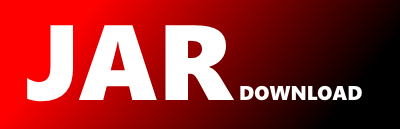
com.qdesrame.openapi.diff.compare.MapKeyDiff Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openapi-diff Show documentation
Show all versions of openapi-diff Show documentation
Utility for comparing two OpenAPI specifications.
package com.qdesrame.openapi.diff.compare;
import java.util.*;
import java.util.Map.Entry;
/**
* compare two Maps by key
*
* @author QDesrame
*/
public class MapKeyDiff {
private Map increased;
private Map missing;
private List sharedKey;
private MapKeyDiff() {
this.sharedKey = new ArrayList<>();
this.increased = new HashMap<>();
this.missing = new HashMap<>();
}
public static MapKeyDiff diff(Map mapLeft,
Map mapRight) {
MapKeyDiff instance = new MapKeyDiff<>();
if (null == mapLeft && null == mapRight) return instance;
if (null == mapLeft) {
instance.increased = mapRight;
return instance;
}
if (null == mapRight) {
instance.missing = mapLeft;
return instance;
}
instance.increased = new LinkedHashMap<>(mapRight);
instance.missing = new LinkedHashMap<>();
for (Entry entry : mapLeft.entrySet()) {
K leftKey = entry.getKey();
V leftValue = entry.getValue();
if (mapRight.containsKey(leftKey)) {
instance.increased.remove(leftKey);
instance.sharedKey.add(leftKey);
} else {
instance.missing.put(leftKey, leftValue);
}
}
return instance;
}
public Map getIncreased() {
return increased;
}
public Map getMissing() {
return missing;
}
public List getSharedKey() {
return sharedKey;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy