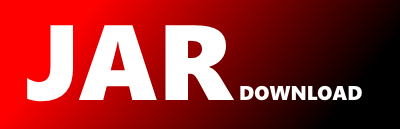
com.qingxun.javasdkapi.clients.OnlineVoiceTransWebSocketClient Maven / Gradle / Ivy
package com.qingxun.javasdkapi.clients;
import com.fasterxml.jackson.core.JsonProcessingException;
import com.qingxun.javasdkapi.response.OnlineMsg;
import org.java_websocket.client.WebSocketClient;
import org.java_websocket.handshake.ServerHandshake;
import java.net.URI;
import java.net.URISyntaxException;
import static com.qingxun.javasdkapi.common.JsonUtil.objectMapper;
/**
* 语音识别翻译
*/
public class OnlineVoiceTransWebSocketClient extends WebSocketClient {
private AbstractOnlineVoiceHandler onlineVoiceHandler;
public static abstract class AbstractOnlineVoiceHandler {
protected void handMessage(String message) {
try {
OnlineMsg onlineMsg = objectMapper.readValue(message, OnlineMsg.class);
handMessage(onlineMsg);
} catch (JsonProcessingException e) {
e.printStackTrace();
}
}
protected abstract void handMessage(OnlineMsg onlineMsg);
protected abstract void handleOpen(ServerHandshake serverHandshake);
protected abstract void handleClose(int i, String s, boolean b);
protected abstract void handleError(Exception e);
}
public OnlineVoiceTransWebSocketClient(String url, AbstractOnlineVoiceHandler onlineVoiceHandler) throws URISyntaxException {
super(new URI(url));
this.onlineVoiceHandler = onlineVoiceHandler;
}
@Override
public void onOpen(ServerHandshake serverHandshake) {
onlineVoiceHandler.handleOpen(serverHandshake);
}
@Override
public void onMessage(String s) {
onlineVoiceHandler.handMessage(s);
}
@Override
public void onClose(int i, String s, boolean b) {
onlineVoiceHandler.handleClose(i, s, b);
}
@Override
public void onError(Exception e) {
onlineVoiceHandler.handleError(e);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy