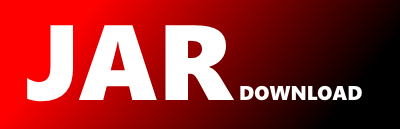
com.qdesrame.openapi.diff.compare.PathsDiff Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of openapi-diff Show documentation
Show all versions of openapi-diff Show documentation
Utility for comparing two OpenAPI specifications.
The newest version!
package com.qdesrame.openapi.diff.compare;
import com.qdesrame.openapi.diff.model.ChangedPaths;
import io.swagger.v3.oas.models.PathItem;
import io.swagger.v3.oas.models.Paths;
import java.util.*;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class PathsDiff {
private static final String REGEX_PATH = "\\{([^/]+)\\}";
private OpenApiDiff openApiDiff;
public PathsDiff(OpenApiDiff openApiDiff) {
this.openApiDiff = openApiDiff;
}
private static String normalizePath(String path) {
return path.replaceAll(REGEX_PATH, "{}");
}
private static List extractParameters(String path) {
ArrayList params = new ArrayList<>();
Pattern pattern = Pattern.compile(REGEX_PATH);
Matcher matcher = pattern.matcher(path);
while (matcher.find()) {
params.add(matcher.group(1));
}
return params;
}
public Optional diff(final Map left, final Map right) {
ChangedPaths changedPaths = new ChangedPaths(left, right);
changedPaths.getIncreased().putAll(right);
left.keySet().forEach((String url) -> {
PathItem leftPath = left.get(url);
String template = normalizePath(url);
Optional result = right.keySet().stream().filter(s -> normalizePath(s).equals(template)).findFirst();
if (result.isPresent()) {
if (!changedPaths.getIncreased().containsKey(result.get())) {
throw new IllegalArgumentException("Two path items have the same signature: " + template);
}
PathItem rightPath = changedPaths.getIncreased().remove(result.get());
Map params = new HashMap<>();
if (!url.equals(result.get())) {
List oldParams = extractParameters(url);
List newParams = extractParameters(result.get());
for (int i = 0; i < oldParams.size(); i++) {
params.put(oldParams.get(i), newParams.get(i));
}
}
openApiDiff.getPathDiff().diff(url, params, leftPath, rightPath).ifPresent(path -> changedPaths.getChanged().put(result.get(), path));
} else {
changedPaths.getMissing().put(url, leftPath);
}
});
return changedPaths.isDiff() ? Optional.of(changedPaths) : Optional.empty();
}
public static Paths valOrEmpty(Paths path) {
if (path == null) {
path = new Paths();
}
return path;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy