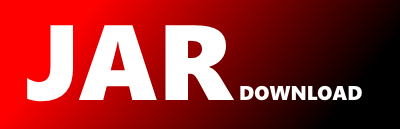
com.qiniu.process.qoss.MoveFile Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qsuits Show documentation
Show all versions of qsuits Show documentation
qiniu-suits is a efficient tools for qiniu api implemented by java8.
package com.qiniu.process.qoss;
import com.qiniu.common.QiniuException;
import com.qiniu.process.Base;
import com.qiniu.storage.BucketManager;
import com.qiniu.storage.BucketManager.*;
import com.qiniu.storage.Configuration;
import com.qiniu.util.Auth;
import com.qiniu.util.FileNameUtils;
import com.qiniu.util.HttpResponseUtils;
import java.io.IOException;
import java.util.List;
import java.util.Map;
public class MoveFile extends Base {
private String toBucket;
private String newKeyIndex;
private String addPrefix;
private String rmPrefix;
private BatchOperations batchOperations;
private BucketManager bucketManager;
public MoveFile(String accessKey, String secretKey, Configuration configuration, String bucket, String toBucket,
String newKeyIndex, String addPrefix, boolean forceIfOnlyPrefix, String rmPrefix, String savePath,
int saveIndex) throws IOException {
// 目标 bucket 为空时规定为 rename 操作
super(toBucket == null || "".equals(toBucket) ? "rename" : "move", accessKey, secretKey, configuration, bucket,
savePath, saveIndex);
set(toBucket, newKeyIndex, addPrefix, forceIfOnlyPrefix, rmPrefix);
this.batchSize = 1000;
this.batchOperations = new BatchOperations();
this.bucketManager = new BucketManager(Auth.create(accessKey, secretKey), configuration.clone());
}
public void updateMove(String bucket, String toBucket, String newKeyIndex, String addPrefix,
boolean forceIfOnlyPrefix, String rmPrefix) throws IOException {
this.bucket = bucket;
set(toBucket, newKeyIndex, addPrefix, forceIfOnlyPrefix, rmPrefix);
}
private void set(String toBucket, String newKeyIndex, String addPrefix, boolean forceIfOnlyPrefix, String rmPrefix)
throws IOException {
this.toBucket = toBucket;
if (newKeyIndex == null || "".equals(newKeyIndex)) {
this.newKeyIndex = "key";
if (toBucket == null || "".equals(toBucket)) {
// rename 操作时未设置 new-key 的条件判断
if (forceIfOnlyPrefix) {
if (addPrefix == null || "".equals(addPrefix))
throw new IOException("although prefix-force is true, but the add-prefix is empty.");
} else {
throw new IOException("there is no newKey index, if you only want to add prefix for renaming, " +
"please set the \"prefix-force\" as true.");
}
}
} else {
this.newKeyIndex = newKeyIndex;
}
this.addPrefix = addPrefix == null ? "" : addPrefix;
this.rmPrefix = rmPrefix == null ? "" : rmPrefix;
}
public MoveFile(String accessKey, String secretKey, Configuration configuration, String bucket, String toBucket,
String newKeyIndex, String keyPrefix, boolean forceIfOnlyPrefix, String rmPrefix, String savePath)
throws IOException {
this(accessKey, secretKey, configuration, bucket, toBucket, newKeyIndex, keyPrefix, forceIfOnlyPrefix, rmPrefix,
savePath, 0);
}
public MoveFile clone() throws CloneNotSupportedException {
MoveFile moveFile = (MoveFile)super.clone();
moveFile.bucketManager = new BucketManager(Auth.create(accessKey, secretKey), configuration.clone());
if (batchSize > 1) moveFile.batchOperations = new BatchOperations();
return moveFile;
}
@Override
protected String resultInfo(Map line) {
return line.get("key") + "\t" + line.get(newKeyIndex);
}
@Override
synchronized protected String batchResult(List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy