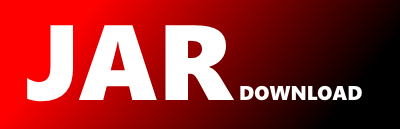
com.qiniu.util.Json Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qiniu-java-sdk Show documentation
Show all versions of qiniu-java-sdk Show documentation
Qiniu Cloud Storage SDK for Java
package com.qiniu.util;
import com.google.gson.Gson;
import com.google.gson.GsonBuilder;
import com.google.gson.JsonElement;
import com.google.gson.reflect.TypeToken;
import java.lang.reflect.Type;
import java.util.Map;
public final class Json {
private Json() {
}
public static String encode(StringMap map) {
return new Gson().toJson(map.map());
}
public static String encode(Object obj) {
return new GsonBuilder().serializeNulls().create().toJson(obj);
}
public static T decode(String json, Class classOfT) {
return new Gson().fromJson(json, classOfT);
}
public static T decode(JsonElement jsonElement, Class clazz) {
Gson gson = new Gson();
return gson.fromJson(jsonElement, clazz);
}
public static StringMap decode(String json) {
// CHECKSTYLE:OFF
Type t = new TypeToken
© 2015 - 2024 Weber Informatics LLC | Privacy Policy