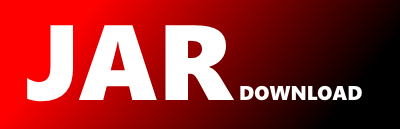
org.qas.qtest.api.services.user.UserServiceAsync Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.user;
import org.qas.api.AuthServiceException;
import org.qas.api.handler.AsyncHandler;
import org.qas.qtest.api.services.user.model.AssignToProjectRequest;
import org.qas.qtest.api.services.user.model.AssignToProjectResult;
import org.qas.qtest.api.services.user.model.CreateUserRequest;
import org.qas.qtest.api.services.user.model.User;
import java.util.concurrent.Future;
/**
* UserServiceAsync
*
* @author Dzung Nguyen
* @version $Id UserServiceAsync 2014-07-10 22:00:30z dungvnguyen $
* @since 1.0
*/
public interface UserServiceAsync extends UserService {
/**
* Creates user information from the given create user request.
*
* @param createUserRequest the given user request to perform create action.
* @return the user information.
* @throws AuthServiceException if an error occurs during create user information.
*/
Future createAsync(CreateUserRequest createUserRequest) throws AuthServiceException;
/**
* Creates user information from the given create user request.
*
* @param createUserRequest the given user request to perform create action.
* @param asyncHandler callback handler for events in the life-cycle of the request.
* Users could provide the implementation of the for callback
* methods in this interface to process the operation result or
* handle the exception.
* @return the user information.
* @throws AuthServiceException if an error occurs during create user information.
*/
Future createAsync(CreateUserRequest createUserRequest,
AsyncHandler asyncHandler) throws AuthServiceException;
/**
* Assign user to project from the request.
*
* @param assignToProjectRequest the given assign to project request.
* @return the assign to project result.
* @throws AuthServiceException if an error occurs during assigning user to project.
*/
Future assignToProjectAsync(AssignToProjectRequest assignToProjectRequest)
throws AuthServiceException;
/**
* Assign user to project from the request.
*
* @param assignToProjectRequest the given assign to project request.
* @param asyncHandler callback handler for events in the life-cycle of the request.
* Users could provide the implementation of the for callback
* methods in this interface to process the operation result or
* handle the exception.
* @return the assign to project result.
* @throws AuthServiceException if an error occurs during assigning user to project.
*/
Future assignToProjectAsync(AssignToProjectRequest assignToProjectRequest,
AsyncHandler asyncHandler)
throws AuthServiceException;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy