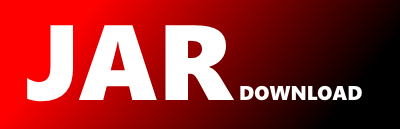
org.qas.qtest.api.services.user.UserServiceAsyncClient Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qtest-sdk-java Show documentation
Show all versions of qtest-sdk-java Show documentation
A java SDK client wrap qTest REST API
The newest version!
package org.qas.qtest.api.services.user;
import org.qas.api.AuthServiceException;
import org.qas.api.ClientConfiguration;
import org.qas.api.handler.AsyncHandler;
import org.qas.qtest.api.auth.DefaultQTestCredentialsProviderChain;
import org.qas.qtest.api.auth.QTestCredentials;
import org.qas.qtest.api.auth.QTestCredentialsProvider;
import org.qas.qtest.api.auth.StaticQTestCredentialsProvider;
import org.qas.qtest.api.services.user.model.AssignToProjectRequest;
import org.qas.qtest.api.services.user.model.AssignToProjectResult;
import org.qas.qtest.api.services.user.model.CreateUserRequest;
import org.qas.qtest.api.services.user.model.User;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.Future;
/**
* UserServiceAsyncClient
*
* @author Dzung Nguyen
* @version $Id UserServiceAsyncClient 2014-07-10 22:05:30z dungvnguyen $
* @since 1.0
*/
public class UserServiceAsyncClient extends UserServiceClient implements UserServiceAsync {
/**
* Constructs a new client to invoke service method on UserService using
* the default qTest credentials provider and default client configuration options.
*/
public UserServiceAsyncClient() {
this(new DefaultQTestCredentialsProviderChain(), new ClientConfiguration(), Executors.newCachedThreadPool());
}
/**
* Constructs a new client to invoke service method on UserService using
* the default qTest credentials provider and default client configuration options.
*
* @param executorService the executor service for executing asynchronous request.
*/
public UserServiceAsyncClient(ExecutorService executorService) {
this(new DefaultQTestCredentialsProviderChain(), new ClientConfiguration(), executorService);
}
/**
* Constructs a new client to invoke service method on UserService using
* the default qTest credentials provider and client configuration options.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to UserService
*/
public UserServiceAsyncClient(ClientConfiguration clientConfiguration) {
this(new DefaultQTestCredentialsProviderChain(), clientConfiguration, Executors.newCachedThreadPool());
}
/**
* Constructs a new client to invoke service method on UserService using
* the default qTest credentials provider and client configuration options.
*
* @param clientConfiguration The client configuration options controlling how this
* client connects to UserService.
* @param executorService the executor service for executing asynchronous request.
*/
public UserServiceAsyncClient(ClientConfiguration clientConfiguration, ExecutorService executorService) {
this(new DefaultQTestCredentialsProviderChain(), clientConfiguration, executorService);
}
/**
* Constructs a new client to invoke service method on UserService using
* the specified qTest credentials.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
*/
public UserServiceAsyncClient(QTestCredentials credentials) {
this(credentials, new ClientConfiguration(), Executors.newCachedThreadPool());
}
/**
* Constructs a new client to invoke service method on UserService using
* the specified qTest credentials.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
* @param executorService the executor service for executing asynchronous request.
*/
public UserServiceAsyncClient(QTestCredentials credentials, ExecutorService executorService) {
this(credentials, new ClientConfiguration(), executorService);
}
/**
* Constructs a new client to invoke service method on UserService using
* the specified qTest credentials and client configuration options.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to UserService
*/
public UserServiceAsyncClient(QTestCredentials credentials, ClientConfiguration clientConfiguration) {
this(credentials, clientConfiguration, Executors.newCachedThreadPool());
}
/**
* Constructs a new client to invoke service method on UserService using
* the specified qTest credentials and client configuration options.
*
* @param credentials The qTest credentials which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to UserService
* @param executorService the executor service for executing asynchronous request.
*/
public UserServiceAsyncClient(QTestCredentials credentials, ClientConfiguration clientConfiguration,
ExecutorService executorService) {
this(new StaticQTestCredentialsProvider(credentials), clientConfiguration, executorService);
}
/**
* Constructs a new client to invoke service method on UserService using
* the specified qTest credentials provider and client configuration options.
*
* @param credentialsProvider The qTest credentials provider which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to UserService
*/
public UserServiceAsyncClient(QTestCredentialsProvider credentialsProvider,
ClientConfiguration clientConfiguration) {
this(credentialsProvider, clientConfiguration, Executors.newCachedThreadPool());
}
/**
* Constructs a new client to invoke service method on UserService using
* the specified qTest credentials provider and client configuration options.
*
* @param credentialsProvider The qTest credentials provider which will provide
* credentials to authenticate request with qTest services.
* @param clientConfiguration The client configuration options controlling how this
* client connects to UserService
* @param executorService the executor service for executing asynchronous request.
*/
public UserServiceAsyncClient(QTestCredentialsProvider credentialsProvider,
ClientConfiguration clientConfiguration, ExecutorService executorService) {
super(credentialsProvider, clientConfiguration);
this.executorService = executorService;
}
//~ implements methods ======================================================
@Override
public Future createAsync(final CreateUserRequest createUserRequest) throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public User call() throws Exception {
return create(createUserRequest);
}
});
}
@Override
public Future createAsync(final CreateUserRequest createUserRequest,
final AsyncHandler asyncHandler) throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public User call() throws Exception {
final User result;
try {
result = create(createUserRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(createUserRequest, result);
return result;
}
});
}
@Override
public Future assignToProjectAsync(final AssignToProjectRequest assignToProjectRequest) throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public AssignToProjectResult call() throws Exception {
return assignToProject(assignToProjectRequest);
}
});
}
@Override
public Future assignToProjectAsync(final AssignToProjectRequest assignToProjectRequest,
final AsyncHandler asyncHandler) throws AuthServiceException {
return executorService.submit(new Callable() {
@Override
public AssignToProjectResult call() throws Exception {
final AssignToProjectResult result;
try {
result = assignToProject(assignToProjectRequest);
} catch (Exception ex) {
asyncHandler.onError(ex);
throw ex;
}
asyncHandler.onSuccess(assignToProjectRequest, result);
return result;
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy