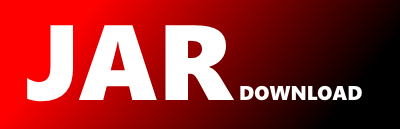
com.qingxun.javasdkapi.request.TextTransRequest Maven / Gradle / Ivy
package com.qingxun.javasdkapi.request;
import org.apache.commons.lang3.StringUtils;
import java.util.TreeMap;
/**
* 文字翻译请求参数
*
* @author cedric
*/
public class TextTransRequest extends BaseRequest {
private String from;
private String to;
private String text;
private TextTransRequest() {
}
public String getFrom() {
return from;
}
public void setFrom(String from) {
this.from = from;
}
public String getTo() {
return to;
}
public void setTo(String to) {
this.to = to;
}
public String getText() {
return text;
}
public void setText(String text) {
this.text = text;
}
@Override
public TreeMap transToMap() {
TreeMap map = new TreeMap();
map.put("from", this.from);
map.put("to", this.to);
map.put("text", this.text);
return map;
}
public TextTransRequest(Builder builder) {
if (StringUtils.isEmpty(builder.from)) {
throw new NullPointerException("from 字段为空");
}
if (StringUtils.isEmpty(builder.to)) {
throw new NullPointerException("to 字段为空");
}
if (StringUtils.isEmpty(builder.text)) {
throw new NullPointerException("text 字段为空");
}
this.from = builder.from;
this.to = builder.to;
this.text = builder.text;
}
public static class Builder {
private String from;
private String to;
private String text;
public Builder setFrom(String from) {
this.from = from;
return this;
}
public Builder setTo(String to) {
this.to = to;
return this;
}
public Builder setText(String text) {
this.text = text;
return this;
}
public TextTransRequest builder() {
return new TextTransRequest(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy