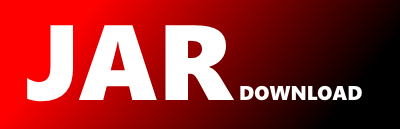
polyglot.ConfigProto Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of qaf-support-grpc Show documentation
Show all versions of qaf-support-grpc Show documentation
QAF support library for grpc test automation
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// source: config.proto
package polyglot;
public final class ConfigProto {
private ConfigProto() {}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistryLite registry) {
}
public static void registerAllExtensions(
com.google.protobuf.ExtensionRegistry registry) {
registerAllExtensions(
(com.google.protobuf.ExtensionRegistryLite) registry);
}
public interface ConfigurationSetOrBuilder extends
// @@protoc_insertion_point(interface_extends:polyglot.ConfigurationSet)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .polyglot.Configuration configurations = 1;
*/
java.util.List
getConfigurationsList();
/**
* repeated .polyglot.Configuration configurations = 1;
*/
polyglot.ConfigProto.Configuration getConfigurations(int index);
/**
* repeated .polyglot.Configuration configurations = 1;
*/
int getConfigurationsCount();
/**
* repeated .polyglot.Configuration configurations = 1;
*/
java.util.List extends polyglot.ConfigProto.ConfigurationOrBuilder>
getConfigurationsOrBuilderList();
/**
* repeated .polyglot.Configuration configurations = 1;
*/
polyglot.ConfigProto.ConfigurationOrBuilder getConfigurationsOrBuilder(
int index);
}
/**
*
* Holds multiple named configurations. By default, the first configuration is
* used, but a command line flag can have Polyglot use a specific config.
*
*
* Protobuf type {@code polyglot.ConfigurationSet}
*/
public static final class ConfigurationSet extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:polyglot.ConfigurationSet)
ConfigurationSetOrBuilder {
private static final long serialVersionUID = 0L;
// Use ConfigurationSet.newBuilder() to construct.
private ConfigurationSet(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ConfigurationSet() {
configurations_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ConfigurationSet();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ConfigurationSet(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
configurations_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
configurations_.add(
input.readMessage(polyglot.ConfigProto.Configuration.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
configurations_ = java.util.Collections.unmodifiableList(configurations_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return polyglot.ConfigProto.internal_static_polyglot_ConfigurationSet_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return polyglot.ConfigProto.internal_static_polyglot_ConfigurationSet_fieldAccessorTable
.ensureFieldAccessorsInitialized(
polyglot.ConfigProto.ConfigurationSet.class, polyglot.ConfigProto.ConfigurationSet.Builder.class);
}
public static final int CONFIGURATIONS_FIELD_NUMBER = 1;
private java.util.List configurations_;
/**
* repeated .polyglot.Configuration configurations = 1;
*/
@java.lang.Override
public java.util.List getConfigurationsList() {
return configurations_;
}
/**
* repeated .polyglot.Configuration configurations = 1;
*/
@java.lang.Override
public java.util.List extends polyglot.ConfigProto.ConfigurationOrBuilder>
getConfigurationsOrBuilderList() {
return configurations_;
}
/**
* repeated .polyglot.Configuration configurations = 1;
*/
@java.lang.Override
public int getConfigurationsCount() {
return configurations_.size();
}
/**
* repeated .polyglot.Configuration configurations = 1;
*/
@java.lang.Override
public polyglot.ConfigProto.Configuration getConfigurations(int index) {
return configurations_.get(index);
}
/**
* repeated .polyglot.Configuration configurations = 1;
*/
@java.lang.Override
public polyglot.ConfigProto.ConfigurationOrBuilder getConfigurationsOrBuilder(
int index) {
return configurations_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < configurations_.size(); i++) {
output.writeMessage(1, configurations_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < configurations_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, configurations_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof polyglot.ConfigProto.ConfigurationSet)) {
return super.equals(obj);
}
polyglot.ConfigProto.ConfigurationSet other = (polyglot.ConfigProto.ConfigurationSet) obj;
if (!getConfigurationsList()
.equals(other.getConfigurationsList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getConfigurationsCount() > 0) {
hash = (37 * hash) + CONFIGURATIONS_FIELD_NUMBER;
hash = (53 * hash) + getConfigurationsList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static polyglot.ConfigProto.ConfigurationSet parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.ConfigurationSet parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.ConfigurationSet parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.ConfigurationSet parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.ConfigurationSet parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.ConfigurationSet parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.ConfigurationSet parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.ConfigurationSet parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static polyglot.ConfigProto.ConfigurationSet parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.ConfigurationSet parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static polyglot.ConfigProto.ConfigurationSet parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.ConfigurationSet parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(polyglot.ConfigProto.ConfigurationSet prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Holds multiple named configurations. By default, the first configuration is
* used, but a command line flag can have Polyglot use a specific config.
*
*
* Protobuf type {@code polyglot.ConfigurationSet}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:polyglot.ConfigurationSet)
polyglot.ConfigProto.ConfigurationSetOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return polyglot.ConfigProto.internal_static_polyglot_ConfigurationSet_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return polyglot.ConfigProto.internal_static_polyglot_ConfigurationSet_fieldAccessorTable
.ensureFieldAccessorsInitialized(
polyglot.ConfigProto.ConfigurationSet.class, polyglot.ConfigProto.ConfigurationSet.Builder.class);
}
// Construct using polyglot.ConfigProto.ConfigurationSet.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getConfigurationsFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
if (configurationsBuilder_ == null) {
configurations_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
configurationsBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return polyglot.ConfigProto.internal_static_polyglot_ConfigurationSet_descriptor;
}
@java.lang.Override
public polyglot.ConfigProto.ConfigurationSet getDefaultInstanceForType() {
return polyglot.ConfigProto.ConfigurationSet.getDefaultInstance();
}
@java.lang.Override
public polyglot.ConfigProto.ConfigurationSet build() {
polyglot.ConfigProto.ConfigurationSet result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public polyglot.ConfigProto.ConfigurationSet buildPartial() {
polyglot.ConfigProto.ConfigurationSet result = new polyglot.ConfigProto.ConfigurationSet(this);
int from_bitField0_ = bitField0_;
if (configurationsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
configurations_ = java.util.Collections.unmodifiableList(configurations_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.configurations_ = configurations_;
} else {
result.configurations_ = configurationsBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof polyglot.ConfigProto.ConfigurationSet) {
return mergeFrom((polyglot.ConfigProto.ConfigurationSet)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(polyglot.ConfigProto.ConfigurationSet other) {
if (other == polyglot.ConfigProto.ConfigurationSet.getDefaultInstance()) return this;
if (configurationsBuilder_ == null) {
if (!other.configurations_.isEmpty()) {
if (configurations_.isEmpty()) {
configurations_ = other.configurations_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureConfigurationsIsMutable();
configurations_.addAll(other.configurations_);
}
onChanged();
}
} else {
if (!other.configurations_.isEmpty()) {
if (configurationsBuilder_.isEmpty()) {
configurationsBuilder_.dispose();
configurationsBuilder_ = null;
configurations_ = other.configurations_;
bitField0_ = (bitField0_ & ~0x00000001);
configurationsBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getConfigurationsFieldBuilder() : null;
} else {
configurationsBuilder_.addAllMessages(other.configurations_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
polyglot.ConfigProto.ConfigurationSet parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (polyglot.ConfigProto.ConfigurationSet) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.util.List configurations_ =
java.util.Collections.emptyList();
private void ensureConfigurationsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
configurations_ = new java.util.ArrayList(configurations_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
polyglot.ConfigProto.Configuration, polyglot.ConfigProto.Configuration.Builder, polyglot.ConfigProto.ConfigurationOrBuilder> configurationsBuilder_;
/**
* repeated .polyglot.Configuration configurations = 1;
*/
public java.util.List getConfigurationsList() {
if (configurationsBuilder_ == null) {
return java.util.Collections.unmodifiableList(configurations_);
} else {
return configurationsBuilder_.getMessageList();
}
}
/**
* repeated .polyglot.Configuration configurations = 1;
*/
public int getConfigurationsCount() {
if (configurationsBuilder_ == null) {
return configurations_.size();
} else {
return configurationsBuilder_.getCount();
}
}
/**
* repeated .polyglot.Configuration configurations = 1;
*/
public polyglot.ConfigProto.Configuration getConfigurations(int index) {
if (configurationsBuilder_ == null) {
return configurations_.get(index);
} else {
return configurationsBuilder_.getMessage(index);
}
}
/**
* repeated .polyglot.Configuration configurations = 1;
*/
public Builder setConfigurations(
int index, polyglot.ConfigProto.Configuration value) {
if (configurationsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureConfigurationsIsMutable();
configurations_.set(index, value);
onChanged();
} else {
configurationsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .polyglot.Configuration configurations = 1;
*/
public Builder setConfigurations(
int index, polyglot.ConfigProto.Configuration.Builder builderForValue) {
if (configurationsBuilder_ == null) {
ensureConfigurationsIsMutable();
configurations_.set(index, builderForValue.build());
onChanged();
} else {
configurationsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .polyglot.Configuration configurations = 1;
*/
public Builder addConfigurations(polyglot.ConfigProto.Configuration value) {
if (configurationsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureConfigurationsIsMutable();
configurations_.add(value);
onChanged();
} else {
configurationsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .polyglot.Configuration configurations = 1;
*/
public Builder addConfigurations(
int index, polyglot.ConfigProto.Configuration value) {
if (configurationsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureConfigurationsIsMutable();
configurations_.add(index, value);
onChanged();
} else {
configurationsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .polyglot.Configuration configurations = 1;
*/
public Builder addConfigurations(
polyglot.ConfigProto.Configuration.Builder builderForValue) {
if (configurationsBuilder_ == null) {
ensureConfigurationsIsMutable();
configurations_.add(builderForValue.build());
onChanged();
} else {
configurationsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .polyglot.Configuration configurations = 1;
*/
public Builder addConfigurations(
int index, polyglot.ConfigProto.Configuration.Builder builderForValue) {
if (configurationsBuilder_ == null) {
ensureConfigurationsIsMutable();
configurations_.add(index, builderForValue.build());
onChanged();
} else {
configurationsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .polyglot.Configuration configurations = 1;
*/
public Builder addAllConfigurations(
java.lang.Iterable extends polyglot.ConfigProto.Configuration> values) {
if (configurationsBuilder_ == null) {
ensureConfigurationsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, configurations_);
onChanged();
} else {
configurationsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .polyglot.Configuration configurations = 1;
*/
public Builder clearConfigurations() {
if (configurationsBuilder_ == null) {
configurations_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
configurationsBuilder_.clear();
}
return this;
}
/**
* repeated .polyglot.Configuration configurations = 1;
*/
public Builder removeConfigurations(int index) {
if (configurationsBuilder_ == null) {
ensureConfigurationsIsMutable();
configurations_.remove(index);
onChanged();
} else {
configurationsBuilder_.remove(index);
}
return this;
}
/**
* repeated .polyglot.Configuration configurations = 1;
*/
public polyglot.ConfigProto.Configuration.Builder getConfigurationsBuilder(
int index) {
return getConfigurationsFieldBuilder().getBuilder(index);
}
/**
* repeated .polyglot.Configuration configurations = 1;
*/
public polyglot.ConfigProto.ConfigurationOrBuilder getConfigurationsOrBuilder(
int index) {
if (configurationsBuilder_ == null) {
return configurations_.get(index); } else {
return configurationsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .polyglot.Configuration configurations = 1;
*/
public java.util.List extends polyglot.ConfigProto.ConfigurationOrBuilder>
getConfigurationsOrBuilderList() {
if (configurationsBuilder_ != null) {
return configurationsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(configurations_);
}
}
/**
* repeated .polyglot.Configuration configurations = 1;
*/
public polyglot.ConfigProto.Configuration.Builder addConfigurationsBuilder() {
return getConfigurationsFieldBuilder().addBuilder(
polyglot.ConfigProto.Configuration.getDefaultInstance());
}
/**
* repeated .polyglot.Configuration configurations = 1;
*/
public polyglot.ConfigProto.Configuration.Builder addConfigurationsBuilder(
int index) {
return getConfigurationsFieldBuilder().addBuilder(
index, polyglot.ConfigProto.Configuration.getDefaultInstance());
}
/**
* repeated .polyglot.Configuration configurations = 1;
*/
public java.util.List
getConfigurationsBuilderList() {
return getConfigurationsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
polyglot.ConfigProto.Configuration, polyglot.ConfigProto.Configuration.Builder, polyglot.ConfigProto.ConfigurationOrBuilder>
getConfigurationsFieldBuilder() {
if (configurationsBuilder_ == null) {
configurationsBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
polyglot.ConfigProto.Configuration, polyglot.ConfigProto.Configuration.Builder, polyglot.ConfigProto.ConfigurationOrBuilder>(
configurations_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
configurations_ = null;
}
return configurationsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:polyglot.ConfigurationSet)
}
// @@protoc_insertion_point(class_scope:polyglot.ConfigurationSet)
private static final polyglot.ConfigProto.ConfigurationSet DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new polyglot.ConfigProto.ConfigurationSet();
}
public static polyglot.ConfigProto.ConfigurationSet getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ConfigurationSet parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ConfigurationSet(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public polyglot.ConfigProto.ConfigurationSet getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ConfigurationOrBuilder extends
// @@protoc_insertion_point(interface_extends:polyglot.Configuration)
com.google.protobuf.MessageOrBuilder {
/**
* string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
* string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* .polyglot.CallConfiguration call_config = 2;
* @return Whether the callConfig field is set.
*/
boolean hasCallConfig();
/**
* .polyglot.CallConfiguration call_config = 2;
* @return The callConfig.
*/
polyglot.ConfigProto.CallConfiguration getCallConfig();
/**
* .polyglot.CallConfiguration call_config = 2;
*/
polyglot.ConfigProto.CallConfigurationOrBuilder getCallConfigOrBuilder();
/**
* .polyglot.ProtoConfiguration proto_config = 3;
* @return Whether the protoConfig field is set.
*/
boolean hasProtoConfig();
/**
* .polyglot.ProtoConfiguration proto_config = 3;
* @return The protoConfig.
*/
polyglot.ConfigProto.ProtoConfiguration getProtoConfig();
/**
* .polyglot.ProtoConfiguration proto_config = 3;
*/
polyglot.ConfigProto.ProtoConfigurationOrBuilder getProtoConfigOrBuilder();
/**
* .polyglot.OutputConfiguration output_config = 4;
* @return Whether the outputConfig field is set.
*/
boolean hasOutputConfig();
/**
* .polyglot.OutputConfiguration output_config = 4;
* @return The outputConfig.
*/
polyglot.ConfigProto.OutputConfiguration getOutputConfig();
/**
* .polyglot.OutputConfiguration output_config = 4;
*/
polyglot.ConfigProto.OutputConfigurationOrBuilder getOutputConfigOrBuilder();
}
/**
*
* Holds parameters used by Polylgot at runtime.
*
*
* Protobuf type {@code polyglot.Configuration}
*/
public static final class Configuration extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:polyglot.Configuration)
ConfigurationOrBuilder {
private static final long serialVersionUID = 0L;
// Use Configuration.newBuilder() to construct.
private Configuration(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private Configuration() {
name_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new Configuration();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private Configuration(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 18: {
polyglot.ConfigProto.CallConfiguration.Builder subBuilder = null;
if (callConfig_ != null) {
subBuilder = callConfig_.toBuilder();
}
callConfig_ = input.readMessage(polyglot.ConfigProto.CallConfiguration.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(callConfig_);
callConfig_ = subBuilder.buildPartial();
}
break;
}
case 26: {
polyglot.ConfigProto.ProtoConfiguration.Builder subBuilder = null;
if (protoConfig_ != null) {
subBuilder = protoConfig_.toBuilder();
}
protoConfig_ = input.readMessage(polyglot.ConfigProto.ProtoConfiguration.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(protoConfig_);
protoConfig_ = subBuilder.buildPartial();
}
break;
}
case 34: {
polyglot.ConfigProto.OutputConfiguration.Builder subBuilder = null;
if (outputConfig_ != null) {
subBuilder = outputConfig_.toBuilder();
}
outputConfig_ = input.readMessage(polyglot.ConfigProto.OutputConfiguration.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(outputConfig_);
outputConfig_ = subBuilder.buildPartial();
}
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return polyglot.ConfigProto.internal_static_polyglot_Configuration_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return polyglot.ConfigProto.internal_static_polyglot_Configuration_fieldAccessorTable
.ensureFieldAccessorsInitialized(
polyglot.ConfigProto.Configuration.class, polyglot.ConfigProto.Configuration.Builder.class);
}
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
* string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CALL_CONFIG_FIELD_NUMBER = 2;
private polyglot.ConfigProto.CallConfiguration callConfig_;
/**
* .polyglot.CallConfiguration call_config = 2;
* @return Whether the callConfig field is set.
*/
@java.lang.Override
public boolean hasCallConfig() {
return callConfig_ != null;
}
/**
* .polyglot.CallConfiguration call_config = 2;
* @return The callConfig.
*/
@java.lang.Override
public polyglot.ConfigProto.CallConfiguration getCallConfig() {
return callConfig_ == null ? polyglot.ConfigProto.CallConfiguration.getDefaultInstance() : callConfig_;
}
/**
* .polyglot.CallConfiguration call_config = 2;
*/
@java.lang.Override
public polyglot.ConfigProto.CallConfigurationOrBuilder getCallConfigOrBuilder() {
return getCallConfig();
}
public static final int PROTO_CONFIG_FIELD_NUMBER = 3;
private polyglot.ConfigProto.ProtoConfiguration protoConfig_;
/**
* .polyglot.ProtoConfiguration proto_config = 3;
* @return Whether the protoConfig field is set.
*/
@java.lang.Override
public boolean hasProtoConfig() {
return protoConfig_ != null;
}
/**
* .polyglot.ProtoConfiguration proto_config = 3;
* @return The protoConfig.
*/
@java.lang.Override
public polyglot.ConfigProto.ProtoConfiguration getProtoConfig() {
return protoConfig_ == null ? polyglot.ConfigProto.ProtoConfiguration.getDefaultInstance() : protoConfig_;
}
/**
* .polyglot.ProtoConfiguration proto_config = 3;
*/
@java.lang.Override
public polyglot.ConfigProto.ProtoConfigurationOrBuilder getProtoConfigOrBuilder() {
return getProtoConfig();
}
public static final int OUTPUT_CONFIG_FIELD_NUMBER = 4;
private polyglot.ConfigProto.OutputConfiguration outputConfig_;
/**
* .polyglot.OutputConfiguration output_config = 4;
* @return Whether the outputConfig field is set.
*/
@java.lang.Override
public boolean hasOutputConfig() {
return outputConfig_ != null;
}
/**
* .polyglot.OutputConfiguration output_config = 4;
* @return The outputConfig.
