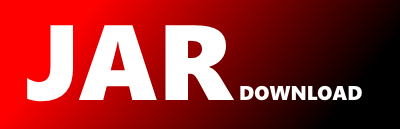
com.qcloud.cos.internal.Unmarshallers Maven / Gradle / Ivy
package com.qcloud.cos.internal;
import java.io.InputStream;
import com.qcloud.cos.internal.XmlResponsesSaxParser.CompleteMultipartUploadHandler;
import com.qcloud.cos.internal.XmlResponsesSaxParser.CopyObjectResultHandler;
import com.qcloud.cos.model.InitiateMultipartUploadResult;
import com.qcloud.cos.model.MultipartUploadListing;
import com.qcloud.cos.model.ObjectListing;
import com.qcloud.cos.model.PartListing;
/*** Collection of unmarshallers for COS XML responses. */
public class Unmarshallers {
// /**
// * Unmarshaller for the ListBuckets XML response.
// */
// public static final class ListBucketsUnmarshaller
// implements Unmarshaller, InputStream> {
// public List unmarshall(InputStream in) throws Exception {
// return new XmlResponsesSaxParser().parseListMyBucketsResponse(in).getBuckets();
// }
// }
//
// /**
// * Unmarshaller for the ListBuckets XML response, parsing out the owner even if the list is
// * empty.
// */
// public static final class ListBucketsOwnerUnmarshaller
// implements Unmarshaller {
// public Owner unmarshall(InputStream in) throws Exception {
// return new XmlResponsesSaxParser().parseListMyBucketsResponse(in).getOwner();
// }
// }
/**
* Unmarshaller for the ListObjects XML response.
*/
public static final class ListObjectsUnmarshaller
implements Unmarshaller {
public ObjectListing unmarshall(InputStream in) throws Exception {
return new XmlResponsesSaxParser().parseListBucketObjectsResponse(in)
.getObjectListing();
}
}
//
// /**
// * Unmarshaller for the ListVersions XML response.
// */
// public static final class VersionListUnmarshaller
// implements Unmarshaller {
// public VersionListing unmarshall(InputStream in) throws Exception {
// return new XmlResponsesSaxParser().parseListVersionsResponse(in).getListing();
// }
// }
//
// /**
// * Unmarshaller for the AccessControlList XML response.
// */
// public static final class AccessControlListUnmarshaller
// implements Unmarshaller {
// public AccessControlList unmarshall(InputStream in) throws Exception {
// return new XmlResponsesSaxParser().parseAccessControlListResponse(in)
// .getAccessControlList();
// }
// }
//
// /**
// * Unmarshaller for the BucketLoggingStatus XML response.
// */
// public static final class BucketLoggingConfigurationnmarshaller
// implements Unmarshaller {
// public BucketLoggingConfiguration unmarshall(InputStream in) throws Exception {
// return new XmlResponsesSaxParser().parseLoggingStatusResponse(in)
// .getBucketLoggingConfiguration();
// }
// }
//
// /**
// * Unmarshaller for the BucketLocation XML response.
// */
// public static final class BucketLocationUnmarshaller
// implements Unmarshaller {
// public String unmarshall(InputStream in) throws Exception {
// String location = new XmlResponsesSaxParser().parseBucketLocationResponse(in);
//
// /*
// * COS treats the US location differently, and assumes that if the reported location is
// * null, then it's a US bucket.
// */
// if (location == null)
// location = "US";
//
// return location;
// }
// }
//
// /**
// * Unmarshaller for the BucketVersionConfiguration XML response.
// */
// public static final class BucketVersioningConfigurationUnmarshaller
// implements Unmarshaller {
// public BucketVersioningConfiguration unmarshall(InputStream in) throws Exception {
// return new XmlResponsesSaxParser().parseVersioningConfigurationResponse(in)
// .getConfiguration();
// }
// }
//
// /**
// * Unmarshaller for the BucketWebsiteConfiguration XML response.
// */
// public static final class BucketWebsiteConfigurationUnmarshaller
// implements Unmarshaller {
// public BucketWebsiteConfiguration unmarshall(InputStream in) throws Exception {
// return new XmlResponsesSaxParser().parseWebsiteConfigurationResponse(in)
// .getConfiguration();
// }
// }
//
// /**
// * Unmarshaller for the BucketNotificationConfiguration XML response.
// */
// public static final class BucketNotificationConfigurationUnmarshaller
// implements Unmarshaller {
// public BucketNotificationConfiguration unmarshall(InputStream in) throws Exception {
// return new XmlResponsesSaxParser().parseNotificationConfigurationResponse(in)
// .getConfiguration();
// }
// }
//
// /**
// * Unmarshaller for the BucketTaggingConfiguration XML response.
// */
// public static final class BucketTaggingConfigurationUnmarshaller
// implements Unmarshaller {
// public BucketTaggingConfiguration unmarshall(InputStream in) throws Exception {
// return new XmlResponsesSaxParser().parseTaggingConfigurationResponse(in)
// .getConfiguration();
// }
// }
/**
* Unmarshaller for the a direct InputStream response.
*/
public static final class InputStreamUnmarshaller
implements Unmarshaller {
public InputStream unmarshall(InputStream in) throws Exception {
return in;
}
}
/**
* Unmarshaller for the CopyObject XML response.
*/
public static final class CopyObjectUnmarshaller
implements Unmarshaller {
public CopyObjectResultHandler unmarshall(InputStream in) throws Exception {
return new XmlResponsesSaxParser().parseCopyObjectResponse(in);
}
}
public static final class CompleteMultipartUploadResultUnmarshaller
implements Unmarshaller {
public CompleteMultipartUploadHandler unmarshall(InputStream in) throws Exception {
return new XmlResponsesSaxParser().parseCompleteMultipartUploadResponse(in);
}
}
public static final class InitiateMultipartUploadResultUnmarshaller
implements Unmarshaller {
public InitiateMultipartUploadResult unmarshall(InputStream in) throws Exception {
return new XmlResponsesSaxParser().parseInitiateMultipartUploadResponse(in)
.getInitiateMultipartUploadResult();
}
}
public static final class ListMultipartUploadsResultUnmarshaller
implements Unmarshaller {
public MultipartUploadListing unmarshall(InputStream in) throws Exception {
return new XmlResponsesSaxParser().parseListMultipartUploadsResponse(in)
.getListMultipartUploadsResult();
}
}
public static final class ListPartsResultUnmarshaller
implements Unmarshaller {
public PartListing unmarshall(InputStream in) throws Exception {
return new XmlResponsesSaxParser().parseListPartsResponse(in).getListPartsResult();
}
}
// public static final class DeleteObjectsResultUnmarshaller
// implements Unmarshaller {
//
// public DeleteObjectsResponse unmarshall(InputStream in) throws Exception {
// return new XmlResponsesSaxParser().parseDeletedObjectsResult(in)
// .getDeleteObjectResult();
// }
// }
//
// public static final class BucketLifecycleConfigurationUnmarshaller
// implements Unmarshaller {
//
// public BucketLifecycleConfiguration unmarshall(InputStream in) throws Exception {
// return new XmlResponsesSaxParser().parseBucketLifecycleConfigurationResponse(in)
// .getConfiguration();
// }
// }
//
// public static final class BucketCrossOriginConfigurationUnmarshaller
// implements Unmarshaller {
// public BucketCrossOriginConfiguration unmarshall(InputStream in) throws Exception {
// return new XmlResponsesSaxParser().parseBucketCrossOriginConfigurationResponse(in)
// .getConfiguration();
// }
// }
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy