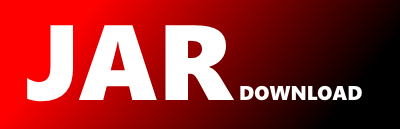
com.qiniu.process.qoss.AsyncFetch Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qsuits Show documentation
Show all versions of qsuits Show documentation
qiniu-suits is a efficient tools for qiniu api implemented by java8.
package com.qiniu.process.qoss;
import com.qiniu.common.QiniuException;
import com.qiniu.http.Response;
import com.qiniu.process.Base;
import com.qiniu.storage.BucketManager;
import com.qiniu.storage.Configuration;
import com.qiniu.util.*;
import java.io.IOException;
import java.util.Map;
public class AsyncFetch extends Base {
private String domain;
private String protocol;
private String urlIndex;
private String keyPrefix;
private boolean hasCustomArgs;
private String host;
private String md5Index;
private String callbackUrl;
private String callbackBody;
private String callbackBodyType;
private String callbackHost;
private int fileType;
private boolean ignoreSameKey;
private BucketManager bucketManager;
public AsyncFetch(String accessKey, String secretKey, Configuration configuration, String bucket, String domain,
String protocol, String urlIndex, String keyPrefix, String rmPrefix, String savePath,
int saveIndex) throws IOException {
super("asyncfetch", accessKey, secretKey, configuration, bucket, rmPrefix, savePath, saveIndex);
set(domain, protocol, urlIndex, keyPrefix);
this.bucketManager = new BucketManager(Auth.create(accessKey, secretKey), configuration.clone());
}
public void updateFetch(String bucket, String domain, String protocol, String urlIndex, String keyPrefix,
String rmPrefix) throws IOException {
this.bucket = bucket;
set(domain, protocol, urlIndex, keyPrefix);
this.rmPrefix = rmPrefix;
}
private void set(String domain, String protocol, String urlIndex, String keyPrefix) throws IOException {
if (urlIndex == null || "".equals(urlIndex)) {
this.urlIndex = null;
if (domain == null || "".equals(domain)) {
throw new IOException("please set one of domain and urlIndex.");
} else {
RequestUtils.checkHost(domain);
this.domain = domain;
this.protocol = protocol == null || !protocol.matches("(http|https)") ? "http" : protocol;
}
} else {
this.urlIndex = urlIndex;
}
this.keyPrefix = keyPrefix == null ? "" : keyPrefix;
}
public AsyncFetch(String accessKey, String secretKey, Configuration configuration, String bucket, String domain,
String protocol, String urlIndex, String keyPrefix, String rmPrefix, String savePath)
throws IOException {
this(accessKey, secretKey, configuration, bucket, domain, protocol, urlIndex, keyPrefix, rmPrefix,
savePath, 0);
}
public void setFetchArgs(String host, String md5Index, String callbackUrl, String callbackBody,
String callbackBodyType, String callbackHost, int fileType, boolean ignoreSameKey) {
this.host = host;
this.md5Index = md5Index == null ? "" : md5Index;
this.callbackUrl = callbackUrl;
this.callbackBody = callbackBody;
this.callbackBodyType = callbackBodyType;
this.callbackHost = callbackHost;
this.fileType = fileType;
this.ignoreSameKey = ignoreSameKey;
this.hasCustomArgs = true;
}
public AsyncFetch clone() throws CloneNotSupportedException {
AsyncFetch asyncFetch = (AsyncFetch)super.clone();
asyncFetch.bucketManager = new BucketManager(Auth.create(accessKey, secretKey), configuration.clone());
return asyncFetch;
}
private Response fetch(String url, String key, String md5, String etag) throws QiniuException {
return hasCustomArgs ?
bucketManager.asynFetch(url, bucket, key, md5, etag, callbackUrl, callbackBody, callbackBodyType,
callbackHost, String.valueOf(fileType)) :
bucketManager.asynFetch(url, bucket, key);
}
@Override
protected Map formatLine(Map line) throws IOException {
if (urlIndex == null) {
line.put("key", FileNameUtils.rmPrefix(rmPrefix, line.get("key")));
urlIndex = "url";
line.put(urlIndex, protocol + "://" + domain + "/" + line.get("key").replaceAll("\\?", "%3F"));
} else {
line.put("key", URLUtils.getKey(line.get(urlIndex)));
}
return line;
}
@Override
protected String resultInfo(Map line) {
return line.get("key") + "\t" + line.get(urlIndex);
}
@Override
protected String singleResult(Map line) throws QiniuException {
Response response = fetch(line.get(urlIndex), keyPrefix + line.get("key"), line.get(md5Index), line.get("hash"));
return line.get(urlIndex) + "\t" + HttpResponseUtils.responseJson(response);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy