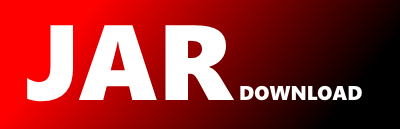
org.apache.flink.kubernetes.utils.KubernetesInitializerUtils Maven / Gradle / Ivy
The newest version!
/*
* Licensed to the Apache Software Foundation (ASF) under one
* or more contributor license agreements. See the NOTICE file
* distributed with this work for additional information
* regarding copyright ownership. The ASF licenses this file
* to you under the Apache License, Version 2.0 (the
* "License"); you may not use this file except in compliance
* with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.apache.flink.kubernetes.utils;
import org.apache.flink.configuration.Configuration;
import org.apache.flink.core.plugin.PluginUtils;
import org.apache.flink.kubernetes.configuration.KubernetesConfigOptionsInternal;
import org.apache.flink.kubernetes.kubeclient.parameters.KubernetesJobManagerParameters;
import org.apache.flink.runtime.jobgraph.JobGraph;
import org.apache.hadoop.fs.FileSystem;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.hdfs.HdfsConfiguration;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.ObjectOutputStream;
import java.net.URL;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import static org.apache.flink.runtime.entrypoint.component.FileJobGraphRetriever.JOB_GRAPH_FILE_PATH;
/**
* Utilities for the init Container.
*/
public class KubernetesInitializerUtils {
private static final Logger LOG = LoggerFactory.getLogger(KubernetesInitializerUtils.class);
public static void uploadLocalDependencies(
Configuration flinkConfig,
JobGraph jobGraph,
KubernetesJobManagerParameters kubernetesJobManagerParameters,
String clusterId) throws IOException {
final String jarsDownloadDir = kubernetesJobManagerParameters.getJarsDownloadDirInPod();
final String filesDownloadDir = kubernetesJobManagerParameters.getFilesDownloadDirInPod();
final Optional localHadoopConfigirationDirectoryOption =
kubernetesJobManagerParameters.getLocalHadoopConfigurationDirectory();
if (!localHadoopConfigirationDirectoryOption.isPresent()) {
throw new RuntimeException("Failed to find hadoop configuration directory.");
}
// ------------------ Initialize the file systems -------------------------
org.apache.flink.core.fs.FileSystem.initialize(
flinkConfig,
PluginUtils.createPluginManagerFromRootFolder(flinkConfig));
// initialize file system
// Copy the application master jar to the filesystem
// Create a local resource to point to the destination jar path
final HdfsConfiguration hdfsConfiguration = new HdfsConfiguration();
final FileSystem fs = FileSystem.get(hdfsConfiguration);
final Path homeDir = fs.getHomeDirectory();
final List userJarDst = new ArrayList<>();
final List classPaths = new ArrayList<>(jobGraph.getClasspaths());
final long currentTimeMillis = System.currentTimeMillis();
for (org.apache.flink.core.fs.Path path: jobGraph.getUserJars()) {
final String suffix = ".flink/" + clusterId + "/" + currentTimeMillis + "/" + path.getName();
final Path dst = new Path(homeDir, suffix);
final Path localSrcPath = new Path(path.getPath());
LOG.debug("Copying from {} to {} ", localSrcPath, dst);
fs.copyFromLocalFile(false, true, localSrcPath, dst);
userJarDst.add(dst.toUri().getRawPath());
classPaths.add(new URL("file://" + jarsDownloadDir + "/" + path.getName()));
}
flinkConfig.set(KubernetesConfigOptionsInternal.REMOTE_JAR_DEPENDENCIES, userJarDst);
jobGraph.setClasspaths(classPaths);
// write job graph to tmp file and upload it to remote DFS
File tmpJobGraphFile = null;
try {
tmpJobGraphFile = File.createTempFile(clusterId, null);
try (FileOutputStream output = new FileOutputStream(tmpJobGraphFile);
ObjectOutputStream obOutput = new ObjectOutputStream(output)) {
obOutput.writeObject(jobGraph);
}
final String jobGraphFilename = "job.graph";
final String suffix = ".flink/" + clusterId + "/" + currentTimeMillis + "/" + jobGraphFilename;
final Path dst = new Path(homeDir, suffix);
final Path localSrcPath = new Path(tmpJobGraphFile.toURI());
LOG.debug("Copying from {} to {} ", localSrcPath, dst);
fs.copyFromLocalFile(false, true, localSrcPath, dst);
flinkConfig.setString(JOB_GRAPH_FILE_PATH, filesDownloadDir + "/" + jobGraphFilename);
flinkConfig.set(KubernetesConfigOptionsInternal.REMOTE_FILE_DEPENDENCIES, Collections.singletonList(dst.toUri().getRawPath()));
} catch (Exception e) {
LOG.warn("Failed to upload job graph file");
throw e;
} finally {
if (tmpJobGraphFile != null && !tmpJobGraphFile.delete()) {
LOG.warn("Fail to delete temporary JobGraph file {}.", tmpJobGraphFile.toPath());
}
}
}
private KubernetesInitializerUtils() {}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy