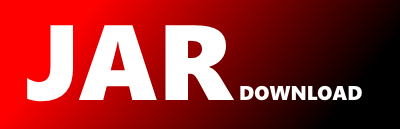
com.qingxun.javasdkapi.request.UploadTransRequest Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-sdk-api Show documentation
Show all versions of java-sdk-api Show documentation
openapi SDK for Java
Copyright © 2023 杭州轻寻科技
All rights reserved.
版权所有 (C)杭州轻寻科技
http://open.qingxun.com
The newest version!
package com.qingxun.javasdkapi.request;
import cn.hutool.crypto.SecureUtil;
import com.qingxun.javasdkapi.utils.InputStreamUtil;
import com.qingxun.javasdkapi.utils.Md5BytesUtil;
import org.apache.commons.lang3.StringUtils;
import java.io.File;
import java.io.InputStream;
import java.util.TreeMap;
/**
* 文件上传请求参数
*
* @author cedric
*/
public class UploadTransRequest extends BaseRequest {
private String from;
private String to;
/**
* md5小写
*/
private String md5;
/**
* 见行业列表
*/
private Integer industryId;
/**
* 0:不翻译文档内图片(默认),
* 1:翻译文档内图片。目前支持中、英、日、韩的文档内图片翻译。(如有需要请联系销售开通)
*/
private Integer transImg;
/**
* 0:只翻译当前打开sheet(默认),
* 1:翻译全部sheet(页数按全部sheet字符数来计算)
*/
private Integer excelMode;
/**
* 0:译文单独为一个文档(默认)
* 1:双语对照(原文和译文在一个文档)
*/
private Integer bilingualControl;
private String fileName;
private byte [] fileBytes;
public String getFrom() {
return from;
}
public String getTo() {
return to;
}
public String getMd5() {
return md5;
}
public Integer getIndustryId() {
return industryId;
}
public Integer getTransImg() {
return transImg;
}
public Integer getExcelMode() {
return excelMode;
}
public Integer getBilingualControl() {
return bilingualControl;
}
public File getFile() {
return file;
}
private File file;
public String getFileName() {
return fileName;
}
public byte[] getFileBytes() {
return fileBytes;
}
private UploadTransRequest() {
}
@Override
public TreeMap transToMap() {
TreeMap map = new TreeMap();
map.put("from", this.from);
map.put("to", this.to);
map.put("md5", this.md5);
if (this.industryId != null) {
map.put("industryId", this.industryId);
}
if (this.transImg != null) {
map.put("transImg", this.transImg);
}
if (this.excelMode != null) {
map.put("excelMode", this.excelMode);
}
if (this.bilingualControl !=null) {
map.put("bilingualControl", this.bilingualControl);
}
return map;
}
public UploadTransRequest(Builder builder) {
if (StringUtils.isEmpty(builder.from)) {
throw new NullPointerException("from 字段为空");
}
if (StringUtils.isEmpty(builder.to)) {
throw new NullPointerException("to 字段为空");
}
if (builder.file == null) {
if (StringUtils.isEmpty(builder.fileName)) {
throw new NullPointerException("file为空");
}
if (builder.fileStream == null ){
throw new NullPointerException("file为空");
}
}
if (StringUtils.isEmpty(builder.md5)) {
if (null != builder.file) {
builder.setMd5(SecureUtil.md5(builder.file));
} else if (null != builder.fileStream){
byte[] bytes = InputStreamUtil.transToBytes(builder.fileStream);
this.fileBytes = bytes;
builder.setMd5(Md5BytesUtil.md5(bytes));
}
}
if (StringUtils.isEmpty(builder.md5)) {
throw new NullPointerException("md5 字段为空");
}
this.from = builder.from;
this.to = builder.to;
this.md5 = builder.md5;
this.file = builder.file;
this.industryId = builder.industryId;
this.transImg = builder.transImg;
this.excelMode = builder.excelMode;
this.bilingualControl = builder.bilingualControl;
this.fileName = builder.fileName;;
}
public static class Builder {
private String from;
private String to;
/**
* md5小写
*/
private String md5;
private File file;
/**
* 见行业列表
*/
private Integer industryId;
/**
* 0:不翻译文档内图片(默认),
* 1:翻译文档内图片。目前支持中、英、日、韩的文档内图片翻译。(如有需要请联系销售开通)
*/
private Integer transImg;
/**
* 0:只翻译当前打开sheet(默认),
* 1:翻译全部sheet(页数按全部sheet字符数来计算)
*/
private Integer excelMode;
/**
* 0:译文单独为一个文档(默认)
* 1:双语对照(原文和译文在一个文档)
*/
private Integer bilingualControl;
private InputStream fileStream;
private String fileName;
public Builder setFrom(String from) {
this.from = from;
return this;
}
public Builder setTo(String to) {
this.to = to;
return this;
}
public Builder setMd5(String md5) {
this.md5 = md5;
return this;
}
public Builder setIndustryId(Integer industryId) {
this.industryId = industryId;
return this;
}
public Builder setTransImg(Integer transImg) {
this.transImg = transImg;
return this;
}
public Builder setExcelMode(Integer excelMode) {
this.excelMode = excelMode;
return this;
}
public Builder setBilingualControl(Integer bilingualControl) {
this.bilingualControl = bilingualControl;
return this;
}
public Builder setFile(File file) {
this.file = file;
return this;
}
public Builder setFileStream(InputStream fileStream) {
this.fileStream = fileStream;
return this;
}
public Builder setFileName(String fileName){
this.fileName = fileName;
return this;
}
public UploadTransRequest builder() {
return new UploadTransRequest(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy