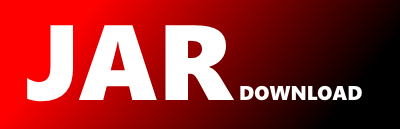
org.qas.qtest.api.services.requirement.model.Requirement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.requirement.model;
import com.fasterxml.jackson.annotation.JsonProperty;
import org.qas.qtest.api.internal.model.FieldValue;
import org.qas.qtest.api.internal.model.QTestBaseModel;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
/**
* Requirement
*
* @author Dzung Nguyen
* @version $Id Requirement 2014-05-19 00:34:30z dungvnguyen $
* @since 1.0
*/
public class Requirement extends QTestBaseModel {
@JsonProperty("id")
private Long id;
@JsonProperty("name")
private String name;
@JsonProperty("order")
private Integer order;
@JsonProperty("pid")
private String pid;
@JsonProperty("properties")
private List fieldValues;
public Requirement() {
}
/**
* @return the requirement identifier.
*/
public Long getId() {
return id;
}
/**
* Sets the requirement identifier.
*
* @param id the given requirement identifier.
* @return current instance.
*/
public Requirement setId(Long id) {
this.id = id;
return this;
}
/**
* Sets the requirement identifier and return itself.
*
* @param id the given requirement identifier value to set.
* @return the requirement instance.
*/
public Requirement withId(Long id) {
setId(id);
return this;
}
/**
* @return the requirement name.
*/
public String getName() {
return name;
}
/**
* Sets the requirement name.
*
* @param name the given requirement name to set.
* @return current instance.
*/
public Requirement setName(String name) {
this.name = name;
return this;
}
/**
* Sets the requirement name and return itselt.
*
* @param name the given requirement name to set.
* @return the requirement instance.
*/
public Requirement withName(String name) {
setName(name);
return this;
}
/**
* @return the requirement order.
*/
public Integer getOrder() {
return order;
}
/**
* Sets the requirement order.
*
* @param order the given requirement order value to set.
* @return current instance.
*/
public Requirement setOrder(Integer order) {
this.order = order;
return this;
}
/**
* Sets the requirement order and return itself.
*
* @param order the given requirement order value to set.
* @return the given requirement instance.
*/
public Requirement withOrder(Integer order) {
setOrder(order);
return this;
}
/**
* @return the requirement pid value.
*/
public String getPid() {
return pid;
}
/**
* Sets the requirement pid value.
*
* @param pid the given requirement pid value to set.
* @return current instance.
*/
public Requirement setPid(String pid) {
this.pid = pid;
return this;
}
/**
* Sets the requirement pid value.
*
* @param pid the given requirement pid value to set.
* @return the requirement instance.
*/
public Requirement withPid(String pid) {
setPid(pid);
return this;
}
/**
* @return the list of field value objects.
*/
public List getFieldValues() {
if (null == fieldValues) {
return Collections.emptyList();
}
return fieldValues;
}
/**
* Sets the list of field value objects.
*
* @param values the given field value objects to set.
* @return current instance.
*/
public Requirement setFieldValues(List values) {
this.fieldValues = new ArrayList<>();
if (null == values) {
return this;
}
for (FieldValue fieldValue : values) {
addFieldValue(fieldValue);
}
return this;
}
/**
* Sets the list of field value objects.
*
* @param values the given field value objects to set.
* @return the requirement instance.
*/
public Requirement withFieldValues(List values) {
setFieldValues(values);
return this;
}
/**
* Adds the property value to requirement and return itself.
*
* @param value the given field value to add.
* @return the requirement instance.
*/
public Requirement addFieldValue(FieldValue value) {
if (value == null || value.getValue() == null) {
return this;
}
if (fieldValues == null) {
fieldValues = new ArrayList<>();
}
fieldValues.add(value);
return this;
}
@Override
public Requirement clone() {
Requirement that = new Requirement();
that.setPropertiesFrom(this);
return that;
}
@Override
public String elementName() {
return "requirement";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy