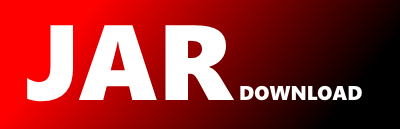
com.qingcloud.sdk.service.JobService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qingcloud-sdk-java Show documentation
Show all versions of qingcloud-sdk-java Show documentation
青云为开发者提供软件开发包 SDK (Software Development Kit), 可以利用它对青云的资源进行查看、创建和操作,借此开发出更富创造力的产品。
// +-------------------------------------------------------------------------
// | Copyright (C) 2016 Yunify, Inc.
// +-------------------------------------------------------------------------
// | Licensed under the Apache License, Version 2.0 (the "License");
// | you may not use this work except in compliance with the License.
// | You may obtain a copy of the License in the LICENSE file, or at:
// |
// | http://www.apache.org/licenses/LICENSE-2.0
// |
// | Unless required by applicable law or agreed to in writing, software
// | distributed under the License is distributed on an "AS IS" BASIS,
// | WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// | See the License for the specific language governing permissions and
// | limitations under the License.
// +-------------------------------------------------------------------------
package com.qingcloud.sdk.service;
import com.fasterxml.jackson.annotation.JsonIgnoreProperties;
import com.qingcloud.sdk.config.EnvContext;
import com.qingcloud.sdk.constants.QCConstant;
import com.qingcloud.sdk.model.OutputModel;
import com.qingcloud.sdk.request.ResponseCallBack;
import com.qingcloud.sdk.request.ResourceRequestFactory;
import com.qingcloud.sdk.exception.QCException;
import com.qingcloud.sdk.model.IaasParamBody;
import com.qingcloud.sdk.service.Types.*;
import com.qingcloud.sdk.utils.QCStringUtil;
import com.fasterxml.jackson.annotation.JsonProperty;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
public class JobService {
private EnvContext envContext;
private String zone;
public JobService(EnvContext envContext) {
this.envContext = envContext;
}
public JobService(EnvContext envContext, String zone) {
this.envContext = envContext;
this.zone = zone;
}
/**
* @param input
* @throws QCException
*
* Documentation URL: https://docs.qingcloud.com/api/job/describe_jobs.html
*/
@SuppressWarnings({"rawtypes", "unchecked"})
public DescribeJobsOutput describeJobs(DescribeJobsInput input) throws QCException {
if (input == null) {
input = new DescribeJobsInput();
}
Map context = new HashMap();
context.put(QCConstant.ENV_CONTEXT_KEY, this.envContext);
context.put("OperationName", "DescribeJobs");
context.put("APIName", "DescribeJobs");
context.put("ServiceName", "DescribeJobs");
context.put("RequestMethod", "GET");
context.put("RequestURI", "/iaas/DescribeJobs");
input.setAction("DescribeJobs");
if (QCStringUtil.isEmpty(this.zone)) {
input.setZone(this.envContext.getZone());
} else {
input.setZone(this.zone);
}
Object backModel = ResourceRequestFactory.getResourceRequest().sendApiRequest(context, input,DescribeJobsOutput.class);
if(backModel != null){
return (DescribeJobsOutput)backModel;
}
return null;
}
/**
* @param input
* @param callback
* @throws QCException
*
* Documentation URL: https://docs.qingcloud.com/api/job/describe_jobs.html
*/
@SuppressWarnings({"rawtypes", "unchecked"})
public void describeJobs(DescribeJobsInput input, ResponseCallBack callback) throws QCException {
if (input == null) {
input = new DescribeJobsInput();
}
Map context = new HashMap();
context.put(QCConstant.ENV_CONTEXT_KEY, this.envContext);
context.put("OperationName", "DescribeJobs");
context.put("APIName", "DescribeJobs");
context.put("ServiceName", "DescribeJobs");
context.put("RequestMethod", "GET");
context.put("RequestURI", "/iaas/DescribeJobs");
input.setAction("DescribeJobs");
if (QCStringUtil.isEmpty(this.zone)) {
input.setZone(this.envContext.getZone());
} else {
input.setZone(this.zone);
}
if(callback == null) {
throw new QCException("callback can't be null");
}
ResourceRequestFactory.getResourceRequest().sendApiRequestAsync(context,input,callback);
}
public static class DescribeJobsInput extends IaasParamBody {
private List jobs;
@JsonProperty(value = "jobs")
public void setJobs(List jobs) {
this.jobs = jobs;
}
@JsonProperty(value = "jobs")
public List getJobs() {
return this.jobs;
}
private Integer limit;
@JsonProperty(value = "limit")
public void setLimit(Integer limit) {
this.limit = limit;
}
@JsonProperty(value = "limit")
public Integer getLimit() {
return this.limit;
}
private Integer offset;
@JsonProperty(value = "offset")
public void setOffset(Integer offset) {
this.offset = offset;
}
@JsonProperty(value = "offset")
public Integer getOffset() {
return this.offset;
}
private List status;
@JsonProperty(value = "status")
public void setStatus(List status) {
this.status = status;
}
@JsonProperty(value = "status")
public List getStatus() {
return this.status;
}
// Verbose's available values: 0
private Integer verbose;
@JsonProperty(value = "verbose")
public void setVerbose(Integer verbose) {
this.verbose = verbose;
}
@JsonProperty(value = "verbose")
public Integer getVerbose() {
return this.verbose;
}
public String validateParam() throws QCException {
String[]verboseValidValues = {"0"};
boolean verboseIsValid = false;
for (String v : verboseValidValues) {
if (v.equals(this.getVerbose()+"")) {
verboseIsValid = true;
}
Boolean verboseIsRequired = Boolean.FALSE;
if (verboseIsRequired.equals(Boolean.FALSE) && this.getVerbose()==null) {
verboseIsValid = true;
}
}
if (!verboseIsValid) {
throw new QCException("Verbose value " + this.getVerbose() + "is invalid");
}
return null;
}
}
@JsonIgnoreProperties(ignoreUnknown = true)
public static class DescribeJobsOutput extends OutputModel {
private String action;
@JsonProperty(value = "action")
public void setAction(String action) {
this.action = action;
}
@JsonProperty(value = "action")
public String getAction() {
return this.action;
}
private List jobSet;
@JsonProperty(value = "job_set")
public void setJobSet(List jobSet) {
this.jobSet = jobSet;
}
@JsonProperty(value = "job_set")
public List getJobSet() {
return this.jobSet;
}
private Integer retCode;
@JsonProperty(value = "ret_code")
public void setRetCode(Integer retCode) {
this.retCode = retCode;
}
@JsonProperty(value = "ret_code")
public Integer getRetCode() {
return this.retCode;
}
private Integer totalCount;
@JsonProperty(value = "total_count")
public void setTotalCount(Integer totalCount) {
this.totalCount = totalCount;
}
@JsonProperty(value = "total_count")
public Integer getTotalCount() {
return this.totalCount;
}
}}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy