}
/**
* @return the project name.
*/
public String getName() {
return name;
}
/**
* Sets project name.
*
* @param name the given project name to set.
* @return the current module.
*/
public Project setName(String name) {
this.name = name;
return this;
}
/**
* Sets the project name and return itself.
*
* @param name the given name of project to set.
* @return current {@link Project} object.
*/
public Project withName(String name) {
setName(name);
return this;
}
/**
* @return the project description.
*/
public String getDescription() {
return description;
}
/**
* Sets the project description.
*
* @param description the given project description.
* @return the current module.
*/
public Project setDescription(String description) {
this.description = description;
return this;
}
/**
* Sets project description and return itself.
*
* @param description the given description to set.
* @return the current {@link Project} object.
*/
public Project withDescription(String description) {
setDescription(description);
return this;
}
/**
* @return {@code true} if the project is sample project, otherwise {@code false}
*/
public Boolean isSample() {
return sample;
}
/**
* Sets the flag that marks the project is sample project.
*
* @param sample the given sample project flag to set.
* @return the current module.
*/
public Project setSample(Boolean sample) {
this.sample = (sample == null ? Boolean.FALSE : sample);
return this;
}
/**
* Sets the flag that marks the project is sample project and return itself.
*
* @param sample the given sample project flag to set.
* @return the current {@link Project} object.
*/
public Project withSample(Boolean sample) {
setSample(sample);
return this;
}
/**
* @return the start date of project.
*/
@JsonSerialize(using = DateTimeSerializer.class, typing = JsonSerialize.Typing.STATIC, include = JsonSerialize.Inclusion.NON_NULL)
public Date getStartDate() {
return startDate;
}
/**
* Sets the start date of project.
*
* @param startDate the given project's start date to set.
* @return the current module.
*/
@JsonDeserialize(using = DateTimeDeserializer.class)
public Project setStartDate(Date startDate) {
this.startDate = startDate;
return this;
}
/**
* Sets the start date of project.
*
* @param startDate the given project's start date to set.
* @return current {@link Project} object.
*/
public Project withStartDate(Date startDate) {
setStartDate(startDate);
return this;
}
/**
* @return the end date of project.
*/
@JsonSerialize(using = DateTimeSerializer.class, typing = JsonSerialize.Typing.STATIC, include = JsonSerialize.Inclusion.NON_NULL)
public Date getEndDate() {
return endDate;
}
/**
* Sets the end date of project.
*
* @param endDate the given project's end date to set.
* @return the current module.
*/
@JsonDeserialize(using = DateTimeDeserializer.class)
public Project setEndDate(Date endDate) {
this.endDate = endDate;
return this;
}
/**
* Sets the end date of project.
*
* @param startDate the given project's end date to set.
* @return current {@link Project} object.
*/
public Project withEndDate(Date startDate) {
setEndDate(startDate);
return this;
}
/**
* @return the list of project's defect tracking system.
*/
public List getDefectTrackingSystems() {
if (defectTrackingSystems == null) {
return Collections.emptyList();
}
return defectTrackingSystems;
}
/**
* Sets the list of project's defect tracking system.
*
* @param dtses the given list of defect tracking systems.
* @return current instance.
*/
public Project setDefectTrackingSystems(List dtses) {
this.defectTrackingSystems = dtses;
return this;
}
/**
* Sets the list of project's defect tracking system and return itself.
*
* @param dtses the given list of defect tracking systems.
* @return current instance.
*/
public Project withDefectTrackingSystems(List dtses) {
setDefectTrackingSystems(dtses);
return this;
}
/**
* Adds defect tracking system to project.
*
* @param dts the given defect tracking system object.
* @return the current {@link Project} object.
*/
public Project addDefectTrackingSystem(DefectTrackingSystem dts) {
if (defectTrackingSystems == null) {
defectTrackingSystems = new LinkedList<>();
}
defectTrackingSystems.add(dts);
return this;
}
@Override
@JsonIgnore
public Project clone() {
Project that = new Project();
that.setPropertiesFrom(this);
return that;
}
@Override
@JsonIgnore
public String elementName() {
return "project";
}
@Override
public String toString() {
return JsonMapper.toJson(this);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy