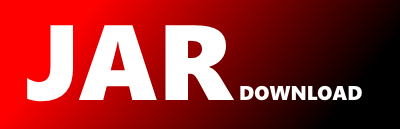
com.qiniu.util.LineUtils Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qsuits Show documentation
Show all versions of qsuits Show documentation
qiniu-suits is a efficient tools for qiniu api implemented by java8.
package com.qiniu.util;
import com.google.gson.JsonNull;
import com.google.gson.JsonObject;
import com.google.gson.JsonParser;
import com.qiniu.storage.model.FileInfo;
import java.io.IOException;
import java.util.*;
public class LineUtils {
final static private List longFields = new ArrayList(){{
add("fsize");
add("putTime");
}};
final static private List intFields = new ArrayList(){{
add("type");
add("status");
}};
final static public List fileInfoFields = new ArrayList(){{
add("key");
add("hash");
addAll(longFields);
add("mimeType");
addAll(intFields);
add("md5");
add("endUser");
}};
public static Map getItemMap(FileInfo fileInfo, Map indexMap) throws IOException {
if (fileInfo == null || fileInfo.key == null) throw new IOException("empty file info.");
Map itemMap = new HashMap<>();
fileInfoFields.forEach(key -> {
if (indexMap.get(key) != null) {
switch (key) {
case "key": itemMap.put(indexMap.get(key), fileInfo.key); break;
case "hash": itemMap.put(indexMap.get(key), fileInfo.hash); break;
case "fsize": itemMap.put(indexMap.get(key), String.valueOf(fileInfo.fsize)); break;
case "putTime": itemMap.put(indexMap.get(key), String.valueOf(fileInfo.putTime)); break;
case "mimeType": itemMap.put(indexMap.get(key), fileInfo.mimeType); break;
case "type": itemMap.put(indexMap.get(key), String.valueOf(fileInfo.type)); break;
case "status": itemMap.put(indexMap.get(key), String.valueOf(fileInfo.status)); break;
// case "md5": itemMap.put(key, String.valueOf(fileInfo.md5)); break;
case "endUser": itemMap.put(indexMap.get(key), fileInfo.endUser); break;
}
}
});
return itemMap;
}
public static Map getItemMap(JsonObject json, Map indexMap, boolean force)
throws IOException {
if (indexMap == null || indexMap.size() == 0) throw new IOException("no index map to get.");
Map itemMap = new HashMap<>();
String mapKey;
for (String key : json.keySet()) {
mapKey = indexMap.get(key);
if (mapKey != null) {
if (json.get(key) instanceof JsonNull) itemMap.put(mapKey, null);
else itemMap.put(mapKey, json.get(key).getAsString());
}
}
// 是否需要强制转换,即使字段数没有达到 indexMap 的要求
if (!force && itemMap.size() < indexMap.size())
throw new IOException("no enough indexes in line. The parameter indexes may have incorrect order or name.");
return itemMap;
}
public static Map getItemMap(String line, Map indexMap, boolean force)
throws IOException {
JsonObject parsed = new JsonParser().parse(line).getAsJsonObject();
return getItemMap(parsed ,indexMap, force);
}
public static Map getItemMap(String line, String separator, Map indexMap,
boolean force) throws IOException {
if (indexMap == null || indexMap.size() == 0) throw new IOException("no index map to get.");
String[] items = line.split(separator);
Map itemMap = new HashMap<>();
String mapKey;
for (int i = 0; i < items.length; i++) {
mapKey = indexMap.get(String.valueOf(i));
if (mapKey != null) {
if (items[i] == null) itemMap.put(mapKey, null);
else itemMap.put(mapKey, items[i]);
}
}
// 是否需要强制转换,即使字段数没有达到 indexMap 的要求
if (!force && itemMap.size() < indexMap.size())
throw new IOException("no enough indexes in line. The parameter indexes may have incorrect order or name.");
return itemMap;
}
public static String toFormatString(FileInfo fileInfo, String separator, List rmFields) throws IOException {
StringBuilder converted = new StringBuilder();
if (rmFields == null || !rmFields.contains("key")) converted.append(fileInfo.key).append(separator);
if (rmFields == null || !rmFields.contains("hash")) converted.append(fileInfo.hash).append(separator);
if (rmFields == null || !rmFields.contains("fsize")) converted.append(fileInfo.fsize).append(separator);
if (rmFields == null || !rmFields.contains("putTime")) converted.append(fileInfo.putTime).append(separator);
if (rmFields == null || !rmFields.contains("mimeType")) converted.append(fileInfo.mimeType).append(separator);
if (rmFields == null || !rmFields.contains("type")) converted.append(fileInfo.type).append(separator);
if (rmFields == null || !rmFields.contains("status")) converted.append(fileInfo.status).append(separator);
// if (rmFields == null || !rmFields.contains("md5")) converted.append(fileInfo.md5).append(separator);
if ((rmFields == null || !rmFields.contains("endUser")) && fileInfo.endUser != null)
converted.append(fileInfo.endUser).append(separator);
if (converted.length() < separator.length()) throw new IOException("empty result.");
return converted.deleteCharAt(converted.length() - separator.length()).toString();
}
public static String toFormatString(FileInfo fileInfo, List rmFields) throws IOException {
JsonObject converted = new JsonObject();
if (rmFields == null || !rmFields.contains("key")) converted.addProperty("key", fileInfo.key);
if (rmFields == null || !rmFields.contains("hash")) converted.addProperty("hash", fileInfo.hash);
if (rmFields == null || !rmFields.contains("fsize")) converted.addProperty("fsize", fileInfo.fsize);
if (rmFields == null || !rmFields.contains("putTime")) converted.addProperty("putTime", fileInfo.putTime);
if (rmFields == null || !rmFields.contains("mimeType")) converted.addProperty("mimeType", fileInfo.mimeType);
if (rmFields == null || !rmFields.contains("type")) converted.addProperty("type", fileInfo.type);
if (rmFields == null || !rmFields.contains("status")) converted.addProperty("status", fileInfo.status);
// if (rmFields == null || !rmFields.contains("md5")) converted.addProperty("md5", fileInfo.md5);
if ((rmFields == null || !rmFields.contains("endUser")) && fileInfo.endUser != null)
converted.addProperty("endUser", fileInfo.endUser);
if (converted.size() == 0) throw new IOException("empty result.");
return converted.toString();
}
public static String toFormatString(JsonObject json, String separator, List rmFields) throws IOException {
StringBuilder converted = new StringBuilder();
Set set = json.keySet();
List keys = new ArrayList(){{
this.addAll(set);
}};
if (rmFields != null) keys.removeAll(rmFields);
fileInfoFields.forEach(key -> {
if (keys.contains(key) && !(json.get(key) instanceof JsonNull)) {
if (longFields.contains(key)) converted.append(json.get(key).getAsLong()).append(separator);
else if (intFields.contains(key)) converted.append(json.get(key).getAsInt()).append(separator);
else converted.append(json.get(key).getAsString()).append(separator);
}
keys.remove(key);
});
for (String key : keys) {
converted.append(json.get(key).getAsString()).append(separator);
}
if (converted.length() < separator.length()) throw new IOException("empty result.");
return converted.deleteCharAt(converted.length() - separator.length()).toString();
}
public static String toFormatString(Map line, List rmFields) throws IOException {
JsonObject converted = new JsonObject();
Set set = line.keySet();
List keys = new ArrayList(){{
this.addAll(set);
}};
if (rmFields != null) keys.removeAll(rmFields);
fileInfoFields.forEach(key -> {
if (keys.contains(key) && line.get(key) != null) {
if (longFields.contains(key)) converted.addProperty(key, Long.valueOf(line.get(key)));
else if (intFields.contains(key)) converted.addProperty(key, Integer.valueOf(line.get(key)));
else converted.addProperty(key, line.get(key));
}
keys.remove(key);
});
for (String key : keys) {
converted.addProperty(key, line.get(key));
}
if (converted.size() == 0) throw new IOException("empty result.");
return converted.toString();
}
public static String toFormatString(Map line, String separator, List rmFields)
throws IOException {
StringBuilder converted = new StringBuilder();
Set set = line.keySet();
List keys = new ArrayList(){{
this.addAll(set);
}};
if (rmFields != null) keys.removeAll(rmFields);
fileInfoFields.forEach(key -> {
if (keys.contains(key) && line.get(key) != null) {
converted.append(line.get(key)).append(separator);
}
keys.remove(key);
});
for (String key : keys) {
converted.append(line.get(key)).append(separator);
}
if (converted.length() < separator.length()) throw new IOException("empty result.");
return converted.deleteCharAt(converted.length() - separator.length()).toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy