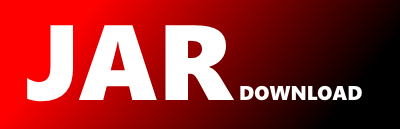
org.qas.qtest.api.services.project.model.UserPermissions Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.project.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import org.qas.qtest.api.internal.model.QTestBaseModel;
import java.util.Map;
/**
* UserPermissions
*
* @author Dzung Nguyen
* @version $Id UserPermissions 2014-11-05 14:20:30z dungvnguyen $
* @since 1.0
*/
public final class UserPermissions extends QTestBaseModel {
@JsonProperty("user_id")
private Long userId;
@JsonProperty("project_id")
private Long projectId;
/**
* Creates the permission instance.
*/
public UserPermissions() {
}
/**
* Sets the given user identifier.
*
* @param userId the given user identifier to set.
* @return the current module.
*/
public UserPermissions setUserId(Long userId) {
this.userId = userId;
return this;
}
/**
* Sets the given user identifier.
*
* @param userId the given user identifier to set.
* @return current {@link UserPermissions} instance.
*/
public UserPermissions withUserId(Long userId) {
setUserId(userId);
return this;
}
/**
* @return the user identifier.
*/
public Long getUserId() {
return userId;
}
/**
* Sets the user project.
*
* @param projectId the given project identifier.
* @return the current module.
*/
public UserPermissions setProjectId(Long projectId) {
this.projectId = projectId;
return this;
}
/**
* Sets the given project identifier.
*
* @param projectId the given project identifier to set.
* @return the current {@link UserPermissions} instance.
*/
public UserPermissions withProjectId(Long projectId) {
setProjectId(projectId);
return this;
}
/**
* @return the current project id.
*/
public Long getProjectId() {
return projectId;
}
/**
* Sets the user permissions.
*
* @param permissions the given user permissions to set.
* @return the current module.
*/
public UserPermissions setPermissions(Permissions permissions) {
if (permissions != null) {
setProperty(permissions.getTypeName(), permissions.getPropertyMap());
}
return this;
}
/**
* Sets the user permissions.
*
* @param permissions the given user permissions to set.
* @return the current {@link UserPermissions} object.
*/
public UserPermissions withPermissions(Permissions permissions) {
setPermissions(permissions);
return this;
}
/**
* @param name name
* @return the permissions from it's name.
*/
public Permissions getPermissions(String name) {
if (hasProperty(name) && getProperty(name) instanceof Map) {
Map map = (Map) getProperty(name);
Permissions permissions = new Permissions(map);
permissions.setType(Permissions.PermissionType.resolve(name));
return permissions;
}
return null;
}
/**
* Returns {@code true} if the user has the given permission.
*
* @param permission the given permission to check.
* @return {@code true} if the user has the given permission, otherwise {@code false}
*/
public boolean hasPermission(String permission) {
if (permission.indexOf('_') >= 0) {
String type = permission.substring(permission.lastIndexOf('_') + 1);
if (type != null && hasProperty(type)) {
Permissions permissions = getPermissions(type);
if (permissions != null) {
return permissions.hasPermission(permission.substring(0, permission.lastIndexOf('_')));
}
}
}
return false;
}
@Override
public UserPermissions clone() {
UserPermissions that = new UserPermissions();
that.setPropertiesFrom(this);
return that;
}
@Override
public String jsonElementName() {
return "user_permissions";
}
@Override
public String elementName() {
return "user-permissions";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy