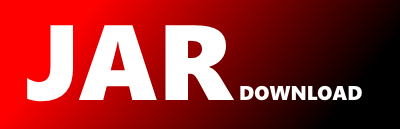
com.qiniu.service.convert.FileInfoToMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qsuits Show documentation
Show all versions of qsuits Show documentation
qiniu-suits is a efficient tools for qiniu api implemented by java8.
package com.qiniu.service.convert;
import com.qiniu.service.interfaces.ITypeConvert;
import com.qiniu.storage.model.FileInfo;
import java.util.*;
import java.util.stream.Collectors;
public class FileInfoToMap implements ITypeConvert> {
final private List fileInfoFields = new ArrayList(){{
add("key");
add("hash");
add("fsize");
add("putTime");
add("mimeType");
add("type");
add("status");
add("endUser");
}};
private List errorList = new ArrayList<>();
public List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy