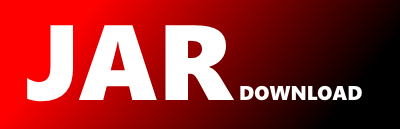
com.qiniu.process.qoss.FileChecker Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qsuits Show documentation
Show all versions of qsuits Show documentation
qiniu-suits is a efficient tools for qiniu api implemented by java8.
package com.qiniu.process.qoss;
import com.google.gson.*;
import com.qiniu.common.QiniuException;
import com.qiniu.http.Client;
import com.qiniu.http.Response;
import com.qiniu.model.qoss.Qhash;
import com.qiniu.storage.Configuration;
import java.util.ArrayList;
import java.util.List;
public class FileChecker {
private Client client;
private String algorithm;
private String protocol;
final private List algorithms = new ArrayList(){{
add("md5");
add("sha1");
}};
public FileChecker() {
}
public FileChecker(String algorithm, String protocol) {
this.client = new Client();
this.algorithm = algorithms.contains(algorithm) ? algorithm : "md5";
this.protocol = "https".equals(protocol)? "https" : "http";
}
public FileChecker(Configuration configuration, String algorithm, String protocol) {
this.client = new Client(configuration);
this.algorithm = algorithms.contains(algorithm) ? algorithm : "md5";
this.protocol = "https".equals(protocol)? "https" : "http";
}
public Qhash getQHash(String url) throws QiniuException {
return getQHashByJson(getQHashBody(url));
}
public Qhash getQHash(String domain, String sourceKey) throws QiniuException {
return getQHashByJson(getQHashBody(domain, sourceKey));
}
public Qhash getQHashByJson(String qHashJson) throws QiniuException {
Qhash qhash;
try {
Gson gson = new Gson();
qhash = gson.fromJson(qHashJson, Qhash.class);
} catch (JsonParseException e) {
throw new QiniuException(e, e.getMessage());
}
return qhash;
}
public Qhash getQHashByJson(JsonObject qHashJson) throws QiniuException {
Qhash qhash;
try {
Gson gson = new Gson();
qhash = gson.fromJson(qHashJson, Qhash.class);
} catch (JsonParseException e) {
throw new QiniuException(e, e.getMessage());
}
return qhash;
}
public JsonObject getQHashJson(String domain, String sourceKey) throws QiniuException {
JsonParser jsonParser = new JsonParser();
return jsonParser.parse(getQHashBody(domain, sourceKey)).getAsJsonObject();
}
public String getQHashBody(String domain, String sourceKey) throws QiniuException {
String url = protocol + "://" + domain + "/" + sourceKey.split("\\?")[0];
return getQHashBody(url);
}
public String getQHashBody(String url) throws QiniuException {
Response response = client.get(url + "?qhash/" + algorithm);
String qhash = response.bodyString();
if (response.statusCode != 200) throw new QiniuException(response);
response.close();
return qhash;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy