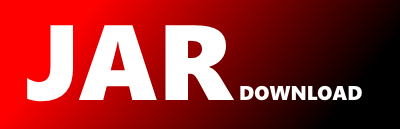
com.qiniu.datasource.LocalFileContainer Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qsuits Show documentation
Show all versions of qsuits Show documentation
qiniu-suits is a efficient tools for qiniu api implemented by java8.
package com.qiniu.datasource;
import com.qiniu.convert.LineToMap;
import com.qiniu.convert.MapToString;
import com.qiniu.interfaces.IReader;
import com.qiniu.interfaces.ITypeConvert;
import com.qiniu.persistence.FileSaveMapper;
import com.qiniu.interfaces.IResultOutput;
import com.qiniu.util.FileUtils;
import java.io.*;
import java.util.*;
public class LocalFileContainer extends FileContainer> {
public LocalFileContainer(String filePath, String parseFormat, String separator, String addKeyPrefix,
String rmKeyPrefix, Map linesMap, Map indexMap,
List fields, int unitLen, int threads) throws IOException {
super(filePath, parseFormat, separator, addKeyPrefix, rmKeyPrefix, linesMap, indexMap, fields, unitLen, threads);
}
@Override
protected ITypeConvert> getNewConverter() throws IOException {
return new LineToMap(parse, separator, addKeyPrefix, rmKeyPrefix, indexMap);
}
@Override
protected ITypeConvert
© 2015 - 2025 Weber Informatics LLC | Privacy Policy