*/
@java.lang.Override
public polyglot.ConfigProto.OutputConfiguration getOutputConfig() {
return outputConfig_ == null ? polyglot.ConfigProto.OutputConfiguration.getDefaultInstance() : outputConfig_;
}
/**
* .polyglot.OutputConfiguration output_config = 4;
*/
@java.lang.Override
public polyglot.ConfigProto.OutputConfigurationOrBuilder getOutputConfigOrBuilder() {
return getOutputConfig();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (callConfig_ != null) {
output.writeMessage(2, getCallConfig());
}
if (protoConfig_ != null) {
output.writeMessage(3, getProtoConfig());
}
if (outputConfig_ != null) {
output.writeMessage(4, getOutputConfig());
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (callConfig_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getCallConfig());
}
if (protoConfig_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getProtoConfig());
}
if (outputConfig_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(4, getOutputConfig());
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof polyglot.ConfigProto.Configuration)) {
return super.equals(obj);
}
polyglot.ConfigProto.Configuration other = (polyglot.ConfigProto.Configuration) obj;
if (!getName()
.equals(other.getName())) return false;
if (hasCallConfig() != other.hasCallConfig()) return false;
if (hasCallConfig()) {
if (!getCallConfig()
.equals(other.getCallConfig())) return false;
}
if (hasProtoConfig() != other.hasProtoConfig()) return false;
if (hasProtoConfig()) {
if (!getProtoConfig()
.equals(other.getProtoConfig())) return false;
}
if (hasOutputConfig() != other.hasOutputConfig()) return false;
if (hasOutputConfig()) {
if (!getOutputConfig()
.equals(other.getOutputConfig())) return false;
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
if (hasCallConfig()) {
hash = (37 * hash) + CALL_CONFIG_FIELD_NUMBER;
hash = (53 * hash) + getCallConfig().hashCode();
}
if (hasProtoConfig()) {
hash = (37 * hash) + PROTO_CONFIG_FIELD_NUMBER;
hash = (53 * hash) + getProtoConfig().hashCode();
}
if (hasOutputConfig()) {
hash = (37 * hash) + OUTPUT_CONFIG_FIELD_NUMBER;
hash = (53 * hash) + getOutputConfig().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static polyglot.ConfigProto.Configuration parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.Configuration parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.Configuration parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.Configuration parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.Configuration parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.Configuration parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.Configuration parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.Configuration parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static polyglot.ConfigProto.Configuration parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.Configuration parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static polyglot.ConfigProto.Configuration parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.Configuration parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(polyglot.ConfigProto.Configuration prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Holds parameters used by Polylgot at runtime.
*
*
* Protobuf type {@code polyglot.Configuration}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:polyglot.Configuration)
polyglot.ConfigProto.ConfigurationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return polyglot.ConfigProto.internal_static_polyglot_Configuration_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return polyglot.ConfigProto.internal_static_polyglot_Configuration_fieldAccessorTable
.ensureFieldAccessorsInitialized(
polyglot.ConfigProto.Configuration.class, polyglot.ConfigProto.Configuration.Builder.class);
}
// Construct using polyglot.ConfigProto.Configuration.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
name_ = "";
if (callConfigBuilder_ == null) {
callConfig_ = null;
} else {
callConfig_ = null;
callConfigBuilder_ = null;
}
if (protoConfigBuilder_ == null) {
protoConfig_ = null;
} else {
protoConfig_ = null;
protoConfigBuilder_ = null;
}
if (outputConfigBuilder_ == null) {
outputConfig_ = null;
} else {
outputConfig_ = null;
outputConfigBuilder_ = null;
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return polyglot.ConfigProto.internal_static_polyglot_Configuration_descriptor;
}
@java.lang.Override
public polyglot.ConfigProto.Configuration getDefaultInstanceForType() {
return polyglot.ConfigProto.Configuration.getDefaultInstance();
}
@java.lang.Override
public polyglot.ConfigProto.Configuration build() {
polyglot.ConfigProto.Configuration result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public polyglot.ConfigProto.Configuration buildPartial() {
polyglot.ConfigProto.Configuration result = new polyglot.ConfigProto.Configuration(this);
result.name_ = name_;
if (callConfigBuilder_ == null) {
result.callConfig_ = callConfig_;
} else {
result.callConfig_ = callConfigBuilder_.build();
}
if (protoConfigBuilder_ == null) {
result.protoConfig_ = protoConfig_;
} else {
result.protoConfig_ = protoConfigBuilder_.build();
}
if (outputConfigBuilder_ == null) {
result.outputConfig_ = outputConfig_;
} else {
result.outputConfig_ = outputConfigBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof polyglot.ConfigProto.Configuration) {
return mergeFrom((polyglot.ConfigProto.Configuration)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(polyglot.ConfigProto.Configuration other) {
if (other == polyglot.ConfigProto.Configuration.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (other.hasCallConfig()) {
mergeCallConfig(other.getCallConfig());
}
if (other.hasProtoConfig()) {
mergeProtoConfig(other.getProtoConfig());
}
if (other.hasOutputConfig()) {
mergeOutputConfig(other.getOutputConfig());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
polyglot.ConfigProto.Configuration parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (polyglot.ConfigProto.Configuration) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object name_ = "";
/**
* string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
* string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private polyglot.ConfigProto.CallConfiguration callConfig_;
private com.google.protobuf.SingleFieldBuilderV3<
polyglot.ConfigProto.CallConfiguration, polyglot.ConfigProto.CallConfiguration.Builder, polyglot.ConfigProto.CallConfigurationOrBuilder> callConfigBuilder_;
/**
* .polyglot.CallConfiguration call_config = 2;
* @return Whether the callConfig field is set.
*/
public boolean hasCallConfig() {
return callConfigBuilder_ != null || callConfig_ != null;
}
/**
* .polyglot.CallConfiguration call_config = 2;
* @return The callConfig.
*/
public polyglot.ConfigProto.CallConfiguration getCallConfig() {
if (callConfigBuilder_ == null) {
return callConfig_ == null ? polyglot.ConfigProto.CallConfiguration.getDefaultInstance() : callConfig_;
} else {
return callConfigBuilder_.getMessage();
}
}
/**
* .polyglot.CallConfiguration call_config = 2;
*/
public Builder setCallConfig(polyglot.ConfigProto.CallConfiguration value) {
if (callConfigBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
callConfig_ = value;
onChanged();
} else {
callConfigBuilder_.setMessage(value);
}
return this;
}
/**
* .polyglot.CallConfiguration call_config = 2;
*/
public Builder setCallConfig(
polyglot.ConfigProto.CallConfiguration.Builder builderForValue) {
if (callConfigBuilder_ == null) {
callConfig_ = builderForValue.build();
onChanged();
} else {
callConfigBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .polyglot.CallConfiguration call_config = 2;
*/
public Builder mergeCallConfig(polyglot.ConfigProto.CallConfiguration value) {
if (callConfigBuilder_ == null) {
if (callConfig_ != null) {
callConfig_ =
polyglot.ConfigProto.CallConfiguration.newBuilder(callConfig_).mergeFrom(value).buildPartial();
} else {
callConfig_ = value;
}
onChanged();
} else {
callConfigBuilder_.mergeFrom(value);
}
return this;
}
/**
* .polyglot.CallConfiguration call_config = 2;
*/
public Builder clearCallConfig() {
if (callConfigBuilder_ == null) {
callConfig_ = null;
onChanged();
} else {
callConfig_ = null;
callConfigBuilder_ = null;
}
return this;
}
/**
* .polyglot.CallConfiguration call_config = 2;
*/
public polyglot.ConfigProto.CallConfiguration.Builder getCallConfigBuilder() {
onChanged();
return getCallConfigFieldBuilder().getBuilder();
}
/**
* .polyglot.CallConfiguration call_config = 2;
*/
public polyglot.ConfigProto.CallConfigurationOrBuilder getCallConfigOrBuilder() {
if (callConfigBuilder_ != null) {
return callConfigBuilder_.getMessageOrBuilder();
} else {
return callConfig_ == null ?
polyglot.ConfigProto.CallConfiguration.getDefaultInstance() : callConfig_;
}
}
/**
* .polyglot.CallConfiguration call_config = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
polyglot.ConfigProto.CallConfiguration, polyglot.ConfigProto.CallConfiguration.Builder, polyglot.ConfigProto.CallConfigurationOrBuilder>
getCallConfigFieldBuilder() {
if (callConfigBuilder_ == null) {
callConfigBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
polyglot.ConfigProto.CallConfiguration, polyglot.ConfigProto.CallConfiguration.Builder, polyglot.ConfigProto.CallConfigurationOrBuilder>(
getCallConfig(),
getParentForChildren(),
isClean());
callConfig_ = null;
}
return callConfigBuilder_;
}
private polyglot.ConfigProto.ProtoConfiguration protoConfig_;
private com.google.protobuf.SingleFieldBuilderV3<
polyglot.ConfigProto.ProtoConfiguration, polyglot.ConfigProto.ProtoConfiguration.Builder, polyglot.ConfigProto.ProtoConfigurationOrBuilder> protoConfigBuilder_;
/**
* .polyglot.ProtoConfiguration proto_config = 3;
* @return Whether the protoConfig field is set.
*/
public boolean hasProtoConfig() {
return protoConfigBuilder_ != null || protoConfig_ != null;
}
/**
* .polyglot.ProtoConfiguration proto_config = 3;
* @return The protoConfig.
*/
public polyglot.ConfigProto.ProtoConfiguration getProtoConfig() {
if (protoConfigBuilder_ == null) {
return protoConfig_ == null ? polyglot.ConfigProto.ProtoConfiguration.getDefaultInstance() : protoConfig_;
} else {
return protoConfigBuilder_.getMessage();
}
}
/**
* .polyglot.ProtoConfiguration proto_config = 3;
*/
public Builder setProtoConfig(polyglot.ConfigProto.ProtoConfiguration value) {
if (protoConfigBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
protoConfig_ = value;
onChanged();
} else {
protoConfigBuilder_.setMessage(value);
}
return this;
}
/**
* .polyglot.ProtoConfiguration proto_config = 3;
*/
public Builder setProtoConfig(
polyglot.ConfigProto.ProtoConfiguration.Builder builderForValue) {
if (protoConfigBuilder_ == null) {
protoConfig_ = builderForValue.build();
onChanged();
} else {
protoConfigBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .polyglot.ProtoConfiguration proto_config = 3;
*/
public Builder mergeProtoConfig(polyglot.ConfigProto.ProtoConfiguration value) {
if (protoConfigBuilder_ == null) {
if (protoConfig_ != null) {
protoConfig_ =
polyglot.ConfigProto.ProtoConfiguration.newBuilder(protoConfig_).mergeFrom(value).buildPartial();
} else {
protoConfig_ = value;
}
onChanged();
} else {
protoConfigBuilder_.mergeFrom(value);
}
return this;
}
/**
* .polyglot.ProtoConfiguration proto_config = 3;
*/
public Builder clearProtoConfig() {
if (protoConfigBuilder_ == null) {
protoConfig_ = null;
onChanged();
} else {
protoConfig_ = null;
protoConfigBuilder_ = null;
}
return this;
}
/**
* .polyglot.ProtoConfiguration proto_config = 3;
*/
public polyglot.ConfigProto.ProtoConfiguration.Builder getProtoConfigBuilder() {
onChanged();
return getProtoConfigFieldBuilder().getBuilder();
}
/**
* .polyglot.ProtoConfiguration proto_config = 3;
*/
public polyglot.ConfigProto.ProtoConfigurationOrBuilder getProtoConfigOrBuilder() {
if (protoConfigBuilder_ != null) {
return protoConfigBuilder_.getMessageOrBuilder();
} else {
return protoConfig_ == null ?
polyglot.ConfigProto.ProtoConfiguration.getDefaultInstance() : protoConfig_;
}
}
/**
* .polyglot.ProtoConfiguration proto_config = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
polyglot.ConfigProto.ProtoConfiguration, polyglot.ConfigProto.ProtoConfiguration.Builder, polyglot.ConfigProto.ProtoConfigurationOrBuilder>
getProtoConfigFieldBuilder() {
if (protoConfigBuilder_ == null) {
protoConfigBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
polyglot.ConfigProto.ProtoConfiguration, polyglot.ConfigProto.ProtoConfiguration.Builder, polyglot.ConfigProto.ProtoConfigurationOrBuilder>(
getProtoConfig(),
getParentForChildren(),
isClean());
protoConfig_ = null;
}
return protoConfigBuilder_;
}
private polyglot.ConfigProto.OutputConfiguration outputConfig_;
private com.google.protobuf.SingleFieldBuilderV3<
polyglot.ConfigProto.OutputConfiguration, polyglot.ConfigProto.OutputConfiguration.Builder, polyglot.ConfigProto.OutputConfigurationOrBuilder> outputConfigBuilder_;
/**
* .polyglot.OutputConfiguration output_config = 4;
* @return Whether the outputConfig field is set.
*/
public boolean hasOutputConfig() {
return outputConfigBuilder_ != null || outputConfig_ != null;
}
/**
* .polyglot.OutputConfiguration output_config = 4;
* @return The outputConfig.
*/
public polyglot.ConfigProto.OutputConfiguration getOutputConfig() {
if (outputConfigBuilder_ == null) {
return outputConfig_ == null ? polyglot.ConfigProto.OutputConfiguration.getDefaultInstance() : outputConfig_;
} else {
return outputConfigBuilder_.getMessage();
}
}
/**
* .polyglot.OutputConfiguration output_config = 4;
*/
public Builder setOutputConfig(polyglot.ConfigProto.OutputConfiguration value) {
if (outputConfigBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
outputConfig_ = value;
onChanged();
} else {
outputConfigBuilder_.setMessage(value);
}
return this;
}
/**
* .polyglot.OutputConfiguration output_config = 4;
*/
public Builder setOutputConfig(
polyglot.ConfigProto.OutputConfiguration.Builder builderForValue) {
if (outputConfigBuilder_ == null) {
outputConfig_ = builderForValue.build();
onChanged();
} else {
outputConfigBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .polyglot.OutputConfiguration output_config = 4;
*/
public Builder mergeOutputConfig(polyglot.ConfigProto.OutputConfiguration value) {
if (outputConfigBuilder_ == null) {
if (outputConfig_ != null) {
outputConfig_ =
polyglot.ConfigProto.OutputConfiguration.newBuilder(outputConfig_).mergeFrom(value).buildPartial();
} else {
outputConfig_ = value;
}
onChanged();
} else {
outputConfigBuilder_.mergeFrom(value);
}
return this;
}
/**
* .polyglot.OutputConfiguration output_config = 4;
*/
public Builder clearOutputConfig() {
if (outputConfigBuilder_ == null) {
outputConfig_ = null;
onChanged();
} else {
outputConfig_ = null;
outputConfigBuilder_ = null;
}
return this;
}
/**
* .polyglot.OutputConfiguration output_config = 4;
*/
public polyglot.ConfigProto.OutputConfiguration.Builder getOutputConfigBuilder() {
onChanged();
return getOutputConfigFieldBuilder().getBuilder();
}
/**
* .polyglot.OutputConfiguration output_config = 4;
*/
public polyglot.ConfigProto.OutputConfigurationOrBuilder getOutputConfigOrBuilder() {
if (outputConfigBuilder_ != null) {
return outputConfigBuilder_.getMessageOrBuilder();
} else {
return outputConfig_ == null ?
polyglot.ConfigProto.OutputConfiguration.getDefaultInstance() : outputConfig_;
}
}
/**
* .polyglot.OutputConfiguration output_config = 4;
*/
private com.google.protobuf.SingleFieldBuilderV3<
polyglot.ConfigProto.OutputConfiguration, polyglot.ConfigProto.OutputConfiguration.Builder, polyglot.ConfigProto.OutputConfigurationOrBuilder>
getOutputConfigFieldBuilder() {
if (outputConfigBuilder_ == null) {
outputConfigBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
polyglot.ConfigProto.OutputConfiguration, polyglot.ConfigProto.OutputConfiguration.Builder, polyglot.ConfigProto.OutputConfigurationOrBuilder>(
getOutputConfig(),
getParentForChildren(),
isClean());
outputConfig_ = null;
}
return outputConfigBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:polyglot.Configuration)
}
// @@protoc_insertion_point(class_scope:polyglot.Configuration)
private static final polyglot.ConfigProto.Configuration DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new polyglot.ConfigProto.Configuration();
}
public static polyglot.ConfigProto.Configuration getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Configuration parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new Configuration(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public polyglot.ConfigProto.Configuration getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CallConfigurationOrBuilder extends
// @@protoc_insertion_point(interface_extends:polyglot.CallConfiguration)
com.google.protobuf.MessageOrBuilder {
/**
* uint32 deadline_ms = 1;
* @return The deadlineMs.
*/
int getDeadlineMs();
/**
* bool use_tls = 2;
* @return The useTls.
*/
boolean getUseTls();
/**
* .polyglot.OauthConfiguration oauth_config = 3;
* @return Whether the oauthConfig field is set.
*/
boolean hasOauthConfig();
/**
* .polyglot.OauthConfiguration oauth_config = 3;
* @return The oauthConfig.
*/
polyglot.ConfigProto.OauthConfiguration getOauthConfig();
/**
* .polyglot.OauthConfiguration oauth_config = 3;
*/
polyglot.ConfigProto.OauthConfigurationOrBuilder getOauthConfigOrBuilder();
/**
*
* If set, this file will be used as a root certificate for calls using TLS.
*
*
* string tls_ca_cert_path = 4;
* @return The tlsCaCertPath.
*/
java.lang.String getTlsCaCertPath();
/**
*
* If set, this file will be used as a root certificate for calls using TLS.
*
*
* string tls_ca_cert_path = 4;
* @return The bytes for tlsCaCertPath.
*/
com.google.protobuf.ByteString
getTlsCaCertPathBytes();
/**
*
* If set, this will use client certs for authentication
*
*
* string tls_client_cert_path = 5;
* @return The tlsClientCertPath.
*/
java.lang.String getTlsClientCertPath();
/**
*
* If set, this will use client certs for authentication
*
*
* string tls_client_cert_path = 5;
* @return The bytes for tlsClientCertPath.
*/
com.google.protobuf.ByteString
getTlsClientCertPathBytes();
/**
* string tls_client_key_path = 6;
* @return The tlsClientKeyPath.
*/
java.lang.String getTlsClientKeyPath();
/**
* string tls_client_key_path = 6;
* @return The bytes for tlsClientKeyPath.
*/
com.google.protobuf.ByteString
getTlsClientKeyPathBytes();
/**
* string tls_client_override_authority = 7;
* @return The tlsClientOverrideAuthority.
*/
java.lang.String getTlsClientOverrideAuthority();
/**
* string tls_client_override_authority = 7;
* @return The bytes for tlsClientOverrideAuthority.
*/
com.google.protobuf.ByteString
getTlsClientOverrideAuthorityBytes();
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
java.util.List
getMetadataList();
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
polyglot.ConfigProto.CallMetadataEntry getMetadata(int index);
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
int getMetadataCount();
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
java.util.List extends polyglot.ConfigProto.CallMetadataEntryOrBuilder>
getMetadataOrBuilderList();
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
polyglot.ConfigProto.CallMetadataEntryOrBuilder getMetadataOrBuilder(
int index);
}
/**
*
* Holds parameters used to make rpc calls.
*
*
* Protobuf type {@code polyglot.CallConfiguration}
*/
public static final class CallConfiguration extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:polyglot.CallConfiguration)
CallConfigurationOrBuilder {
private static final long serialVersionUID = 0L;
// Use CallConfiguration.newBuilder() to construct.
private CallConfiguration(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CallConfiguration() {
tlsCaCertPath_ = "";
tlsClientCertPath_ = "";
tlsClientKeyPath_ = "";
tlsClientOverrideAuthority_ = "";
metadata_ = java.util.Collections.emptyList();
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CallConfiguration();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CallConfiguration(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
deadlineMs_ = input.readUInt32();
break;
}
case 16: {
useTls_ = input.readBool();
break;
}
case 26: {
polyglot.ConfigProto.OauthConfiguration.Builder subBuilder = null;
if (oauthConfig_ != null) {
subBuilder = oauthConfig_.toBuilder();
}
oauthConfig_ = input.readMessage(polyglot.ConfigProto.OauthConfiguration.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(oauthConfig_);
oauthConfig_ = subBuilder.buildPartial();
}
break;
}
case 34: {
java.lang.String s = input.readStringRequireUtf8();
tlsCaCertPath_ = s;
break;
}
case 42: {
java.lang.String s = input.readStringRequireUtf8();
tlsClientCertPath_ = s;
break;
}
case 50: {
java.lang.String s = input.readStringRequireUtf8();
tlsClientKeyPath_ = s;
break;
}
case 58: {
java.lang.String s = input.readStringRequireUtf8();
tlsClientOverrideAuthority_ = s;
break;
}
case 66: {
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
metadata_ = new java.util.ArrayList();
mutable_bitField0_ |= 0x00000001;
}
metadata_.add(
input.readMessage(polyglot.ConfigProto.CallMetadataEntry.parser(), extensionRegistry));
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
metadata_ = java.util.Collections.unmodifiableList(metadata_);
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return polyglot.ConfigProto.internal_static_polyglot_CallConfiguration_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return polyglot.ConfigProto.internal_static_polyglot_CallConfiguration_fieldAccessorTable
.ensureFieldAccessorsInitialized(
polyglot.ConfigProto.CallConfiguration.class, polyglot.ConfigProto.CallConfiguration.Builder.class);
}
public static final int DEADLINE_MS_FIELD_NUMBER = 1;
private int deadlineMs_;
/**
* uint32 deadline_ms = 1;
* @return The deadlineMs.
*/
@java.lang.Override
public int getDeadlineMs() {
return deadlineMs_;
}
public static final int USE_TLS_FIELD_NUMBER = 2;
private boolean useTls_;
/**
* bool use_tls = 2;
* @return The useTls.
*/
@java.lang.Override
public boolean getUseTls() {
return useTls_;
}
public static final int OAUTH_CONFIG_FIELD_NUMBER = 3;
private polyglot.ConfigProto.OauthConfiguration oauthConfig_;
/**
* .polyglot.OauthConfiguration oauth_config = 3;
* @return Whether the oauthConfig field is set.
*/
@java.lang.Override
public boolean hasOauthConfig() {
return oauthConfig_ != null;
}
/**
* .polyglot.OauthConfiguration oauth_config = 3;
* @return The oauthConfig.
*/
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration getOauthConfig() {
return oauthConfig_ == null ? polyglot.ConfigProto.OauthConfiguration.getDefaultInstance() : oauthConfig_;
}
/**
* .polyglot.OauthConfiguration oauth_config = 3;
*/
@java.lang.Override
public polyglot.ConfigProto.OauthConfigurationOrBuilder getOauthConfigOrBuilder() {
return getOauthConfig();
}
public static final int TLS_CA_CERT_PATH_FIELD_NUMBER = 4;
private volatile java.lang.Object tlsCaCertPath_;
/**
*
* If set, this file will be used as a root certificate for calls using TLS.
*
*
* string tls_ca_cert_path = 4;
* @return The tlsCaCertPath.
*/
@java.lang.Override
public java.lang.String getTlsCaCertPath() {
java.lang.Object ref = tlsCaCertPath_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tlsCaCertPath_ = s;
return s;
}
}
/**
*
* If set, this file will be used as a root certificate for calls using TLS.
*
*
* string tls_ca_cert_path = 4;
* @return The bytes for tlsCaCertPath.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTlsCaCertPathBytes() {
java.lang.Object ref = tlsCaCertPath_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tlsCaCertPath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TLS_CLIENT_CERT_PATH_FIELD_NUMBER = 5;
private volatile java.lang.Object tlsClientCertPath_;
/**
*
* If set, this will use client certs for authentication
*
*
* string tls_client_cert_path = 5;
* @return The tlsClientCertPath.
*/
@java.lang.Override
public java.lang.String getTlsClientCertPath() {
java.lang.Object ref = tlsClientCertPath_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tlsClientCertPath_ = s;
return s;
}
}
/**
*
* If set, this will use client certs for authentication
*
*
* string tls_client_cert_path = 5;
* @return The bytes for tlsClientCertPath.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTlsClientCertPathBytes() {
java.lang.Object ref = tlsClientCertPath_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tlsClientCertPath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TLS_CLIENT_KEY_PATH_FIELD_NUMBER = 6;
private volatile java.lang.Object tlsClientKeyPath_;
/**
* string tls_client_key_path = 6;
* @return The tlsClientKeyPath.
*/
@java.lang.Override
public java.lang.String getTlsClientKeyPath() {
java.lang.Object ref = tlsClientKeyPath_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tlsClientKeyPath_ = s;
return s;
}
}
/**
* string tls_client_key_path = 6;
* @return The bytes for tlsClientKeyPath.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTlsClientKeyPathBytes() {
java.lang.Object ref = tlsClientKeyPath_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tlsClientKeyPath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TLS_CLIENT_OVERRIDE_AUTHORITY_FIELD_NUMBER = 7;
private volatile java.lang.Object tlsClientOverrideAuthority_;
/**
* string tls_client_override_authority = 7;
* @return The tlsClientOverrideAuthority.
*/
@java.lang.Override
public java.lang.String getTlsClientOverrideAuthority() {
java.lang.Object ref = tlsClientOverrideAuthority_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tlsClientOverrideAuthority_ = s;
return s;
}
}
/**
* string tls_client_override_authority = 7;
* @return The bytes for tlsClientOverrideAuthority.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTlsClientOverrideAuthorityBytes() {
java.lang.Object ref = tlsClientOverrideAuthority_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tlsClientOverrideAuthority_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int METADATA_FIELD_NUMBER = 8;
private java.util.List metadata_;
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
@java.lang.Override
public java.util.List getMetadataList() {
return metadata_;
}
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
@java.lang.Override
public java.util.List extends polyglot.ConfigProto.CallMetadataEntryOrBuilder>
getMetadataOrBuilderList() {
return metadata_;
}
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
@java.lang.Override
public int getMetadataCount() {
return metadata_.size();
}
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
@java.lang.Override
public polyglot.ConfigProto.CallMetadataEntry getMetadata(int index) {
return metadata_.get(index);
}
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
@java.lang.Override
public polyglot.ConfigProto.CallMetadataEntryOrBuilder getMetadataOrBuilder(
int index) {
return metadata_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (deadlineMs_ != 0) {
output.writeUInt32(1, deadlineMs_);
}
if (useTls_ != false) {
output.writeBool(2, useTls_);
}
if (oauthConfig_ != null) {
output.writeMessage(3, getOauthConfig());
}
if (!getTlsCaCertPathBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 4, tlsCaCertPath_);
}
if (!getTlsClientCertPathBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 5, tlsClientCertPath_);
}
if (!getTlsClientKeyPathBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 6, tlsClientKeyPath_);
}
if (!getTlsClientOverrideAuthorityBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 7, tlsClientOverrideAuthority_);
}
for (int i = 0; i < metadata_.size(); i++) {
output.writeMessage(8, metadata_.get(i));
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (deadlineMs_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeUInt32Size(1, deadlineMs_);
}
if (useTls_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(2, useTls_);
}
if (oauthConfig_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(3, getOauthConfig());
}
if (!getTlsCaCertPathBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(4, tlsCaCertPath_);
}
if (!getTlsClientCertPathBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(5, tlsClientCertPath_);
}
if (!getTlsClientKeyPathBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(6, tlsClientKeyPath_);
}
if (!getTlsClientOverrideAuthorityBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(7, tlsClientOverrideAuthority_);
}
for (int i = 0; i < metadata_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, metadata_.get(i));
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof polyglot.ConfigProto.CallConfiguration)) {
return super.equals(obj);
}
polyglot.ConfigProto.CallConfiguration other = (polyglot.ConfigProto.CallConfiguration) obj;
if (getDeadlineMs()
!= other.getDeadlineMs()) return false;
if (getUseTls()
!= other.getUseTls()) return false;
if (hasOauthConfig() != other.hasOauthConfig()) return false;
if (hasOauthConfig()) {
if (!getOauthConfig()
.equals(other.getOauthConfig())) return false;
}
if (!getTlsCaCertPath()
.equals(other.getTlsCaCertPath())) return false;
if (!getTlsClientCertPath()
.equals(other.getTlsClientCertPath())) return false;
if (!getTlsClientKeyPath()
.equals(other.getTlsClientKeyPath())) return false;
if (!getTlsClientOverrideAuthority()
.equals(other.getTlsClientOverrideAuthority())) return false;
if (!getMetadataList()
.equals(other.getMetadataList())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + DEADLINE_MS_FIELD_NUMBER;
hash = (53 * hash) + getDeadlineMs();
hash = (37 * hash) + USE_TLS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseTls());
if (hasOauthConfig()) {
hash = (37 * hash) + OAUTH_CONFIG_FIELD_NUMBER;
hash = (53 * hash) + getOauthConfig().hashCode();
}
hash = (37 * hash) + TLS_CA_CERT_PATH_FIELD_NUMBER;
hash = (53 * hash) + getTlsCaCertPath().hashCode();
hash = (37 * hash) + TLS_CLIENT_CERT_PATH_FIELD_NUMBER;
hash = (53 * hash) + getTlsClientCertPath().hashCode();
hash = (37 * hash) + TLS_CLIENT_KEY_PATH_FIELD_NUMBER;
hash = (53 * hash) + getTlsClientKeyPath().hashCode();
hash = (37 * hash) + TLS_CLIENT_OVERRIDE_AUTHORITY_FIELD_NUMBER;
hash = (53 * hash) + getTlsClientOverrideAuthority().hashCode();
if (getMetadataCount() > 0) {
hash = (37 * hash) + METADATA_FIELD_NUMBER;
hash = (53 * hash) + getMetadataList().hashCode();
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static polyglot.ConfigProto.CallConfiguration parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.CallConfiguration parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.CallConfiguration parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.CallConfiguration parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.CallConfiguration parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.CallConfiguration parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.CallConfiguration parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.CallConfiguration parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static polyglot.ConfigProto.CallConfiguration parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.CallConfiguration parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static polyglot.ConfigProto.CallConfiguration parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.CallConfiguration parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(polyglot.ConfigProto.CallConfiguration prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Holds parameters used to make rpc calls.
*
*
* Protobuf type {@code polyglot.CallConfiguration}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:polyglot.CallConfiguration)
polyglot.ConfigProto.CallConfigurationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return polyglot.ConfigProto.internal_static_polyglot_CallConfiguration_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return polyglot.ConfigProto.internal_static_polyglot_CallConfiguration_fieldAccessorTable
.ensureFieldAccessorsInitialized(
polyglot.ConfigProto.CallConfiguration.class, polyglot.ConfigProto.CallConfiguration.Builder.class);
}
// Construct using polyglot.ConfigProto.CallConfiguration.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
getMetadataFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
deadlineMs_ = 0;
useTls_ = false;
if (oauthConfigBuilder_ == null) {
oauthConfig_ = null;
} else {
oauthConfig_ = null;
oauthConfigBuilder_ = null;
}
tlsCaCertPath_ = "";
tlsClientCertPath_ = "";
tlsClientKeyPath_ = "";
tlsClientOverrideAuthority_ = "";
if (metadataBuilder_ == null) {
metadata_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
} else {
metadataBuilder_.clear();
}
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return polyglot.ConfigProto.internal_static_polyglot_CallConfiguration_descriptor;
}
@java.lang.Override
public polyglot.ConfigProto.CallConfiguration getDefaultInstanceForType() {
return polyglot.ConfigProto.CallConfiguration.getDefaultInstance();
}
@java.lang.Override
public polyglot.ConfigProto.CallConfiguration build() {
polyglot.ConfigProto.CallConfiguration result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public polyglot.ConfigProto.CallConfiguration buildPartial() {
polyglot.ConfigProto.CallConfiguration result = new polyglot.ConfigProto.CallConfiguration(this);
int from_bitField0_ = bitField0_;
result.deadlineMs_ = deadlineMs_;
result.useTls_ = useTls_;
if (oauthConfigBuilder_ == null) {
result.oauthConfig_ = oauthConfig_;
} else {
result.oauthConfig_ = oauthConfigBuilder_.build();
}
result.tlsCaCertPath_ = tlsCaCertPath_;
result.tlsClientCertPath_ = tlsClientCertPath_;
result.tlsClientKeyPath_ = tlsClientKeyPath_;
result.tlsClientOverrideAuthority_ = tlsClientOverrideAuthority_;
if (metadataBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
metadata_ = java.util.Collections.unmodifiableList(metadata_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.metadata_ = metadata_;
} else {
result.metadata_ = metadataBuilder_.build();
}
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof polyglot.ConfigProto.CallConfiguration) {
return mergeFrom((polyglot.ConfigProto.CallConfiguration)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(polyglot.ConfigProto.CallConfiguration other) {
if (other == polyglot.ConfigProto.CallConfiguration.getDefaultInstance()) return this;
if (other.getDeadlineMs() != 0) {
setDeadlineMs(other.getDeadlineMs());
}
if (other.getUseTls() != false) {
setUseTls(other.getUseTls());
}
if (other.hasOauthConfig()) {
mergeOauthConfig(other.getOauthConfig());
}
if (!other.getTlsCaCertPath().isEmpty()) {
tlsCaCertPath_ = other.tlsCaCertPath_;
onChanged();
}
if (!other.getTlsClientCertPath().isEmpty()) {
tlsClientCertPath_ = other.tlsClientCertPath_;
onChanged();
}
if (!other.getTlsClientKeyPath().isEmpty()) {
tlsClientKeyPath_ = other.tlsClientKeyPath_;
onChanged();
}
if (!other.getTlsClientOverrideAuthority().isEmpty()) {
tlsClientOverrideAuthority_ = other.tlsClientOverrideAuthority_;
onChanged();
}
if (metadataBuilder_ == null) {
if (!other.metadata_.isEmpty()) {
if (metadata_.isEmpty()) {
metadata_ = other.metadata_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureMetadataIsMutable();
metadata_.addAll(other.metadata_);
}
onChanged();
}
} else {
if (!other.metadata_.isEmpty()) {
if (metadataBuilder_.isEmpty()) {
metadataBuilder_.dispose();
metadataBuilder_ = null;
metadata_ = other.metadata_;
bitField0_ = (bitField0_ & ~0x00000001);
metadataBuilder_ =
com.google.protobuf.GeneratedMessageV3.alwaysUseFieldBuilders ?
getMetadataFieldBuilder() : null;
} else {
metadataBuilder_.addAllMessages(other.metadata_);
}
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
polyglot.ConfigProto.CallConfiguration parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (polyglot.ConfigProto.CallConfiguration) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private int deadlineMs_ ;
/**
* uint32 deadline_ms = 1;
* @return The deadlineMs.
*/
@java.lang.Override
public int getDeadlineMs() {
return deadlineMs_;
}
/**
* uint32 deadline_ms = 1;
* @param value The deadlineMs to set.
* @return This builder for chaining.
*/
public Builder setDeadlineMs(int value) {
deadlineMs_ = value;
onChanged();
return this;
}
/**
* uint32 deadline_ms = 1;
* @return This builder for chaining.
*/
public Builder clearDeadlineMs() {
deadlineMs_ = 0;
onChanged();
return this;
}
private boolean useTls_ ;
/**
* bool use_tls = 2;
* @return The useTls.
*/
@java.lang.Override
public boolean getUseTls() {
return useTls_;
}
/**
* bool use_tls = 2;
* @param value The useTls to set.
* @return This builder for chaining.
*/
public Builder setUseTls(boolean value) {
useTls_ = value;
onChanged();
return this;
}
/**
* bool use_tls = 2;
* @return This builder for chaining.
*/
public Builder clearUseTls() {
useTls_ = false;
onChanged();
return this;
}
private polyglot.ConfigProto.OauthConfiguration oauthConfig_;
private com.google.protobuf.SingleFieldBuilderV3<
polyglot.ConfigProto.OauthConfiguration, polyglot.ConfigProto.OauthConfiguration.Builder, polyglot.ConfigProto.OauthConfigurationOrBuilder> oauthConfigBuilder_;
/**
* .polyglot.OauthConfiguration oauth_config = 3;
* @return Whether the oauthConfig field is set.
*/
public boolean hasOauthConfig() {
return oauthConfigBuilder_ != null || oauthConfig_ != null;
}
/**
* .polyglot.OauthConfiguration oauth_config = 3;
* @return The oauthConfig.
*/
public polyglot.ConfigProto.OauthConfiguration getOauthConfig() {
if (oauthConfigBuilder_ == null) {
return oauthConfig_ == null ? polyglot.ConfigProto.OauthConfiguration.getDefaultInstance() : oauthConfig_;
} else {
return oauthConfigBuilder_.getMessage();
}
}
/**
* .polyglot.OauthConfiguration oauth_config = 3;
*/
public Builder setOauthConfig(polyglot.ConfigProto.OauthConfiguration value) {
if (oauthConfigBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
oauthConfig_ = value;
onChanged();
} else {
oauthConfigBuilder_.setMessage(value);
}
return this;
}
/**
* .polyglot.OauthConfiguration oauth_config = 3;
*/
public Builder setOauthConfig(
polyglot.ConfigProto.OauthConfiguration.Builder builderForValue) {
if (oauthConfigBuilder_ == null) {
oauthConfig_ = builderForValue.build();
onChanged();
} else {
oauthConfigBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
* .polyglot.OauthConfiguration oauth_config = 3;
*/
public Builder mergeOauthConfig(polyglot.ConfigProto.OauthConfiguration value) {
if (oauthConfigBuilder_ == null) {
if (oauthConfig_ != null) {
oauthConfig_ =
polyglot.ConfigProto.OauthConfiguration.newBuilder(oauthConfig_).mergeFrom(value).buildPartial();
} else {
oauthConfig_ = value;
}
onChanged();
} else {
oauthConfigBuilder_.mergeFrom(value);
}
return this;
}
/**
* .polyglot.OauthConfiguration oauth_config = 3;
*/
public Builder clearOauthConfig() {
if (oauthConfigBuilder_ == null) {
oauthConfig_ = null;
onChanged();
} else {
oauthConfig_ = null;
oauthConfigBuilder_ = null;
}
return this;
}
/**
* .polyglot.OauthConfiguration oauth_config = 3;
*/
public polyglot.ConfigProto.OauthConfiguration.Builder getOauthConfigBuilder() {
onChanged();
return getOauthConfigFieldBuilder().getBuilder();
}
/**
* .polyglot.OauthConfiguration oauth_config = 3;
*/
public polyglot.ConfigProto.OauthConfigurationOrBuilder getOauthConfigOrBuilder() {
if (oauthConfigBuilder_ != null) {
return oauthConfigBuilder_.getMessageOrBuilder();
} else {
return oauthConfig_ == null ?
polyglot.ConfigProto.OauthConfiguration.getDefaultInstance() : oauthConfig_;
}
}
/**
* .polyglot.OauthConfiguration oauth_config = 3;
*/
private com.google.protobuf.SingleFieldBuilderV3<
polyglot.ConfigProto.OauthConfiguration, polyglot.ConfigProto.OauthConfiguration.Builder, polyglot.ConfigProto.OauthConfigurationOrBuilder>
getOauthConfigFieldBuilder() {
if (oauthConfigBuilder_ == null) {
oauthConfigBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
polyglot.ConfigProto.OauthConfiguration, polyglot.ConfigProto.OauthConfiguration.Builder, polyglot.ConfigProto.OauthConfigurationOrBuilder>(
getOauthConfig(),
getParentForChildren(),
isClean());
oauthConfig_ = null;
}
return oauthConfigBuilder_;
}
private java.lang.Object tlsCaCertPath_ = "";
/**
*
* If set, this file will be used as a root certificate for calls using TLS.
*
*
* string tls_ca_cert_path = 4;
* @return The tlsCaCertPath.
*/
public java.lang.String getTlsCaCertPath() {
java.lang.Object ref = tlsCaCertPath_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tlsCaCertPath_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* If set, this file will be used as a root certificate for calls using TLS.
*
*
* string tls_ca_cert_path = 4;
* @return The bytes for tlsCaCertPath.
*/
public com.google.protobuf.ByteString
getTlsCaCertPathBytes() {
java.lang.Object ref = tlsCaCertPath_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tlsCaCertPath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* If set, this file will be used as a root certificate for calls using TLS.
*
*
* string tls_ca_cert_path = 4;
* @param value The tlsCaCertPath to set.
* @return This builder for chaining.
*/
public Builder setTlsCaCertPath(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
tlsCaCertPath_ = value;
onChanged();
return this;
}
/**
*
* If set, this file will be used as a root certificate for calls using TLS.
*
*
* string tls_ca_cert_path = 4;
* @return This builder for chaining.
*/
public Builder clearTlsCaCertPath() {
tlsCaCertPath_ = getDefaultInstance().getTlsCaCertPath();
onChanged();
return this;
}
/**
*
* If set, this file will be used as a root certificate for calls using TLS.
*
*
* string tls_ca_cert_path = 4;
* @param value The bytes for tlsCaCertPath to set.
* @return This builder for chaining.
*/
public Builder setTlsCaCertPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
tlsCaCertPath_ = value;
onChanged();
return this;
}
private java.lang.Object tlsClientCertPath_ = "";
/**
*
* If set, this will use client certs for authentication
*
*
* string tls_client_cert_path = 5;
* @return The tlsClientCertPath.
*/
public java.lang.String getTlsClientCertPath() {
java.lang.Object ref = tlsClientCertPath_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tlsClientCertPath_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* If set, this will use client certs for authentication
*
*
* string tls_client_cert_path = 5;
* @return The bytes for tlsClientCertPath.
*/
public com.google.protobuf.ByteString
getTlsClientCertPathBytes() {
java.lang.Object ref = tlsClientCertPath_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tlsClientCertPath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* If set, this will use client certs for authentication
*
*
* string tls_client_cert_path = 5;
* @param value The tlsClientCertPath to set.
* @return This builder for chaining.
*/
public Builder setTlsClientCertPath(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
tlsClientCertPath_ = value;
onChanged();
return this;
}
/**
*
* If set, this will use client certs for authentication
*
*
* string tls_client_cert_path = 5;
* @return This builder for chaining.
*/
public Builder clearTlsClientCertPath() {
tlsClientCertPath_ = getDefaultInstance().getTlsClientCertPath();
onChanged();
return this;
}
/**
*
* If set, this will use client certs for authentication
*
*
* string tls_client_cert_path = 5;
* @param value The bytes for tlsClientCertPath to set.
* @return This builder for chaining.
*/
public Builder setTlsClientCertPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
tlsClientCertPath_ = value;
onChanged();
return this;
}
private java.lang.Object tlsClientKeyPath_ = "";
/**
* string tls_client_key_path = 6;
* @return The tlsClientKeyPath.
*/
public java.lang.String getTlsClientKeyPath() {
java.lang.Object ref = tlsClientKeyPath_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tlsClientKeyPath_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string tls_client_key_path = 6;
* @return The bytes for tlsClientKeyPath.
*/
public com.google.protobuf.ByteString
getTlsClientKeyPathBytes() {
java.lang.Object ref = tlsClientKeyPath_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tlsClientKeyPath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string tls_client_key_path = 6;
* @param value The tlsClientKeyPath to set.
* @return This builder for chaining.
*/
public Builder setTlsClientKeyPath(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
tlsClientKeyPath_ = value;
onChanged();
return this;
}
/**
* string tls_client_key_path = 6;
* @return This builder for chaining.
*/
public Builder clearTlsClientKeyPath() {
tlsClientKeyPath_ = getDefaultInstance().getTlsClientKeyPath();
onChanged();
return this;
}
/**
* string tls_client_key_path = 6;
* @param value The bytes for tlsClientKeyPath to set.
* @return This builder for chaining.
*/
public Builder setTlsClientKeyPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
tlsClientKeyPath_ = value;
onChanged();
return this;
}
private java.lang.Object tlsClientOverrideAuthority_ = "";
/**
* string tls_client_override_authority = 7;
* @return The tlsClientOverrideAuthority.
*/
public java.lang.String getTlsClientOverrideAuthority() {
java.lang.Object ref = tlsClientOverrideAuthority_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tlsClientOverrideAuthority_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string tls_client_override_authority = 7;
* @return The bytes for tlsClientOverrideAuthority.
*/
public com.google.protobuf.ByteString
getTlsClientOverrideAuthorityBytes() {
java.lang.Object ref = tlsClientOverrideAuthority_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tlsClientOverrideAuthority_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string tls_client_override_authority = 7;
* @param value The tlsClientOverrideAuthority to set.
* @return This builder for chaining.
*/
public Builder setTlsClientOverrideAuthority(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
tlsClientOverrideAuthority_ = value;
onChanged();
return this;
}
/**
* string tls_client_override_authority = 7;
* @return This builder for chaining.
*/
public Builder clearTlsClientOverrideAuthority() {
tlsClientOverrideAuthority_ = getDefaultInstance().getTlsClientOverrideAuthority();
onChanged();
return this;
}
/**
* string tls_client_override_authority = 7;
* @param value The bytes for tlsClientOverrideAuthority to set.
* @return This builder for chaining.
*/
public Builder setTlsClientOverrideAuthorityBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
tlsClientOverrideAuthority_ = value;
onChanged();
return this;
}
private java.util.List metadata_ =
java.util.Collections.emptyList();
private void ensureMetadataIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
metadata_ = new java.util.ArrayList(metadata_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilderV3<
polyglot.ConfigProto.CallMetadataEntry, polyglot.ConfigProto.CallMetadataEntry.Builder, polyglot.ConfigProto.CallMetadataEntryOrBuilder> metadataBuilder_;
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
public java.util.List getMetadataList() {
if (metadataBuilder_ == null) {
return java.util.Collections.unmodifiableList(metadata_);
} else {
return metadataBuilder_.getMessageList();
}
}
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
public int getMetadataCount() {
if (metadataBuilder_ == null) {
return metadata_.size();
} else {
return metadataBuilder_.getCount();
}
}
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
public polyglot.ConfigProto.CallMetadataEntry getMetadata(int index) {
if (metadataBuilder_ == null) {
return metadata_.get(index);
} else {
return metadataBuilder_.getMessage(index);
}
}
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
public Builder setMetadata(
int index, polyglot.ConfigProto.CallMetadataEntry value) {
if (metadataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMetadataIsMutable();
metadata_.set(index, value);
onChanged();
} else {
metadataBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
public Builder setMetadata(
int index, polyglot.ConfigProto.CallMetadataEntry.Builder builderForValue) {
if (metadataBuilder_ == null) {
ensureMetadataIsMutable();
metadata_.set(index, builderForValue.build());
onChanged();
} else {
metadataBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
public Builder addMetadata(polyglot.ConfigProto.CallMetadataEntry value) {
if (metadataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMetadataIsMutable();
metadata_.add(value);
onChanged();
} else {
metadataBuilder_.addMessage(value);
}
return this;
}
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
public Builder addMetadata(
int index, polyglot.ConfigProto.CallMetadataEntry value) {
if (metadataBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureMetadataIsMutable();
metadata_.add(index, value);
onChanged();
} else {
metadataBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
public Builder addMetadata(
polyglot.ConfigProto.CallMetadataEntry.Builder builderForValue) {
if (metadataBuilder_ == null) {
ensureMetadataIsMutable();
metadata_.add(builderForValue.build());
onChanged();
} else {
metadataBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
public Builder addMetadata(
int index, polyglot.ConfigProto.CallMetadataEntry.Builder builderForValue) {
if (metadataBuilder_ == null) {
ensureMetadataIsMutable();
metadata_.add(index, builderForValue.build());
onChanged();
} else {
metadataBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
public Builder addAllMetadata(
java.lang.Iterable extends polyglot.ConfigProto.CallMetadataEntry> values) {
if (metadataBuilder_ == null) {
ensureMetadataIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, metadata_);
onChanged();
} else {
metadataBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
public Builder clearMetadata() {
if (metadataBuilder_ == null) {
metadata_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
metadataBuilder_.clear();
}
return this;
}
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
public Builder removeMetadata(int index) {
if (metadataBuilder_ == null) {
ensureMetadataIsMutable();
metadata_.remove(index);
onChanged();
} else {
metadataBuilder_.remove(index);
}
return this;
}
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
public polyglot.ConfigProto.CallMetadataEntry.Builder getMetadataBuilder(
int index) {
return getMetadataFieldBuilder().getBuilder(index);
}
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
public polyglot.ConfigProto.CallMetadataEntryOrBuilder getMetadataOrBuilder(
int index) {
if (metadataBuilder_ == null) {
return metadata_.get(index); } else {
return metadataBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
public java.util.List extends polyglot.ConfigProto.CallMetadataEntryOrBuilder>
getMetadataOrBuilderList() {
if (metadataBuilder_ != null) {
return metadataBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(metadata_);
}
}
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
public polyglot.ConfigProto.CallMetadataEntry.Builder addMetadataBuilder() {
return getMetadataFieldBuilder().addBuilder(
polyglot.ConfigProto.CallMetadataEntry.getDefaultInstance());
}
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
public polyglot.ConfigProto.CallMetadataEntry.Builder addMetadataBuilder(
int index) {
return getMetadataFieldBuilder().addBuilder(
index, polyglot.ConfigProto.CallMetadataEntry.getDefaultInstance());
}
/**
*
* Metadata to be included with the call.
* Entries will be appended to any existing metadata.
* Entries whose name already exists as a metadata entry will have the value appended to the values list.
*
*
* repeated .polyglot.CallMetadataEntry metadata = 8;
*/
public java.util.List
getMetadataBuilderList() {
return getMetadataFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilderV3<
polyglot.ConfigProto.CallMetadataEntry, polyglot.ConfigProto.CallMetadataEntry.Builder, polyglot.ConfigProto.CallMetadataEntryOrBuilder>
getMetadataFieldBuilder() {
if (metadataBuilder_ == null) {
metadataBuilder_ = new com.google.protobuf.RepeatedFieldBuilderV3<
polyglot.ConfigProto.CallMetadataEntry, polyglot.ConfigProto.CallMetadataEntry.Builder, polyglot.ConfigProto.CallMetadataEntryOrBuilder>(
metadata_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
metadata_ = null;
}
return metadataBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:polyglot.CallConfiguration)
}
// @@protoc_insertion_point(class_scope:polyglot.CallConfiguration)
private static final polyglot.ConfigProto.CallConfiguration DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new polyglot.ConfigProto.CallConfiguration();
}
public static polyglot.ConfigProto.CallConfiguration getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CallConfiguration parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CallConfiguration(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public polyglot.ConfigProto.CallConfiguration getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CallMetadataEntryOrBuilder extends
// @@protoc_insertion_point(interface_extends:polyglot.CallMetadataEntry)
com.google.protobuf.MessageOrBuilder {
/**
* string name = 1;
* @return The name.
*/
java.lang.String getName();
/**
* string name = 1;
* @return The bytes for name.
*/
com.google.protobuf.ByteString
getNameBytes();
/**
* string value = 2;
* @return The value.
*/
java.lang.String getValue();
/**
* string value = 2;
* @return The bytes for value.
*/
com.google.protobuf.ByteString
getValueBytes();
}
/**
* Protobuf type {@code polyglot.CallMetadataEntry}
*/
public static final class CallMetadataEntry extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:polyglot.CallMetadataEntry)
CallMetadataEntryOrBuilder {
private static final long serialVersionUID = 0L;
// Use CallMetadataEntry.newBuilder() to construct.
private CallMetadataEntry(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private CallMetadataEntry() {
name_ = "";
value_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new CallMetadataEntry();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private CallMetadataEntry(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
name_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
value_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return polyglot.ConfigProto.internal_static_polyglot_CallMetadataEntry_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return polyglot.ConfigProto.internal_static_polyglot_CallMetadataEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
polyglot.ConfigProto.CallMetadataEntry.class, polyglot.ConfigProto.CallMetadataEntry.Builder.class);
}
public static final int NAME_FIELD_NUMBER = 1;
private volatile java.lang.Object name_;
/**
* string name = 1;
* @return The name.
*/
@java.lang.Override
public java.lang.String getName() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
}
}
/**
* string name = 1;
* @return The bytes for name.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VALUE_FIELD_NUMBER = 2;
private volatile java.lang.Object value_;
/**
* string value = 2;
* @return The value.
*/
@java.lang.Override
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
value_ = s;
return s;
}
}
/**
* string value = 2;
* @return The bytes for value.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getNameBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, name_);
}
if (!getValueBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, value_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getNameBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, name_);
}
if (!getValueBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, value_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof polyglot.ConfigProto.CallMetadataEntry)) {
return super.equals(obj);
}
polyglot.ConfigProto.CallMetadataEntry other = (polyglot.ConfigProto.CallMetadataEntry) obj;
if (!getName()
.equals(other.getName())) return false;
if (!getValue()
.equals(other.getValue())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + NAME_FIELD_NUMBER;
hash = (53 * hash) + getName().hashCode();
hash = (37 * hash) + VALUE_FIELD_NUMBER;
hash = (53 * hash) + getValue().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static polyglot.ConfigProto.CallMetadataEntry parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.CallMetadataEntry parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.CallMetadataEntry parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.CallMetadataEntry parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.CallMetadataEntry parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.CallMetadataEntry parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.CallMetadataEntry parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.CallMetadataEntry parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static polyglot.ConfigProto.CallMetadataEntry parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.CallMetadataEntry parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static polyglot.ConfigProto.CallMetadataEntry parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.CallMetadataEntry parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(polyglot.ConfigProto.CallMetadataEntry prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code polyglot.CallMetadataEntry}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:polyglot.CallMetadataEntry)
polyglot.ConfigProto.CallMetadataEntryOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return polyglot.ConfigProto.internal_static_polyglot_CallMetadataEntry_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return polyglot.ConfigProto.internal_static_polyglot_CallMetadataEntry_fieldAccessorTable
.ensureFieldAccessorsInitialized(
polyglot.ConfigProto.CallMetadataEntry.class, polyglot.ConfigProto.CallMetadataEntry.Builder.class);
}
// Construct using polyglot.ConfigProto.CallMetadataEntry.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
name_ = "";
value_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return polyglot.ConfigProto.internal_static_polyglot_CallMetadataEntry_descriptor;
}
@java.lang.Override
public polyglot.ConfigProto.CallMetadataEntry getDefaultInstanceForType() {
return polyglot.ConfigProto.CallMetadataEntry.getDefaultInstance();
}
@java.lang.Override
public polyglot.ConfigProto.CallMetadataEntry build() {
polyglot.ConfigProto.CallMetadataEntry result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public polyglot.ConfigProto.CallMetadataEntry buildPartial() {
polyglot.ConfigProto.CallMetadataEntry result = new polyglot.ConfigProto.CallMetadataEntry(this);
result.name_ = name_;
result.value_ = value_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof polyglot.ConfigProto.CallMetadataEntry) {
return mergeFrom((polyglot.ConfigProto.CallMetadataEntry)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(polyglot.ConfigProto.CallMetadataEntry other) {
if (other == polyglot.ConfigProto.CallMetadataEntry.getDefaultInstance()) return this;
if (!other.getName().isEmpty()) {
name_ = other.name_;
onChanged();
}
if (!other.getValue().isEmpty()) {
value_ = other.value_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
polyglot.ConfigProto.CallMetadataEntry parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (polyglot.ConfigProto.CallMetadataEntry) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object name_ = "";
/**
* string name = 1;
* @return The name.
*/
public java.lang.String getName() {
java.lang.Object ref = name_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
name_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string name = 1;
* @return The bytes for name.
*/
public com.google.protobuf.ByteString
getNameBytes() {
java.lang.Object ref = name_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
name_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string name = 1;
* @param value The name to set.
* @return This builder for chaining.
*/
public Builder setName(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
name_ = value;
onChanged();
return this;
}
/**
* string name = 1;
* @return This builder for chaining.
*/
public Builder clearName() {
name_ = getDefaultInstance().getName();
onChanged();
return this;
}
/**
* string name = 1;
* @param value The bytes for name to set.
* @return This builder for chaining.
*/
public Builder setNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
name_ = value;
onChanged();
return this;
}
private java.lang.Object value_ = "";
/**
* string value = 2;
* @return The value.
*/
public java.lang.String getValue() {
java.lang.Object ref = value_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
value_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string value = 2;
* @return The bytes for value.
*/
public com.google.protobuf.ByteString
getValueBytes() {
java.lang.Object ref = value_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
value_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string value = 2;
* @param value The value to set.
* @return This builder for chaining.
*/
public Builder setValue(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
value_ = value;
onChanged();
return this;
}
/**
* string value = 2;
* @return This builder for chaining.
*/
public Builder clearValue() {
value_ = getDefaultInstance().getValue();
onChanged();
return this;
}
/**
* string value = 2;
* @param value The bytes for value to set.
* @return This builder for chaining.
*/
public Builder setValueBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
value_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:polyglot.CallMetadataEntry)
}
// @@protoc_insertion_point(class_scope:polyglot.CallMetadataEntry)
private static final polyglot.ConfigProto.CallMetadataEntry DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new polyglot.ConfigProto.CallMetadataEntry();
}
public static polyglot.ConfigProto.CallMetadataEntry getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CallMetadataEntry parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new CallMetadataEntry(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public polyglot.ConfigProto.CallMetadataEntry getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface OauthConfigurationOrBuilder extends
// @@protoc_insertion_point(interface_extends:polyglot.OauthConfiguration)
com.google.protobuf.MessageOrBuilder {
/**
*
* If present, a refresh token will be exchanged for an access token and
* the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.RefreshTokenCredentials refresh_token_credentials = 1;
* @return Whether the refreshTokenCredentials field is set.
*/
boolean hasRefreshTokenCredentials();
/**
*
* If present, a refresh token will be exchanged for an access token and
* the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.RefreshTokenCredentials refresh_token_credentials = 1;
* @return The refreshTokenCredentials.
*/
polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials getRefreshTokenCredentials();
/**
*
* If present, a refresh token will be exchanged for an access token and
* the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.RefreshTokenCredentials refresh_token_credentials = 1;
*/
polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentialsOrBuilder getRefreshTokenCredentialsOrBuilder();
/**
*
* If present, the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.AccessTokenCredentials access_token_credentials = 2;
* @return Whether the accessTokenCredentials field is set.
*/
boolean hasAccessTokenCredentials();
/**
*
* If present, the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.AccessTokenCredentials access_token_credentials = 2;
* @return The accessTokenCredentials.
*/
polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials getAccessTokenCredentials();
/**
*
* If present, the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.AccessTokenCredentials access_token_credentials = 2;
*/
polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentialsOrBuilder getAccessTokenCredentialsOrBuilder();
public polyglot.ConfigProto.OauthConfiguration.CredentialsCase getCredentialsCase();
}
/**
*
* Holds the necessary parameters for adding authentication to requests using
* Oauth2.
*
*
* Protobuf type {@code polyglot.OauthConfiguration}
*/
public static final class OauthConfiguration extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:polyglot.OauthConfiguration)
OauthConfigurationOrBuilder {
private static final long serialVersionUID = 0L;
// Use OauthConfiguration.newBuilder() to construct.
private OauthConfiguration(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private OauthConfiguration() {
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new OauthConfiguration();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private OauthConfiguration(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials.Builder subBuilder = null;
if (credentialsCase_ == 1) {
subBuilder = ((polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials) credentials_).toBuilder();
}
credentials_ =
input.readMessage(polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials) credentials_);
credentials_ = subBuilder.buildPartial();
}
credentialsCase_ = 1;
break;
}
case 18: {
polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials.Builder subBuilder = null;
if (credentialsCase_ == 2) {
subBuilder = ((polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials) credentials_).toBuilder();
}
credentials_ =
input.readMessage(polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom((polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials) credentials_);
credentials_ = subBuilder.buildPartial();
}
credentialsCase_ = 2;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return polyglot.ConfigProto.internal_static_polyglot_OauthConfiguration_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return polyglot.ConfigProto.internal_static_polyglot_OauthConfiguration_fieldAccessorTable
.ensureFieldAccessorsInitialized(
polyglot.ConfigProto.OauthConfiguration.class, polyglot.ConfigProto.OauthConfiguration.Builder.class);
}
public interface RefreshTokenCredentialsOrBuilder extends
// @@protoc_insertion_point(interface_extends:polyglot.OauthConfiguration.RefreshTokenCredentials)
com.google.protobuf.MessageOrBuilder {
/**
*
* A url to talk to in order to exchange the refresh token for an access token.
*
*
* string token_endpoint_url = 1;
* @return The tokenEndpointUrl.
*/
java.lang.String getTokenEndpointUrl();
/**
*
* A url to talk to in order to exchange the refresh token for an access token.
*
*
* string token_endpoint_url = 1;
* @return The bytes for tokenEndpointUrl.
*/
com.google.protobuf.ByteString
getTokenEndpointUrlBytes();
/**
*
* A client identity to use when exchanging tokens.
*
*
* .polyglot.OauthConfiguration.OauthClient client = 2;
* @return Whether the client field is set.
*/
boolean hasClient();
/**
*
* A client identity to use when exchanging tokens.
*
*
* .polyglot.OauthConfiguration.OauthClient client = 2;
* @return The client.
*/
polyglot.ConfigProto.OauthConfiguration.OauthClient getClient();
/**
*
* A client identity to use when exchanging tokens.
*
*
* .polyglot.OauthConfiguration.OauthClient client = 2;
*/
polyglot.ConfigProto.OauthConfiguration.OauthClientOrBuilder getClientOrBuilder();
/**
*
* A path to a file containing a refresh token used for the exchange.
*
*
* string refresh_token_path = 3;
* @return The refreshTokenPath.
*/
java.lang.String getRefreshTokenPath();
/**
*
* A path to a file containing a refresh token used for the exchange.
*
*
* string refresh_token_path = 3;
* @return The bytes for refreshTokenPath.
*/
com.google.protobuf.ByteString
getRefreshTokenPathBytes();
}
/**
*
* Credentials obtained by exchanging a refresh token for an access token.
*
*
* Protobuf type {@code polyglot.OauthConfiguration.RefreshTokenCredentials}
*/
public static final class RefreshTokenCredentials extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:polyglot.OauthConfiguration.RefreshTokenCredentials)
RefreshTokenCredentialsOrBuilder {
private static final long serialVersionUID = 0L;
// Use RefreshTokenCredentials.newBuilder() to construct.
private RefreshTokenCredentials(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private RefreshTokenCredentials() {
tokenEndpointUrl_ = "";
refreshTokenPath_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new RefreshTokenCredentials();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private RefreshTokenCredentials(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
tokenEndpointUrl_ = s;
break;
}
case 18: {
polyglot.ConfigProto.OauthConfiguration.OauthClient.Builder subBuilder = null;
if (client_ != null) {
subBuilder = client_.toBuilder();
}
client_ = input.readMessage(polyglot.ConfigProto.OauthConfiguration.OauthClient.parser(), extensionRegistry);
if (subBuilder != null) {
subBuilder.mergeFrom(client_);
client_ = subBuilder.buildPartial();
}
break;
}
case 26: {
java.lang.String s = input.readStringRequireUtf8();
refreshTokenPath_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return polyglot.ConfigProto.internal_static_polyglot_OauthConfiguration_RefreshTokenCredentials_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return polyglot.ConfigProto.internal_static_polyglot_OauthConfiguration_RefreshTokenCredentials_fieldAccessorTable
.ensureFieldAccessorsInitialized(
polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials.class, polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials.Builder.class);
}
public static final int TOKEN_ENDPOINT_URL_FIELD_NUMBER = 1;
private volatile java.lang.Object tokenEndpointUrl_;
/**
*
* A url to talk to in order to exchange the refresh token for an access token.
*
*
* string token_endpoint_url = 1;
* @return The tokenEndpointUrl.
*/
@java.lang.Override
public java.lang.String getTokenEndpointUrl() {
java.lang.Object ref = tokenEndpointUrl_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tokenEndpointUrl_ = s;
return s;
}
}
/**
*
* A url to talk to in order to exchange the refresh token for an access token.
*
*
* string token_endpoint_url = 1;
* @return The bytes for tokenEndpointUrl.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTokenEndpointUrlBytes() {
java.lang.Object ref = tokenEndpointUrl_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tokenEndpointUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CLIENT_FIELD_NUMBER = 2;
private polyglot.ConfigProto.OauthConfiguration.OauthClient client_;
/**
*
* A client identity to use when exchanging tokens.
*
*
* .polyglot.OauthConfiguration.OauthClient client = 2;
* @return Whether the client field is set.
*/
@java.lang.Override
public boolean hasClient() {
return client_ != null;
}
/**
*
* A client identity to use when exchanging tokens.
*
*
* .polyglot.OauthConfiguration.OauthClient client = 2;
* @return The client.
*/
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration.OauthClient getClient() {
return client_ == null ? polyglot.ConfigProto.OauthConfiguration.OauthClient.getDefaultInstance() : client_;
}
/**
*
* A client identity to use when exchanging tokens.
*
*
* .polyglot.OauthConfiguration.OauthClient client = 2;
*/
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration.OauthClientOrBuilder getClientOrBuilder() {
return getClient();
}
public static final int REFRESH_TOKEN_PATH_FIELD_NUMBER = 3;
private volatile java.lang.Object refreshTokenPath_;
/**
*
* A path to a file containing a refresh token used for the exchange.
*
*
* string refresh_token_path = 3;
* @return The refreshTokenPath.
*/
@java.lang.Override
public java.lang.String getRefreshTokenPath() {
java.lang.Object ref = refreshTokenPath_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
refreshTokenPath_ = s;
return s;
}
}
/**
*
* A path to a file containing a refresh token used for the exchange.
*
*
* string refresh_token_path = 3;
* @return The bytes for refreshTokenPath.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getRefreshTokenPathBytes() {
java.lang.Object ref = refreshTokenPath_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
refreshTokenPath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getTokenEndpointUrlBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, tokenEndpointUrl_);
}
if (client_ != null) {
output.writeMessage(2, getClient());
}
if (!getRefreshTokenPathBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 3, refreshTokenPath_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getTokenEndpointUrlBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, tokenEndpointUrl_);
}
if (client_ != null) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, getClient());
}
if (!getRefreshTokenPathBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(3, refreshTokenPath_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials)) {
return super.equals(obj);
}
polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials other = (polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials) obj;
if (!getTokenEndpointUrl()
.equals(other.getTokenEndpointUrl())) return false;
if (hasClient() != other.hasClient()) return false;
if (hasClient()) {
if (!getClient()
.equals(other.getClient())) return false;
}
if (!getRefreshTokenPath()
.equals(other.getRefreshTokenPath())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TOKEN_ENDPOINT_URL_FIELD_NUMBER;
hash = (53 * hash) + getTokenEndpointUrl().hashCode();
if (hasClient()) {
hash = (37 * hash) + CLIENT_FIELD_NUMBER;
hash = (53 * hash) + getClient().hashCode();
}
hash = (37 * hash) + REFRESH_TOKEN_PATH_FIELD_NUMBER;
hash = (53 * hash) + getRefreshTokenPath().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Credentials obtained by exchanging a refresh token for an access token.
*
*
* Protobuf type {@code polyglot.OauthConfiguration.RefreshTokenCredentials}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:polyglot.OauthConfiguration.RefreshTokenCredentials)
polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentialsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return polyglot.ConfigProto.internal_static_polyglot_OauthConfiguration_RefreshTokenCredentials_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return polyglot.ConfigProto.internal_static_polyglot_OauthConfiguration_RefreshTokenCredentials_fieldAccessorTable
.ensureFieldAccessorsInitialized(
polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials.class, polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials.Builder.class);
}
// Construct using polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
tokenEndpointUrl_ = "";
if (clientBuilder_ == null) {
client_ = null;
} else {
client_ = null;
clientBuilder_ = null;
}
refreshTokenPath_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return polyglot.ConfigProto.internal_static_polyglot_OauthConfiguration_RefreshTokenCredentials_descriptor;
}
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials getDefaultInstanceForType() {
return polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials.getDefaultInstance();
}
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials build() {
polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials buildPartial() {
polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials result = new polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials(this);
result.tokenEndpointUrl_ = tokenEndpointUrl_;
if (clientBuilder_ == null) {
result.client_ = client_;
} else {
result.client_ = clientBuilder_.build();
}
result.refreshTokenPath_ = refreshTokenPath_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials) {
return mergeFrom((polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials other) {
if (other == polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials.getDefaultInstance()) return this;
if (!other.getTokenEndpointUrl().isEmpty()) {
tokenEndpointUrl_ = other.tokenEndpointUrl_;
onChanged();
}
if (other.hasClient()) {
mergeClient(other.getClient());
}
if (!other.getRefreshTokenPath().isEmpty()) {
refreshTokenPath_ = other.refreshTokenPath_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object tokenEndpointUrl_ = "";
/**
*
* A url to talk to in order to exchange the refresh token for an access token.
*
*
* string token_endpoint_url = 1;
* @return The tokenEndpointUrl.
*/
public java.lang.String getTokenEndpointUrl() {
java.lang.Object ref = tokenEndpointUrl_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
tokenEndpointUrl_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* A url to talk to in order to exchange the refresh token for an access token.
*
*
* string token_endpoint_url = 1;
* @return The bytes for tokenEndpointUrl.
*/
public com.google.protobuf.ByteString
getTokenEndpointUrlBytes() {
java.lang.Object ref = tokenEndpointUrl_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
tokenEndpointUrl_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* A url to talk to in order to exchange the refresh token for an access token.
*
*
* string token_endpoint_url = 1;
* @param value The tokenEndpointUrl to set.
* @return This builder for chaining.
*/
public Builder setTokenEndpointUrl(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
tokenEndpointUrl_ = value;
onChanged();
return this;
}
/**
*
* A url to talk to in order to exchange the refresh token for an access token.
*
*
* string token_endpoint_url = 1;
* @return This builder for chaining.
*/
public Builder clearTokenEndpointUrl() {
tokenEndpointUrl_ = getDefaultInstance().getTokenEndpointUrl();
onChanged();
return this;
}
/**
*
* A url to talk to in order to exchange the refresh token for an access token.
*
*
* string token_endpoint_url = 1;
* @param value The bytes for tokenEndpointUrl to set.
* @return This builder for chaining.
*/
public Builder setTokenEndpointUrlBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
tokenEndpointUrl_ = value;
onChanged();
return this;
}
private polyglot.ConfigProto.OauthConfiguration.OauthClient client_;
private com.google.protobuf.SingleFieldBuilderV3<
polyglot.ConfigProto.OauthConfiguration.OauthClient, polyglot.ConfigProto.OauthConfiguration.OauthClient.Builder, polyglot.ConfigProto.OauthConfiguration.OauthClientOrBuilder> clientBuilder_;
/**
*
* A client identity to use when exchanging tokens.
*
*
* .polyglot.OauthConfiguration.OauthClient client = 2;
* @return Whether the client field is set.
*/
public boolean hasClient() {
return clientBuilder_ != null || client_ != null;
}
/**
*
* A client identity to use when exchanging tokens.
*
*
* .polyglot.OauthConfiguration.OauthClient client = 2;
* @return The client.
*/
public polyglot.ConfigProto.OauthConfiguration.OauthClient getClient() {
if (clientBuilder_ == null) {
return client_ == null ? polyglot.ConfigProto.OauthConfiguration.OauthClient.getDefaultInstance() : client_;
} else {
return clientBuilder_.getMessage();
}
}
/**
*
* A client identity to use when exchanging tokens.
*
*
* .polyglot.OauthConfiguration.OauthClient client = 2;
*/
public Builder setClient(polyglot.ConfigProto.OauthConfiguration.OauthClient value) {
if (clientBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
client_ = value;
onChanged();
} else {
clientBuilder_.setMessage(value);
}
return this;
}
/**
*
* A client identity to use when exchanging tokens.
*
*
* .polyglot.OauthConfiguration.OauthClient client = 2;
*/
public Builder setClient(
polyglot.ConfigProto.OauthConfiguration.OauthClient.Builder builderForValue) {
if (clientBuilder_ == null) {
client_ = builderForValue.build();
onChanged();
} else {
clientBuilder_.setMessage(builderForValue.build());
}
return this;
}
/**
*
* A client identity to use when exchanging tokens.
*
*
* .polyglot.OauthConfiguration.OauthClient client = 2;
*/
public Builder mergeClient(polyglot.ConfigProto.OauthConfiguration.OauthClient value) {
if (clientBuilder_ == null) {
if (client_ != null) {
client_ =
polyglot.ConfigProto.OauthConfiguration.OauthClient.newBuilder(client_).mergeFrom(value).buildPartial();
} else {
client_ = value;
}
onChanged();
} else {
clientBuilder_.mergeFrom(value);
}
return this;
}
/**
*
* A client identity to use when exchanging tokens.
*
*
* .polyglot.OauthConfiguration.OauthClient client = 2;
*/
public Builder clearClient() {
if (clientBuilder_ == null) {
client_ = null;
onChanged();
} else {
client_ = null;
clientBuilder_ = null;
}
return this;
}
/**
*
* A client identity to use when exchanging tokens.
*
*
* .polyglot.OauthConfiguration.OauthClient client = 2;
*/
public polyglot.ConfigProto.OauthConfiguration.OauthClient.Builder getClientBuilder() {
onChanged();
return getClientFieldBuilder().getBuilder();
}
/**
*
* A client identity to use when exchanging tokens.
*
*
* .polyglot.OauthConfiguration.OauthClient client = 2;
*/
public polyglot.ConfigProto.OauthConfiguration.OauthClientOrBuilder getClientOrBuilder() {
if (clientBuilder_ != null) {
return clientBuilder_.getMessageOrBuilder();
} else {
return client_ == null ?
polyglot.ConfigProto.OauthConfiguration.OauthClient.getDefaultInstance() : client_;
}
}
/**
*
* A client identity to use when exchanging tokens.
*
*
* .polyglot.OauthConfiguration.OauthClient client = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
polyglot.ConfigProto.OauthConfiguration.OauthClient, polyglot.ConfigProto.OauthConfiguration.OauthClient.Builder, polyglot.ConfigProto.OauthConfiguration.OauthClientOrBuilder>
getClientFieldBuilder() {
if (clientBuilder_ == null) {
clientBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
polyglot.ConfigProto.OauthConfiguration.OauthClient, polyglot.ConfigProto.OauthConfiguration.OauthClient.Builder, polyglot.ConfigProto.OauthConfiguration.OauthClientOrBuilder>(
getClient(),
getParentForChildren(),
isClean());
client_ = null;
}
return clientBuilder_;
}
private java.lang.Object refreshTokenPath_ = "";
/**
*
* A path to a file containing a refresh token used for the exchange.
*
*
* string refresh_token_path = 3;
* @return The refreshTokenPath.
*/
public java.lang.String getRefreshTokenPath() {
java.lang.Object ref = refreshTokenPath_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
refreshTokenPath_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* A path to a file containing a refresh token used for the exchange.
*
*
* string refresh_token_path = 3;
* @return The bytes for refreshTokenPath.
*/
public com.google.protobuf.ByteString
getRefreshTokenPathBytes() {
java.lang.Object ref = refreshTokenPath_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
refreshTokenPath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* A path to a file containing a refresh token used for the exchange.
*
*
* string refresh_token_path = 3;
* @param value The refreshTokenPath to set.
* @return This builder for chaining.
*/
public Builder setRefreshTokenPath(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
refreshTokenPath_ = value;
onChanged();
return this;
}
/**
*
* A path to a file containing a refresh token used for the exchange.
*
*
* string refresh_token_path = 3;
* @return This builder for chaining.
*/
public Builder clearRefreshTokenPath() {
refreshTokenPath_ = getDefaultInstance().getRefreshTokenPath();
onChanged();
return this;
}
/**
*
* A path to a file containing a refresh token used for the exchange.
*
*
* string refresh_token_path = 3;
* @param value The bytes for refreshTokenPath to set.
* @return This builder for chaining.
*/
public Builder setRefreshTokenPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
refreshTokenPath_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:polyglot.OauthConfiguration.RefreshTokenCredentials)
}
// @@protoc_insertion_point(class_scope:polyglot.OauthConfiguration.RefreshTokenCredentials)
private static final polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials();
}
public static polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public RefreshTokenCredentials parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new RefreshTokenCredentials(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface AccessTokenCredentialsOrBuilder extends
// @@protoc_insertion_point(interface_extends:polyglot.OauthConfiguration.AccessTokenCredentials)
com.google.protobuf.MessageOrBuilder {
/**
*
* A path to a file containing an access token.
*
*
* string access_token_path = 1;
* @return The accessTokenPath.
*/
java.lang.String getAccessTokenPath();
/**
*
* A path to a file containing an access token.
*
*
* string access_token_path = 1;
* @return The bytes for accessTokenPath.
*/
com.google.protobuf.ByteString
getAccessTokenPathBytes();
}
/**
*
* Credentials used directly adding an access token to the rpc.
*
*
* Protobuf type {@code polyglot.OauthConfiguration.AccessTokenCredentials}
*/
public static final class AccessTokenCredentials extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:polyglot.OauthConfiguration.AccessTokenCredentials)
AccessTokenCredentialsOrBuilder {
private static final long serialVersionUID = 0L;
// Use AccessTokenCredentials.newBuilder() to construct.
private AccessTokenCredentials(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private AccessTokenCredentials() {
accessTokenPath_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new AccessTokenCredentials();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private AccessTokenCredentials(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
accessTokenPath_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return polyglot.ConfigProto.internal_static_polyglot_OauthConfiguration_AccessTokenCredentials_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return polyglot.ConfigProto.internal_static_polyglot_OauthConfiguration_AccessTokenCredentials_fieldAccessorTable
.ensureFieldAccessorsInitialized(
polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials.class, polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials.Builder.class);
}
public static final int ACCESS_TOKEN_PATH_FIELD_NUMBER = 1;
private volatile java.lang.Object accessTokenPath_;
/**
*
* A path to a file containing an access token.
*
*
* string access_token_path = 1;
* @return The accessTokenPath.
*/
@java.lang.Override
public java.lang.String getAccessTokenPath() {
java.lang.Object ref = accessTokenPath_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
accessTokenPath_ = s;
return s;
}
}
/**
*
* A path to a file containing an access token.
*
*
* string access_token_path = 1;
* @return The bytes for accessTokenPath.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getAccessTokenPathBytes() {
java.lang.Object ref = accessTokenPath_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
accessTokenPath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getAccessTokenPathBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, accessTokenPath_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getAccessTokenPathBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, accessTokenPath_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials)) {
return super.equals(obj);
}
polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials other = (polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials) obj;
if (!getAccessTokenPath()
.equals(other.getAccessTokenPath())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ACCESS_TOKEN_PATH_FIELD_NUMBER;
hash = (53 * hash) + getAccessTokenPath().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Credentials used directly adding an access token to the rpc.
*
*
* Protobuf type {@code polyglot.OauthConfiguration.AccessTokenCredentials}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:polyglot.OauthConfiguration.AccessTokenCredentials)
polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentialsOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return polyglot.ConfigProto.internal_static_polyglot_OauthConfiguration_AccessTokenCredentials_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return polyglot.ConfigProto.internal_static_polyglot_OauthConfiguration_AccessTokenCredentials_fieldAccessorTable
.ensureFieldAccessorsInitialized(
polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials.class, polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials.Builder.class);
}
// Construct using polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
accessTokenPath_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return polyglot.ConfigProto.internal_static_polyglot_OauthConfiguration_AccessTokenCredentials_descriptor;
}
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials getDefaultInstanceForType() {
return polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials.getDefaultInstance();
}
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials build() {
polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials buildPartial() {
polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials result = new polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials(this);
result.accessTokenPath_ = accessTokenPath_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials) {
return mergeFrom((polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials other) {
if (other == polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials.getDefaultInstance()) return this;
if (!other.getAccessTokenPath().isEmpty()) {
accessTokenPath_ = other.accessTokenPath_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object accessTokenPath_ = "";
/**
*
* A path to a file containing an access token.
*
*
* string access_token_path = 1;
* @return The accessTokenPath.
*/
public java.lang.String getAccessTokenPath() {
java.lang.Object ref = accessTokenPath_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
accessTokenPath_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* A path to a file containing an access token.
*
*
* string access_token_path = 1;
* @return The bytes for accessTokenPath.
*/
public com.google.protobuf.ByteString
getAccessTokenPathBytes() {
java.lang.Object ref = accessTokenPath_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
accessTokenPath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* A path to a file containing an access token.
*
*
* string access_token_path = 1;
* @param value The accessTokenPath to set.
* @return This builder for chaining.
*/
public Builder setAccessTokenPath(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
accessTokenPath_ = value;
onChanged();
return this;
}
/**
*
* A path to a file containing an access token.
*
*
* string access_token_path = 1;
* @return This builder for chaining.
*/
public Builder clearAccessTokenPath() {
accessTokenPath_ = getDefaultInstance().getAccessTokenPath();
onChanged();
return this;
}
/**
*
* A path to a file containing an access token.
*
*
* string access_token_path = 1;
* @param value The bytes for accessTokenPath to set.
* @return This builder for chaining.
*/
public Builder setAccessTokenPathBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
accessTokenPath_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:polyglot.OauthConfiguration.AccessTokenCredentials)
}
// @@protoc_insertion_point(class_scope:polyglot.OauthConfiguration.AccessTokenCredentials)
private static final polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials();
}
public static polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public AccessTokenCredentials parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new AccessTokenCredentials(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface OauthClientOrBuilder extends
// @@protoc_insertion_point(interface_extends:polyglot.OauthConfiguration.OauthClient)
com.google.protobuf.MessageOrBuilder {
/**
* string id = 1;
* @return The id.
*/
java.lang.String getId();
/**
* string id = 1;
* @return The bytes for id.
*/
com.google.protobuf.ByteString
getIdBytes();
/**
* string secret = 2;
* @return The secret.
*/
java.lang.String getSecret();
/**
* string secret = 2;
* @return The bytes for secret.
*/
com.google.protobuf.ByteString
getSecretBytes();
}
/**
*
* Contains the necessary information to identify ourselves as an Oauth2 client.
*
*
* Protobuf type {@code polyglot.OauthConfiguration.OauthClient}
*/
public static final class OauthClient extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:polyglot.OauthConfiguration.OauthClient)
OauthClientOrBuilder {
private static final long serialVersionUID = 0L;
// Use OauthClient.newBuilder() to construct.
private OauthClient(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private OauthClient() {
id_ = "";
secret_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new OauthClient();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private OauthClient(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
id_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
secret_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return polyglot.ConfigProto.internal_static_polyglot_OauthConfiguration_OauthClient_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return polyglot.ConfigProto.internal_static_polyglot_OauthConfiguration_OauthClient_fieldAccessorTable
.ensureFieldAccessorsInitialized(
polyglot.ConfigProto.OauthConfiguration.OauthClient.class, polyglot.ConfigProto.OauthConfiguration.OauthClient.Builder.class);
}
public static final int ID_FIELD_NUMBER = 1;
private volatile java.lang.Object id_;
/**
* string id = 1;
* @return The id.
*/
@java.lang.Override
public java.lang.String getId() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
}
}
/**
* string id = 1;
* @return The bytes for id.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SECRET_FIELD_NUMBER = 2;
private volatile java.lang.Object secret_;
/**
* string secret = 2;
* @return The secret.
*/
@java.lang.Override
public java.lang.String getSecret() {
java.lang.Object ref = secret_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
secret_ = s;
return s;
}
}
/**
* string secret = 2;
* @return The bytes for secret.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSecretBytes() {
java.lang.Object ref = secret_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
secret_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getIdBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, id_);
}
if (!getSecretBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, secret_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getIdBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, id_);
}
if (!getSecretBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, secret_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof polyglot.ConfigProto.OauthConfiguration.OauthClient)) {
return super.equals(obj);
}
polyglot.ConfigProto.OauthConfiguration.OauthClient other = (polyglot.ConfigProto.OauthConfiguration.OauthClient) obj;
if (!getId()
.equals(other.getId())) return false;
if (!getSecret()
.equals(other.getSecret())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ID_FIELD_NUMBER;
hash = (53 * hash) + getId().hashCode();
hash = (37 * hash) + SECRET_FIELD_NUMBER;
hash = (53 * hash) + getSecret().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static polyglot.ConfigProto.OauthConfiguration.OauthClient parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.OauthConfiguration.OauthClient parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.OauthConfiguration.OauthClient parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.OauthConfiguration.OauthClient parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.OauthConfiguration.OauthClient parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.OauthConfiguration.OauthClient parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.OauthConfiguration.OauthClient parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.OauthConfiguration.OauthClient parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static polyglot.ConfigProto.OauthConfiguration.OauthClient parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.OauthConfiguration.OauthClient parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static polyglot.ConfigProto.OauthConfiguration.OauthClient parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.OauthConfiguration.OauthClient parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(polyglot.ConfigProto.OauthConfiguration.OauthClient prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Contains the necessary information to identify ourselves as an Oauth2 client.
*
*
* Protobuf type {@code polyglot.OauthConfiguration.OauthClient}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:polyglot.OauthConfiguration.OauthClient)
polyglot.ConfigProto.OauthConfiguration.OauthClientOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return polyglot.ConfigProto.internal_static_polyglot_OauthConfiguration_OauthClient_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return polyglot.ConfigProto.internal_static_polyglot_OauthConfiguration_OauthClient_fieldAccessorTable
.ensureFieldAccessorsInitialized(
polyglot.ConfigProto.OauthConfiguration.OauthClient.class, polyglot.ConfigProto.OauthConfiguration.OauthClient.Builder.class);
}
// Construct using polyglot.ConfigProto.OauthConfiguration.OauthClient.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
id_ = "";
secret_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return polyglot.ConfigProto.internal_static_polyglot_OauthConfiguration_OauthClient_descriptor;
}
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration.OauthClient getDefaultInstanceForType() {
return polyglot.ConfigProto.OauthConfiguration.OauthClient.getDefaultInstance();
}
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration.OauthClient build() {
polyglot.ConfigProto.OauthConfiguration.OauthClient result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration.OauthClient buildPartial() {
polyglot.ConfigProto.OauthConfiguration.OauthClient result = new polyglot.ConfigProto.OauthConfiguration.OauthClient(this);
result.id_ = id_;
result.secret_ = secret_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof polyglot.ConfigProto.OauthConfiguration.OauthClient) {
return mergeFrom((polyglot.ConfigProto.OauthConfiguration.OauthClient)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(polyglot.ConfigProto.OauthConfiguration.OauthClient other) {
if (other == polyglot.ConfigProto.OauthConfiguration.OauthClient.getDefaultInstance()) return this;
if (!other.getId().isEmpty()) {
id_ = other.id_;
onChanged();
}
if (!other.getSecret().isEmpty()) {
secret_ = other.secret_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
polyglot.ConfigProto.OauthConfiguration.OauthClient parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (polyglot.ConfigProto.OauthConfiguration.OauthClient) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private java.lang.Object id_ = "";
/**
* string id = 1;
* @return The id.
*/
public java.lang.String getId() {
java.lang.Object ref = id_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
id_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string id = 1;
* @return The bytes for id.
*/
public com.google.protobuf.ByteString
getIdBytes() {
java.lang.Object ref = id_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
id_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string id = 1;
* @param value The id to set.
* @return This builder for chaining.
*/
public Builder setId(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
id_ = value;
onChanged();
return this;
}
/**
* string id = 1;
* @return This builder for chaining.
*/
public Builder clearId() {
id_ = getDefaultInstance().getId();
onChanged();
return this;
}
/**
* string id = 1;
* @param value The bytes for id to set.
* @return This builder for chaining.
*/
public Builder setIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
id_ = value;
onChanged();
return this;
}
private java.lang.Object secret_ = "";
/**
* string secret = 2;
* @return The secret.
*/
public java.lang.String getSecret() {
java.lang.Object ref = secret_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
secret_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string secret = 2;
* @return The bytes for secret.
*/
public com.google.protobuf.ByteString
getSecretBytes() {
java.lang.Object ref = secret_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
secret_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string secret = 2;
* @param value The secret to set.
* @return This builder for chaining.
*/
public Builder setSecret(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
secret_ = value;
onChanged();
return this;
}
/**
* string secret = 2;
* @return This builder for chaining.
*/
public Builder clearSecret() {
secret_ = getDefaultInstance().getSecret();
onChanged();
return this;
}
/**
* string secret = 2;
* @param value The bytes for secret to set.
* @return This builder for chaining.
*/
public Builder setSecretBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
secret_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:polyglot.OauthConfiguration.OauthClient)
}
// @@protoc_insertion_point(class_scope:polyglot.OauthConfiguration.OauthClient)
private static final polyglot.ConfigProto.OauthConfiguration.OauthClient DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new polyglot.ConfigProto.OauthConfiguration.OauthClient();
}
public static polyglot.ConfigProto.OauthConfiguration.OauthClient getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public OauthClient parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new OauthClient(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration.OauthClient getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int credentialsCase_ = 0;
private java.lang.Object credentials_;
public enum CredentialsCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
REFRESH_TOKEN_CREDENTIALS(1),
ACCESS_TOKEN_CREDENTIALS(2),
CREDENTIALS_NOT_SET(0);
private final int value;
private CredentialsCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static CredentialsCase valueOf(int value) {
return forNumber(value);
}
public static CredentialsCase forNumber(int value) {
switch (value) {
case 1: return REFRESH_TOKEN_CREDENTIALS;
case 2: return ACCESS_TOKEN_CREDENTIALS;
case 0: return CREDENTIALS_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public CredentialsCase
getCredentialsCase() {
return CredentialsCase.forNumber(
credentialsCase_);
}
public static final int REFRESH_TOKEN_CREDENTIALS_FIELD_NUMBER = 1;
/**
*
* If present, a refresh token will be exchanged for an access token and
* the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.RefreshTokenCredentials refresh_token_credentials = 1;
* @return Whether the refreshTokenCredentials field is set.
*/
@java.lang.Override
public boolean hasRefreshTokenCredentials() {
return credentialsCase_ == 1;
}
/**
*
* If present, a refresh token will be exchanged for an access token and
* the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.RefreshTokenCredentials refresh_token_credentials = 1;
* @return The refreshTokenCredentials.
*/
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials getRefreshTokenCredentials() {
if (credentialsCase_ == 1) {
return (polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials) credentials_;
}
return polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials.getDefaultInstance();
}
/**
*
* If present, a refresh token will be exchanged for an access token and
* the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.RefreshTokenCredentials refresh_token_credentials = 1;
*/
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentialsOrBuilder getRefreshTokenCredentialsOrBuilder() {
if (credentialsCase_ == 1) {
return (polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials) credentials_;
}
return polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials.getDefaultInstance();
}
public static final int ACCESS_TOKEN_CREDENTIALS_FIELD_NUMBER = 2;
/**
*
* If present, the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.AccessTokenCredentials access_token_credentials = 2;
* @return Whether the accessTokenCredentials field is set.
*/
@java.lang.Override
public boolean hasAccessTokenCredentials() {
return credentialsCase_ == 2;
}
/**
*
* If present, the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.AccessTokenCredentials access_token_credentials = 2;
* @return The accessTokenCredentials.
*/
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials getAccessTokenCredentials() {
if (credentialsCase_ == 2) {
return (polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials) credentials_;
}
return polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials.getDefaultInstance();
}
/**
*
* If present, the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.AccessTokenCredentials access_token_credentials = 2;
*/
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentialsOrBuilder getAccessTokenCredentialsOrBuilder() {
if (credentialsCase_ == 2) {
return (polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials) credentials_;
}
return polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials.getDefaultInstance();
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (credentialsCase_ == 1) {
output.writeMessage(1, (polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials) credentials_);
}
if (credentialsCase_ == 2) {
output.writeMessage(2, (polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials) credentials_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (credentialsCase_ == 1) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, (polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials) credentials_);
}
if (credentialsCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(2, (polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials) credentials_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof polyglot.ConfigProto.OauthConfiguration)) {
return super.equals(obj);
}
polyglot.ConfigProto.OauthConfiguration other = (polyglot.ConfigProto.OauthConfiguration) obj;
if (!getCredentialsCase().equals(other.getCredentialsCase())) return false;
switch (credentialsCase_) {
case 1:
if (!getRefreshTokenCredentials()
.equals(other.getRefreshTokenCredentials())) return false;
break;
case 2:
if (!getAccessTokenCredentials()
.equals(other.getAccessTokenCredentials())) return false;
break;
case 0:
default:
}
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
switch (credentialsCase_) {
case 1:
hash = (37 * hash) + REFRESH_TOKEN_CREDENTIALS_FIELD_NUMBER;
hash = (53 * hash) + getRefreshTokenCredentials().hashCode();
break;
case 2:
hash = (37 * hash) + ACCESS_TOKEN_CREDENTIALS_FIELD_NUMBER;
hash = (53 * hash) + getAccessTokenCredentials().hashCode();
break;
case 0:
default:
}
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static polyglot.ConfigProto.OauthConfiguration parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.OauthConfiguration parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.OauthConfiguration parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.OauthConfiguration parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.OauthConfiguration parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.OauthConfiguration parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.OauthConfiguration parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.OauthConfiguration parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static polyglot.ConfigProto.OauthConfiguration parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.OauthConfiguration parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static polyglot.ConfigProto.OauthConfiguration parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.OauthConfiguration parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(polyglot.ConfigProto.OauthConfiguration prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Holds the necessary parameters for adding authentication to requests using
* Oauth2.
*
*
* Protobuf type {@code polyglot.OauthConfiguration}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:polyglot.OauthConfiguration)
polyglot.ConfigProto.OauthConfigurationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return polyglot.ConfigProto.internal_static_polyglot_OauthConfiguration_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return polyglot.ConfigProto.internal_static_polyglot_OauthConfiguration_fieldAccessorTable
.ensureFieldAccessorsInitialized(
polyglot.ConfigProto.OauthConfiguration.class, polyglot.ConfigProto.OauthConfiguration.Builder.class);
}
// Construct using polyglot.ConfigProto.OauthConfiguration.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
credentialsCase_ = 0;
credentials_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return polyglot.ConfigProto.internal_static_polyglot_OauthConfiguration_descriptor;
}
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration getDefaultInstanceForType() {
return polyglot.ConfigProto.OauthConfiguration.getDefaultInstance();
}
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration build() {
polyglot.ConfigProto.OauthConfiguration result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration buildPartial() {
polyglot.ConfigProto.OauthConfiguration result = new polyglot.ConfigProto.OauthConfiguration(this);
if (credentialsCase_ == 1) {
if (refreshTokenCredentialsBuilder_ == null) {
result.credentials_ = credentials_;
} else {
result.credentials_ = refreshTokenCredentialsBuilder_.build();
}
}
if (credentialsCase_ == 2) {
if (accessTokenCredentialsBuilder_ == null) {
result.credentials_ = credentials_;
} else {
result.credentials_ = accessTokenCredentialsBuilder_.build();
}
}
result.credentialsCase_ = credentialsCase_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof polyglot.ConfigProto.OauthConfiguration) {
return mergeFrom((polyglot.ConfigProto.OauthConfiguration)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(polyglot.ConfigProto.OauthConfiguration other) {
if (other == polyglot.ConfigProto.OauthConfiguration.getDefaultInstance()) return this;
switch (other.getCredentialsCase()) {
case REFRESH_TOKEN_CREDENTIALS: {
mergeRefreshTokenCredentials(other.getRefreshTokenCredentials());
break;
}
case ACCESS_TOKEN_CREDENTIALS: {
mergeAccessTokenCredentials(other.getAccessTokenCredentials());
break;
}
case CREDENTIALS_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
polyglot.ConfigProto.OauthConfiguration parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (polyglot.ConfigProto.OauthConfiguration) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int credentialsCase_ = 0;
private java.lang.Object credentials_;
public CredentialsCase
getCredentialsCase() {
return CredentialsCase.forNumber(
credentialsCase_);
}
public Builder clearCredentials() {
credentialsCase_ = 0;
credentials_ = null;
onChanged();
return this;
}
private com.google.protobuf.SingleFieldBuilderV3<
polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials, polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials.Builder, polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentialsOrBuilder> refreshTokenCredentialsBuilder_;
/**
*
* If present, a refresh token will be exchanged for an access token and
* the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.RefreshTokenCredentials refresh_token_credentials = 1;
* @return Whether the refreshTokenCredentials field is set.
*/
@java.lang.Override
public boolean hasRefreshTokenCredentials() {
return credentialsCase_ == 1;
}
/**
*
* If present, a refresh token will be exchanged for an access token and
* the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.RefreshTokenCredentials refresh_token_credentials = 1;
* @return The refreshTokenCredentials.
*/
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials getRefreshTokenCredentials() {
if (refreshTokenCredentialsBuilder_ == null) {
if (credentialsCase_ == 1) {
return (polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials) credentials_;
}
return polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials.getDefaultInstance();
} else {
if (credentialsCase_ == 1) {
return refreshTokenCredentialsBuilder_.getMessage();
}
return polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials.getDefaultInstance();
}
}
/**
*
* If present, a refresh token will be exchanged for an access token and
* the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.RefreshTokenCredentials refresh_token_credentials = 1;
*/
public Builder setRefreshTokenCredentials(polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials value) {
if (refreshTokenCredentialsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
credentials_ = value;
onChanged();
} else {
refreshTokenCredentialsBuilder_.setMessage(value);
}
credentialsCase_ = 1;
return this;
}
/**
*
* If present, a refresh token will be exchanged for an access token and
* the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.RefreshTokenCredentials refresh_token_credentials = 1;
*/
public Builder setRefreshTokenCredentials(
polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials.Builder builderForValue) {
if (refreshTokenCredentialsBuilder_ == null) {
credentials_ = builderForValue.build();
onChanged();
} else {
refreshTokenCredentialsBuilder_.setMessage(builderForValue.build());
}
credentialsCase_ = 1;
return this;
}
/**
*
* If present, a refresh token will be exchanged for an access token and
* the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.RefreshTokenCredentials refresh_token_credentials = 1;
*/
public Builder mergeRefreshTokenCredentials(polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials value) {
if (refreshTokenCredentialsBuilder_ == null) {
if (credentialsCase_ == 1 &&
credentials_ != polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials.getDefaultInstance()) {
credentials_ = polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials.newBuilder((polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials) credentials_)
.mergeFrom(value).buildPartial();
} else {
credentials_ = value;
}
onChanged();
} else {
if (credentialsCase_ == 1) {
refreshTokenCredentialsBuilder_.mergeFrom(value);
}
refreshTokenCredentialsBuilder_.setMessage(value);
}
credentialsCase_ = 1;
return this;
}
/**
*
* If present, a refresh token will be exchanged for an access token and
* the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.RefreshTokenCredentials refresh_token_credentials = 1;
*/
public Builder clearRefreshTokenCredentials() {
if (refreshTokenCredentialsBuilder_ == null) {
if (credentialsCase_ == 1) {
credentialsCase_ = 0;
credentials_ = null;
onChanged();
}
} else {
if (credentialsCase_ == 1) {
credentialsCase_ = 0;
credentials_ = null;
}
refreshTokenCredentialsBuilder_.clear();
}
return this;
}
/**
*
* If present, a refresh token will be exchanged for an access token and
* the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.RefreshTokenCredentials refresh_token_credentials = 1;
*/
public polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials.Builder getRefreshTokenCredentialsBuilder() {
return getRefreshTokenCredentialsFieldBuilder().getBuilder();
}
/**
*
* If present, a refresh token will be exchanged for an access token and
* the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.RefreshTokenCredentials refresh_token_credentials = 1;
*/
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentialsOrBuilder getRefreshTokenCredentialsOrBuilder() {
if ((credentialsCase_ == 1) && (refreshTokenCredentialsBuilder_ != null)) {
return refreshTokenCredentialsBuilder_.getMessageOrBuilder();
} else {
if (credentialsCase_ == 1) {
return (polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials) credentials_;
}
return polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials.getDefaultInstance();
}
}
/**
*
* If present, a refresh token will be exchanged for an access token and
* the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.RefreshTokenCredentials refresh_token_credentials = 1;
*/
private com.google.protobuf.SingleFieldBuilderV3<
polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials, polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials.Builder, polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentialsOrBuilder>
getRefreshTokenCredentialsFieldBuilder() {
if (refreshTokenCredentialsBuilder_ == null) {
if (!(credentialsCase_ == 1)) {
credentials_ = polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials.getDefaultInstance();
}
refreshTokenCredentialsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials, polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials.Builder, polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentialsOrBuilder>(
(polyglot.ConfigProto.OauthConfiguration.RefreshTokenCredentials) credentials_,
getParentForChildren(),
isClean());
credentials_ = null;
}
credentialsCase_ = 1;
onChanged();;
return refreshTokenCredentialsBuilder_;
}
private com.google.protobuf.SingleFieldBuilderV3<
polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials, polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials.Builder, polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentialsOrBuilder> accessTokenCredentialsBuilder_;
/**
*
* If present, the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.AccessTokenCredentials access_token_credentials = 2;
* @return Whether the accessTokenCredentials field is set.
*/
@java.lang.Override
public boolean hasAccessTokenCredentials() {
return credentialsCase_ == 2;
}
/**
*
* If present, the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.AccessTokenCredentials access_token_credentials = 2;
* @return The accessTokenCredentials.
*/
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials getAccessTokenCredentials() {
if (accessTokenCredentialsBuilder_ == null) {
if (credentialsCase_ == 2) {
return (polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials) credentials_;
}
return polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials.getDefaultInstance();
} else {
if (credentialsCase_ == 2) {
return accessTokenCredentialsBuilder_.getMessage();
}
return polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials.getDefaultInstance();
}
}
/**
*
* If present, the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.AccessTokenCredentials access_token_credentials = 2;
*/
public Builder setAccessTokenCredentials(polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials value) {
if (accessTokenCredentialsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
credentials_ = value;
onChanged();
} else {
accessTokenCredentialsBuilder_.setMessage(value);
}
credentialsCase_ = 2;
return this;
}
/**
*
* If present, the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.AccessTokenCredentials access_token_credentials = 2;
*/
public Builder setAccessTokenCredentials(
polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials.Builder builderForValue) {
if (accessTokenCredentialsBuilder_ == null) {
credentials_ = builderForValue.build();
onChanged();
} else {
accessTokenCredentialsBuilder_.setMessage(builderForValue.build());
}
credentialsCase_ = 2;
return this;
}
/**
*
* If present, the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.AccessTokenCredentials access_token_credentials = 2;
*/
public Builder mergeAccessTokenCredentials(polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials value) {
if (accessTokenCredentialsBuilder_ == null) {
if (credentialsCase_ == 2 &&
credentials_ != polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials.getDefaultInstance()) {
credentials_ = polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials.newBuilder((polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials) credentials_)
.mergeFrom(value).buildPartial();
} else {
credentials_ = value;
}
onChanged();
} else {
if (credentialsCase_ == 2) {
accessTokenCredentialsBuilder_.mergeFrom(value);
}
accessTokenCredentialsBuilder_.setMessage(value);
}
credentialsCase_ = 2;
return this;
}
/**
*
* If present, the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.AccessTokenCredentials access_token_credentials = 2;
*/
public Builder clearAccessTokenCredentials() {
if (accessTokenCredentialsBuilder_ == null) {
if (credentialsCase_ == 2) {
credentialsCase_ = 0;
credentials_ = null;
onChanged();
}
} else {
if (credentialsCase_ == 2) {
credentialsCase_ = 0;
credentials_ = null;
}
accessTokenCredentialsBuilder_.clear();
}
return this;
}
/**
*
* If present, the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.AccessTokenCredentials access_token_credentials = 2;
*/
public polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials.Builder getAccessTokenCredentialsBuilder() {
return getAccessTokenCredentialsFieldBuilder().getBuilder();
}
/**
*
* If present, the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.AccessTokenCredentials access_token_credentials = 2;
*/
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentialsOrBuilder getAccessTokenCredentialsOrBuilder() {
if ((credentialsCase_ == 2) && (accessTokenCredentialsBuilder_ != null)) {
return accessTokenCredentialsBuilder_.getMessageOrBuilder();
} else {
if (credentialsCase_ == 2) {
return (polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials) credentials_;
}
return polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials.getDefaultInstance();
}
}
/**
*
* If present, the access token will be used to authenticate the request.
*
*
* .polyglot.OauthConfiguration.AccessTokenCredentials access_token_credentials = 2;
*/
private com.google.protobuf.SingleFieldBuilderV3<
polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials, polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials.Builder, polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentialsOrBuilder>
getAccessTokenCredentialsFieldBuilder() {
if (accessTokenCredentialsBuilder_ == null) {
if (!(credentialsCase_ == 2)) {
credentials_ = polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials.getDefaultInstance();
}
accessTokenCredentialsBuilder_ = new com.google.protobuf.SingleFieldBuilderV3<
polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials, polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials.Builder, polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentialsOrBuilder>(
(polyglot.ConfigProto.OauthConfiguration.AccessTokenCredentials) credentials_,
getParentForChildren(),
isClean());
credentials_ = null;
}
credentialsCase_ = 2;
onChanged();;
return accessTokenCredentialsBuilder_;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:polyglot.OauthConfiguration)
}
// @@protoc_insertion_point(class_scope:polyglot.OauthConfiguration)
private static final polyglot.ConfigProto.OauthConfiguration DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new polyglot.ConfigProto.OauthConfiguration();
}
public static polyglot.ConfigProto.OauthConfiguration getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public OauthConfiguration parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new OauthConfiguration(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public polyglot.ConfigProto.OauthConfiguration getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface OutputConfigurationOrBuilder extends
// @@protoc_insertion_point(interface_extends:polyglot.OutputConfiguration)
com.google.protobuf.MessageOrBuilder {
/**
* .polyglot.OutputConfiguration.Destination destination = 1;
* @return The enum numeric value on the wire for destination.
*/
int getDestinationValue();
/**
* .polyglot.OutputConfiguration.Destination destination = 1;
* @return The destination.
*/
polyglot.ConfigProto.OutputConfiguration.Destination getDestination();
/**
*
* When using a destination of type FILE, indicates which file to write to.
*
*
* string file_path = 2;
* @return The filePath.
*/
java.lang.String getFilePath();
/**
*
* When using a destination of type FILE, indicates which file to write to.
*
*
* string file_path = 2;
* @return The bytes for filePath.
*/
com.google.protobuf.ByteString
getFilePathBytes();
}
/**
*
* Contains parameters controlling the output protos of polyglot.
*
*
* Protobuf type {@code polyglot.OutputConfiguration}
*/
public static final class OutputConfiguration extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:polyglot.OutputConfiguration)
OutputConfigurationOrBuilder {
private static final long serialVersionUID = 0L;
// Use OutputConfiguration.newBuilder() to construct.
private OutputConfiguration(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private OutputConfiguration() {
destination_ = 0;
filePath_ = "";
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new OutputConfiguration();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private OutputConfiguration(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
int rawValue = input.readEnum();
destination_ = rawValue;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
filePath_ = s;
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return polyglot.ConfigProto.internal_static_polyglot_OutputConfiguration_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return polyglot.ConfigProto.internal_static_polyglot_OutputConfiguration_fieldAccessorTable
.ensureFieldAccessorsInitialized(
polyglot.ConfigProto.OutputConfiguration.class, polyglot.ConfigProto.OutputConfiguration.Builder.class);
}
/**
* Protobuf enum {@code polyglot.OutputConfiguration.Destination}
*/
public enum Destination
implements com.google.protobuf.ProtocolMessageEnum {
/**
*
* Content is written to standard output.
*
*
* STDOUT = 0;
*/
STDOUT(0),
/**
*
* Content is written to the execution log, i.e., to standard error.
*
*
* LOG = 1;
*/
LOG(1),
/**
*
* Content is written to a file.
*
*
* FILE = 2;
*/
FILE(2),
UNRECOGNIZED(-1),
;
/**
*
* Content is written to standard output.
*
*
* STDOUT = 0;
*/
public static final int STDOUT_VALUE = 0;
/**
*
* Content is written to the execution log, i.e., to standard error.
*
*
* LOG = 1;
*/
public static final int LOG_VALUE = 1;
/**
*
* Content is written to a file.
*
*
* FILE = 2;
*/
public static final int FILE_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Destination valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Destination forNumber(int value) {
switch (value) {
case 0: return STDOUT;
case 1: return LOG;
case 2: return FILE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Destination> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Destination findValueByNumber(int number) {
return Destination.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return polyglot.ConfigProto.OutputConfiguration.getDescriptor().getEnumTypes().get(0);
}
private static final Destination[] VALUES = values();
public static Destination valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Destination(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:polyglot.OutputConfiguration.Destination)
}
public static final int DESTINATION_FIELD_NUMBER = 1;
private int destination_;
/**
* .polyglot.OutputConfiguration.Destination destination = 1;
* @return The enum numeric value on the wire for destination.
*/
@java.lang.Override public int getDestinationValue() {
return destination_;
}
/**
* .polyglot.OutputConfiguration.Destination destination = 1;
* @return The destination.
*/
@java.lang.Override public polyglot.ConfigProto.OutputConfiguration.Destination getDestination() {
@SuppressWarnings("deprecation")
polyglot.ConfigProto.OutputConfiguration.Destination result = polyglot.ConfigProto.OutputConfiguration.Destination.valueOf(destination_);
return result == null ? polyglot.ConfigProto.OutputConfiguration.Destination.UNRECOGNIZED : result;
}
public static final int FILE_PATH_FIELD_NUMBER = 2;
private volatile java.lang.Object filePath_;
/**
*
* When using a destination of type FILE, indicates which file to write to.
*
*
* string file_path = 2;
* @return The filePath.
*/
@java.lang.Override
public java.lang.String getFilePath() {
java.lang.Object ref = filePath_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
filePath_ = s;
return s;
}
}
/**
*
* When using a destination of type FILE, indicates which file to write to.
*
*
* string file_path = 2;
* @return The bytes for filePath.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getFilePathBytes() {
java.lang.Object ref = filePath_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
filePath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (destination_ != polyglot.ConfigProto.OutputConfiguration.Destination.STDOUT.getNumber()) {
output.writeEnum(1, destination_);
}
if (!getFilePathBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, filePath_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (destination_ != polyglot.ConfigProto.OutputConfiguration.Destination.STDOUT.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, destination_);
}
if (!getFilePathBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(2, filePath_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof polyglot.ConfigProto.OutputConfiguration)) {
return super.equals(obj);
}
polyglot.ConfigProto.OutputConfiguration other = (polyglot.ConfigProto.OutputConfiguration) obj;
if (destination_ != other.destination_) return false;
if (!getFilePath()
.equals(other.getFilePath())) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + DESTINATION_FIELD_NUMBER;
hash = (53 * hash) + destination_;
hash = (37 * hash) + FILE_PATH_FIELD_NUMBER;
hash = (53 * hash) + getFilePath().hashCode();
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static polyglot.ConfigProto.OutputConfiguration parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.OutputConfiguration parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.OutputConfiguration parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.OutputConfiguration parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.OutputConfiguration parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.OutputConfiguration parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.OutputConfiguration parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.OutputConfiguration parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static polyglot.ConfigProto.OutputConfiguration parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.OutputConfiguration parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static polyglot.ConfigProto.OutputConfiguration parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.OutputConfiguration parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(polyglot.ConfigProto.OutputConfiguration prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Contains parameters controlling the output protos of polyglot.
*
*
* Protobuf type {@code polyglot.OutputConfiguration}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:polyglot.OutputConfiguration)
polyglot.ConfigProto.OutputConfigurationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return polyglot.ConfigProto.internal_static_polyglot_OutputConfiguration_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return polyglot.ConfigProto.internal_static_polyglot_OutputConfiguration_fieldAccessorTable
.ensureFieldAccessorsInitialized(
polyglot.ConfigProto.OutputConfiguration.class, polyglot.ConfigProto.OutputConfiguration.Builder.class);
}
// Construct using polyglot.ConfigProto.OutputConfiguration.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
destination_ = 0;
filePath_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return polyglot.ConfigProto.internal_static_polyglot_OutputConfiguration_descriptor;
}
@java.lang.Override
public polyglot.ConfigProto.OutputConfiguration getDefaultInstanceForType() {
return polyglot.ConfigProto.OutputConfiguration.getDefaultInstance();
}
@java.lang.Override
public polyglot.ConfigProto.OutputConfiguration build() {
polyglot.ConfigProto.OutputConfiguration result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public polyglot.ConfigProto.OutputConfiguration buildPartial() {
polyglot.ConfigProto.OutputConfiguration result = new polyglot.ConfigProto.OutputConfiguration(this);
result.destination_ = destination_;
result.filePath_ = filePath_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof polyglot.ConfigProto.OutputConfiguration) {
return mergeFrom((polyglot.ConfigProto.OutputConfiguration)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(polyglot.ConfigProto.OutputConfiguration other) {
if (other == polyglot.ConfigProto.OutputConfiguration.getDefaultInstance()) return this;
if (other.destination_ != 0) {
setDestinationValue(other.getDestinationValue());
}
if (!other.getFilePath().isEmpty()) {
filePath_ = other.filePath_;
onChanged();
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
polyglot.ConfigProto.OutputConfiguration parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (polyglot.ConfigProto.OutputConfiguration) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int destination_ = 0;
/**
* .polyglot.OutputConfiguration.Destination destination = 1;
* @return The enum numeric value on the wire for destination.
*/
@java.lang.Override public int getDestinationValue() {
return destination_;
}
/**
* .polyglot.OutputConfiguration.Destination destination = 1;
* @param value The enum numeric value on the wire for destination to set.
* @return This builder for chaining.
*/
public Builder setDestinationValue(int value) {
destination_ = value;
onChanged();
return this;
}
/**
* .polyglot.OutputConfiguration.Destination destination = 1;
* @return The destination.
*/
@java.lang.Override
public polyglot.ConfigProto.OutputConfiguration.Destination getDestination() {
@SuppressWarnings("deprecation")
polyglot.ConfigProto.OutputConfiguration.Destination result = polyglot.ConfigProto.OutputConfiguration.Destination.valueOf(destination_);
return result == null ? polyglot.ConfigProto.OutputConfiguration.Destination.UNRECOGNIZED : result;
}
/**
* .polyglot.OutputConfiguration.Destination destination = 1;
* @param value The destination to set.
* @return This builder for chaining.
*/
public Builder setDestination(polyglot.ConfigProto.OutputConfiguration.Destination value) {
if (value == null) {
throw new NullPointerException();
}
destination_ = value.getNumber();
onChanged();
return this;
}
/**
* .polyglot.OutputConfiguration.Destination destination = 1;
* @return This builder for chaining.
*/
public Builder clearDestination() {
destination_ = 0;
onChanged();
return this;
}
private java.lang.Object filePath_ = "";
/**
*
* When using a destination of type FILE, indicates which file to write to.
*
*
* string file_path = 2;
* @return The filePath.
*/
public java.lang.String getFilePath() {
java.lang.Object ref = filePath_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
filePath_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* When using a destination of type FILE, indicates which file to write to.
*
*
* string file_path = 2;
* @return The bytes for filePath.
*/
public com.google.protobuf.ByteString
getFilePathBytes() {
java.lang.Object ref = filePath_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
filePath_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* When using a destination of type FILE, indicates which file to write to.
*
*
* string file_path = 2;
* @param value The filePath to set.
* @return This builder for chaining.
*/
public Builder setFilePath(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
filePath_ = value;
onChanged();
return this;
}
/**
*
* When using a destination of type FILE, indicates which file to write to.
*
*
* string file_path = 2;
* @return This builder for chaining.
*/
public Builder clearFilePath() {
filePath_ = getDefaultInstance().getFilePath();
onChanged();
return this;
}
/**
*
* When using a destination of type FILE, indicates which file to write to.
*
*
* string file_path = 2;
* @param value The bytes for filePath to set.
* @return This builder for chaining.
*/
public Builder setFilePathBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
filePath_ = value;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:polyglot.OutputConfiguration)
}
// @@protoc_insertion_point(class_scope:polyglot.OutputConfiguration)
private static final polyglot.ConfigProto.OutputConfiguration DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new polyglot.ConfigProto.OutputConfiguration();
}
public static polyglot.ConfigProto.OutputConfiguration getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public OutputConfiguration parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new OutputConfiguration(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public polyglot.ConfigProto.OutputConfiguration getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface ProtoConfigurationOrBuilder extends
// @@protoc_insertion_point(interface_extends:polyglot.ProtoConfiguration)
com.google.protobuf.MessageOrBuilder {
/**
*
* A root directory to scan for .proto files. All files found this way will
* be analyzed for service definitions by invoking protoc.
*
*
* string proto_discovery_root = 1;
* @return The protoDiscoveryRoot.
*/
java.lang.String getProtoDiscoveryRoot();
/**
*
* A root directory to scan for .proto files. All files found this way will
* be analyzed for service definitions by invoking protoc.
*
*
* string proto_discovery_root = 1;
* @return The bytes for protoDiscoveryRoot.
*/
com.google.protobuf.ByteString
getProtoDiscoveryRootBytes();
/**
*
* Include paths used to resolve imports of the files being analyzed when
* resolving service definitions using protoc.
*
*
* repeated string include_paths = 2;
* @return A list containing the includePaths.
*/
java.util.List
getIncludePathsList();
/**
*
* Include paths used to resolve imports of the files being analyzed when
* resolving service definitions using protoc.
*
*
* repeated string include_paths = 2;
* @return The count of includePaths.
*/
int getIncludePathsCount();
/**
*
* Include paths used to resolve imports of the files being analyzed when
* resolving service definitions using protoc.
*
*
* repeated string include_paths = 2;
* @param index The index of the element to return.
* @return The includePaths at the given index.
*/
java.lang.String getIncludePaths(int index);
/**
*
* Include paths used to resolve imports of the files being analyzed when
* resolving service definitions using protoc.
*
*
* repeated string include_paths = 2;
* @param index The index of the value to return.
* @return The bytes of the includePaths at the given index.
*/
com.google.protobuf.ByteString
getIncludePathsBytes(int index);
/**
*
* If true, protos will first be resolved by reflection if applicable.
*
*
* bool use_reflection = 3;
* @return The useReflection.
*/
boolean getUseReflection();
}
/**
*
* Contains the necessary information to locate .proto files for services.
*
*
* Protobuf type {@code polyglot.ProtoConfiguration}
*/
public static final class ProtoConfiguration extends
com.google.protobuf.GeneratedMessageV3 implements
// @@protoc_insertion_point(message_implements:polyglot.ProtoConfiguration)
ProtoConfigurationOrBuilder {
private static final long serialVersionUID = 0L;
// Use ProtoConfiguration.newBuilder() to construct.
private ProtoConfiguration(com.google.protobuf.GeneratedMessageV3.Builder> builder) {
super(builder);
}
private ProtoConfiguration() {
protoDiscoveryRoot_ = "";
includePaths_ = com.google.protobuf.LazyStringArrayList.EMPTY;
}
@java.lang.Override
@SuppressWarnings({"unused"})
protected java.lang.Object newInstance(
UnusedPrivateParameter unused) {
return new ProtoConfiguration();
}
@java.lang.Override
public final com.google.protobuf.UnknownFieldSet
getUnknownFields() {
return this.unknownFields;
}
private ProtoConfiguration(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
this();
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
int mutable_bitField0_ = 0;
com.google.protobuf.UnknownFieldSet.Builder unknownFields =
com.google.protobuf.UnknownFieldSet.newBuilder();
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
protoDiscoveryRoot_ = s;
break;
}
case 18: {
java.lang.String s = input.readStringRequireUtf8();
if (!((mutable_bitField0_ & 0x00000001) != 0)) {
includePaths_ = new com.google.protobuf.LazyStringArrayList();
mutable_bitField0_ |= 0x00000001;
}
includePaths_.add(s);
break;
}
case 24: {
useReflection_ = input.readBool();
break;
}
default: {
if (!parseUnknownField(
input, unknownFields, extensionRegistry, tag)) {
done = true;
}
break;
}
}
}
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(this);
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(
e).setUnfinishedMessage(this);
} finally {
if (((mutable_bitField0_ & 0x00000001) != 0)) {
includePaths_ = includePaths_.getUnmodifiableView();
}
this.unknownFields = unknownFields.build();
makeExtensionsImmutable();
}
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return polyglot.ConfigProto.internal_static_polyglot_ProtoConfiguration_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return polyglot.ConfigProto.internal_static_polyglot_ProtoConfiguration_fieldAccessorTable
.ensureFieldAccessorsInitialized(
polyglot.ConfigProto.ProtoConfiguration.class, polyglot.ConfigProto.ProtoConfiguration.Builder.class);
}
public static final int PROTO_DISCOVERY_ROOT_FIELD_NUMBER = 1;
private volatile java.lang.Object protoDiscoveryRoot_;
/**
*
* A root directory to scan for .proto files. All files found this way will
* be analyzed for service definitions by invoking protoc.
*
*
* string proto_discovery_root = 1;
* @return The protoDiscoveryRoot.
*/
@java.lang.Override
public java.lang.String getProtoDiscoveryRoot() {
java.lang.Object ref = protoDiscoveryRoot_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
protoDiscoveryRoot_ = s;
return s;
}
}
/**
*
* A root directory to scan for .proto files. All files found this way will
* be analyzed for service definitions by invoking protoc.
*
*
* string proto_discovery_root = 1;
* @return The bytes for protoDiscoveryRoot.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getProtoDiscoveryRootBytes() {
java.lang.Object ref = protoDiscoveryRoot_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
protoDiscoveryRoot_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int INCLUDE_PATHS_FIELD_NUMBER = 2;
private com.google.protobuf.LazyStringList includePaths_;
/**
*
* Include paths used to resolve imports of the files being analyzed when
* resolving service definitions using protoc.
*
*
* repeated string include_paths = 2;
* @return A list containing the includePaths.
*/
public com.google.protobuf.ProtocolStringList
getIncludePathsList() {
return includePaths_;
}
/**
*
* Include paths used to resolve imports of the files being analyzed when
* resolving service definitions using protoc.
*
*
* repeated string include_paths = 2;
* @return The count of includePaths.
*/
public int getIncludePathsCount() {
return includePaths_.size();
}
/**
*
* Include paths used to resolve imports of the files being analyzed when
* resolving service definitions using protoc.
*
*
* repeated string include_paths = 2;
* @param index The index of the element to return.
* @return The includePaths at the given index.
*/
public java.lang.String getIncludePaths(int index) {
return includePaths_.get(index);
}
/**
*
* Include paths used to resolve imports of the files being analyzed when
* resolving service definitions using protoc.
*
*
* repeated string include_paths = 2;
* @param index The index of the value to return.
* @return The bytes of the includePaths at the given index.
*/
public com.google.protobuf.ByteString
getIncludePathsBytes(int index) {
return includePaths_.getByteString(index);
}
public static final int USE_REFLECTION_FIELD_NUMBER = 3;
private boolean useReflection_;
/**
*
* If true, protos will first be resolved by reflection if applicable.
*
*
* bool use_reflection = 3;
* @return The useReflection.
*/
@java.lang.Override
public boolean getUseReflection() {
return useReflection_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!getProtoDiscoveryRootBytes().isEmpty()) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 1, protoDiscoveryRoot_);
}
for (int i = 0; i < includePaths_.size(); i++) {
com.google.protobuf.GeneratedMessageV3.writeString(output, 2, includePaths_.getRaw(i));
}
if (useReflection_ != false) {
output.writeBool(3, useReflection_);
}
unknownFields.writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!getProtoDiscoveryRootBytes().isEmpty()) {
size += com.google.protobuf.GeneratedMessageV3.computeStringSize(1, protoDiscoveryRoot_);
}
{
int dataSize = 0;
for (int i = 0; i < includePaths_.size(); i++) {
dataSize += computeStringSizeNoTag(includePaths_.getRaw(i));
}
size += dataSize;
size += 1 * getIncludePathsList().size();
}
if (useReflection_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(3, useReflection_);
}
size += unknownFields.getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof polyglot.ConfigProto.ProtoConfiguration)) {
return super.equals(obj);
}
polyglot.ConfigProto.ProtoConfiguration other = (polyglot.ConfigProto.ProtoConfiguration) obj;
if (!getProtoDiscoveryRoot()
.equals(other.getProtoDiscoveryRoot())) return false;
if (!getIncludePathsList()
.equals(other.getIncludePathsList())) return false;
if (getUseReflection()
!= other.getUseReflection()) return false;
if (!unknownFields.equals(other.unknownFields)) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PROTO_DISCOVERY_ROOT_FIELD_NUMBER;
hash = (53 * hash) + getProtoDiscoveryRoot().hashCode();
if (getIncludePathsCount() > 0) {
hash = (37 * hash) + INCLUDE_PATHS_FIELD_NUMBER;
hash = (53 * hash) + getIncludePathsList().hashCode();
}
hash = (37 * hash) + USE_REFLECTION_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUseReflection());
hash = (29 * hash) + unknownFields.hashCode();
memoizedHashCode = hash;
return hash;
}
public static polyglot.ConfigProto.ProtoConfiguration parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.ProtoConfiguration parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.ProtoConfiguration parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.ProtoConfiguration parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.ProtoConfiguration parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static polyglot.ConfigProto.ProtoConfiguration parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static polyglot.ConfigProto.ProtoConfiguration parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.ProtoConfiguration parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static polyglot.ConfigProto.ProtoConfiguration parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.ProtoConfiguration parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static polyglot.ConfigProto.ProtoConfiguration parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input);
}
public static polyglot.ConfigProto.ProtoConfiguration parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessageV3
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(polyglot.ConfigProto.ProtoConfiguration prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* Contains the necessary information to locate .proto files for services.
*
*
* Protobuf type {@code polyglot.ProtoConfiguration}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessageV3.Builder implements
// @@protoc_insertion_point(builder_implements:polyglot.ProtoConfiguration)
polyglot.ConfigProto.ProtoConfigurationOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return polyglot.ConfigProto.internal_static_polyglot_ProtoConfiguration_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internalGetFieldAccessorTable() {
return polyglot.ConfigProto.internal_static_polyglot_ProtoConfiguration_fieldAccessorTable
.ensureFieldAccessorsInitialized(
polyglot.ConfigProto.ProtoConfiguration.class, polyglot.ConfigProto.ProtoConfiguration.Builder.class);
}
// Construct using polyglot.ConfigProto.ProtoConfiguration.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessageV3.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessageV3
.alwaysUseFieldBuilders) {
}
}
@java.lang.Override
public Builder clear() {
super.clear();
protoDiscoveryRoot_ = "";
includePaths_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
useReflection_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return polyglot.ConfigProto.internal_static_polyglot_ProtoConfiguration_descriptor;
}
@java.lang.Override
public polyglot.ConfigProto.ProtoConfiguration getDefaultInstanceForType() {
return polyglot.ConfigProto.ProtoConfiguration.getDefaultInstance();
}
@java.lang.Override
public polyglot.ConfigProto.ProtoConfiguration build() {
polyglot.ConfigProto.ProtoConfiguration result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public polyglot.ConfigProto.ProtoConfiguration buildPartial() {
polyglot.ConfigProto.ProtoConfiguration result = new polyglot.ConfigProto.ProtoConfiguration(this);
int from_bitField0_ = bitField0_;
result.protoDiscoveryRoot_ = protoDiscoveryRoot_;
if (((bitField0_ & 0x00000001) != 0)) {
includePaths_ = includePaths_.getUnmodifiableView();
bitField0_ = (bitField0_ & ~0x00000001);
}
result.includePaths_ = includePaths_;
result.useReflection_ = useReflection_;
onBuilt();
return result;
}
@java.lang.Override
public Builder clone() {
return super.clone();
}
@java.lang.Override
public Builder setField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.setField(field, value);
}
@java.lang.Override
public Builder clearField(
com.google.protobuf.Descriptors.FieldDescriptor field) {
return super.clearField(field);
}
@java.lang.Override
public Builder clearOneof(
com.google.protobuf.Descriptors.OneofDescriptor oneof) {
return super.clearOneof(oneof);
}
@java.lang.Override
public Builder setRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
int index, java.lang.Object value) {
return super.setRepeatedField(field, index, value);
}
@java.lang.Override
public Builder addRepeatedField(
com.google.protobuf.Descriptors.FieldDescriptor field,
java.lang.Object value) {
return super.addRepeatedField(field, value);
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof polyglot.ConfigProto.ProtoConfiguration) {
return mergeFrom((polyglot.ConfigProto.ProtoConfiguration)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(polyglot.ConfigProto.ProtoConfiguration other) {
if (other == polyglot.ConfigProto.ProtoConfiguration.getDefaultInstance()) return this;
if (!other.getProtoDiscoveryRoot().isEmpty()) {
protoDiscoveryRoot_ = other.protoDiscoveryRoot_;
onChanged();
}
if (!other.includePaths_.isEmpty()) {
if (includePaths_.isEmpty()) {
includePaths_ = other.includePaths_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureIncludePathsIsMutable();
includePaths_.addAll(other.includePaths_);
}
onChanged();
}
if (other.getUseReflection() != false) {
setUseReflection(other.getUseReflection());
}
this.mergeUnknownFields(other.unknownFields);
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
polyglot.ConfigProto.ProtoConfiguration parsedMessage = null;
try {
parsedMessage = PARSER.parsePartialFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
parsedMessage = (polyglot.ConfigProto.ProtoConfiguration) e.getUnfinishedMessage();
throw e.unwrapIOException();
} finally {
if (parsedMessage != null) {
mergeFrom(parsedMessage);
}
}
return this;
}
private int bitField0_;
private java.lang.Object protoDiscoveryRoot_ = "";
/**
*
* A root directory to scan for .proto files. All files found this way will
* be analyzed for service definitions by invoking protoc.
*
*
* string proto_discovery_root = 1;
* @return The protoDiscoveryRoot.
*/
public java.lang.String getProtoDiscoveryRoot() {
java.lang.Object ref = protoDiscoveryRoot_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
protoDiscoveryRoot_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* A root directory to scan for .proto files. All files found this way will
* be analyzed for service definitions by invoking protoc.
*
*
* string proto_discovery_root = 1;
* @return The bytes for protoDiscoveryRoot.
*/
public com.google.protobuf.ByteString
getProtoDiscoveryRootBytes() {
java.lang.Object ref = protoDiscoveryRoot_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
protoDiscoveryRoot_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* A root directory to scan for .proto files. All files found this way will
* be analyzed for service definitions by invoking protoc.
*
*
* string proto_discovery_root = 1;
* @param value The protoDiscoveryRoot to set.
* @return This builder for chaining.
*/
public Builder setProtoDiscoveryRoot(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
protoDiscoveryRoot_ = value;
onChanged();
return this;
}
/**
*
* A root directory to scan for .proto files. All files found this way will
* be analyzed for service definitions by invoking protoc.
*
*
* string proto_discovery_root = 1;
* @return This builder for chaining.
*/
public Builder clearProtoDiscoveryRoot() {
protoDiscoveryRoot_ = getDefaultInstance().getProtoDiscoveryRoot();
onChanged();
return this;
}
/**
*
* A root directory to scan for .proto files. All files found this way will
* be analyzed for service definitions by invoking protoc.
*
*
* string proto_discovery_root = 1;
* @param value The bytes for protoDiscoveryRoot to set.
* @return This builder for chaining.
*/
public Builder setProtoDiscoveryRootBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
protoDiscoveryRoot_ = value;
onChanged();
return this;
}
private com.google.protobuf.LazyStringList includePaths_ = com.google.protobuf.LazyStringArrayList.EMPTY;
private void ensureIncludePathsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
includePaths_ = new com.google.protobuf.LazyStringArrayList(includePaths_);
bitField0_ |= 0x00000001;
}
}
/**
*
* Include paths used to resolve imports of the files being analyzed when
* resolving service definitions using protoc.
*
*
* repeated string include_paths = 2;
* @return A list containing the includePaths.
*/
public com.google.protobuf.ProtocolStringList
getIncludePathsList() {
return includePaths_.getUnmodifiableView();
}
/**
*
* Include paths used to resolve imports of the files being analyzed when
* resolving service definitions using protoc.
*
*
* repeated string include_paths = 2;
* @return The count of includePaths.
*/
public int getIncludePathsCount() {
return includePaths_.size();
}
/**
*
* Include paths used to resolve imports of the files being analyzed when
* resolving service definitions using protoc.
*
*
* repeated string include_paths = 2;
* @param index The index of the element to return.
* @return The includePaths at the given index.
*/
public java.lang.String getIncludePaths(int index) {
return includePaths_.get(index);
}
/**
*
* Include paths used to resolve imports of the files being analyzed when
* resolving service definitions using protoc.
*
*
* repeated string include_paths = 2;
* @param index The index of the value to return.
* @return The bytes of the includePaths at the given index.
*/
public com.google.protobuf.ByteString
getIncludePathsBytes(int index) {
return includePaths_.getByteString(index);
}
/**
*
* Include paths used to resolve imports of the files being analyzed when
* resolving service definitions using protoc.
*
*
* repeated string include_paths = 2;
* @param index The index to set the value at.
* @param value The includePaths to set.
* @return This builder for chaining.
*/
public Builder setIncludePaths(
int index, java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureIncludePathsIsMutable();
includePaths_.set(index, value);
onChanged();
return this;
}
/**
*
* Include paths used to resolve imports of the files being analyzed when
* resolving service definitions using protoc.
*
*
* repeated string include_paths = 2;
* @param value The includePaths to add.
* @return This builder for chaining.
*/
public Builder addIncludePaths(
java.lang.String value) {
if (value == null) {
throw new NullPointerException();
}
ensureIncludePathsIsMutable();
includePaths_.add(value);
onChanged();
return this;
}
/**
*
* Include paths used to resolve imports of the files being analyzed when
* resolving service definitions using protoc.
*
*
* repeated string include_paths = 2;
* @param values The includePaths to add.
* @return This builder for chaining.
*/
public Builder addAllIncludePaths(
java.lang.Iterable values) {
ensureIncludePathsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, includePaths_);
onChanged();
return this;
}
/**
*
* Include paths used to resolve imports of the files being analyzed when
* resolving service definitions using protoc.
*
*
* repeated string include_paths = 2;
* @return This builder for chaining.
*/
public Builder clearIncludePaths() {
includePaths_ = com.google.protobuf.LazyStringArrayList.EMPTY;
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* Include paths used to resolve imports of the files being analyzed when
* resolving service definitions using protoc.
*
*
* repeated string include_paths = 2;
* @param value The bytes of the includePaths to add.
* @return This builder for chaining.
*/
public Builder addIncludePathsBytes(
com.google.protobuf.ByteString value) {
if (value == null) {
throw new NullPointerException();
}
checkByteStringIsUtf8(value);
ensureIncludePathsIsMutable();
includePaths_.add(value);
onChanged();
return this;
}
private boolean useReflection_ ;
/**
*
* If true, protos will first be resolved by reflection if applicable.
*
*
* bool use_reflection = 3;
* @return The useReflection.
*/
@java.lang.Override
public boolean getUseReflection() {
return useReflection_;
}
/**
*
* If true, protos will first be resolved by reflection if applicable.
*
*
* bool use_reflection = 3;
* @param value The useReflection to set.
* @return This builder for chaining.
*/
public Builder setUseReflection(boolean value) {
useReflection_ = value;
onChanged();
return this;
}
/**
*
* If true, protos will first be resolved by reflection if applicable.
*
*
* bool use_reflection = 3;
* @return This builder for chaining.
*/
public Builder clearUseReflection() {
useReflection_ = false;
onChanged();
return this;
}
@java.lang.Override
public final Builder setUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.setUnknownFields(unknownFields);
}
@java.lang.Override
public final Builder mergeUnknownFields(
final com.google.protobuf.UnknownFieldSet unknownFields) {
return super.mergeUnknownFields(unknownFields);
}
// @@protoc_insertion_point(builder_scope:polyglot.ProtoConfiguration)
}
// @@protoc_insertion_point(class_scope:polyglot.ProtoConfiguration)
private static final polyglot.ConfigProto.ProtoConfiguration DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new polyglot.ConfigProto.ProtoConfiguration();
}
public static polyglot.ConfigProto.ProtoConfiguration getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public ProtoConfiguration parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return new ProtoConfiguration(input, extensionRegistry);
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public polyglot.ConfigProto.ProtoConfiguration getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_polyglot_ConfigurationSet_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_polyglot_ConfigurationSet_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_polyglot_Configuration_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_polyglot_Configuration_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_polyglot_CallConfiguration_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_polyglot_CallConfiguration_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_polyglot_CallMetadataEntry_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_polyglot_CallMetadataEntry_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_polyglot_OauthConfiguration_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_polyglot_OauthConfiguration_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_polyglot_OauthConfiguration_RefreshTokenCredentials_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_polyglot_OauthConfiguration_RefreshTokenCredentials_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_polyglot_OauthConfiguration_AccessTokenCredentials_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_polyglot_OauthConfiguration_AccessTokenCredentials_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_polyglot_OauthConfiguration_OauthClient_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_polyglot_OauthConfiguration_OauthClient_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_polyglot_OutputConfiguration_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_polyglot_OutputConfiguration_fieldAccessorTable;
private static final com.google.protobuf.Descriptors.Descriptor
internal_static_polyglot_ProtoConfiguration_descriptor;
private static final
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable
internal_static_polyglot_ProtoConfiguration_fieldAccessorTable;
public static com.google.protobuf.Descriptors.FileDescriptor
getDescriptor() {
return descriptor;
}
private static com.google.protobuf.Descriptors.FileDescriptor
descriptor;
static {
java.lang.String[] descriptorData = {
"\n\014config.proto\022\010polyglot\"C\n\020Configuratio" +
"nSet\022/\n\016configurations\030\001 \003(\0132\027.polyglot." +
"Configuration\"\271\001\n\rConfiguration\022\014\n\004name\030" +
"\001 \001(\t\0220\n\013call_config\030\002 \001(\0132\033.polyglot.Ca" +
"llConfiguration\0222\n\014proto_config\030\003 \001(\0132\034." +
"polyglot.ProtoConfiguration\0224\n\routput_co" +
"nfig\030\004 \001(\0132\035.polyglot.OutputConfiguratio" +
"n\"\230\002\n\021CallConfiguration\022\023\n\013deadline_ms\030\001" +
" \001(\r\022\017\n\007use_tls\030\002 \001(\010\0222\n\014oauth_config\030\003 " +
"\001(\0132\034.polyglot.OauthConfiguration\022\030\n\020tls" +
"_ca_cert_path\030\004 \001(\t\022\034\n\024tls_client_cert_p" +
"ath\030\005 \001(\t\022\033\n\023tls_client_key_path\030\006 \001(\t\022%" +
"\n\035tls_client_override_authority\030\007 \001(\t\022-\n" +
"\010metadata\030\010 \003(\0132\033.polyglot.CallMetadataE" +
"ntry\"0\n\021CallMetadataEntry\022\014\n\004name\030\001 \001(\t\022" +
"\r\n\005value\030\002 \001(\t\"\305\003\n\022OauthConfiguration\022Y\n" +
"\031refresh_token_credentials\030\001 \001(\01324.polyg" +
"lot.OauthConfiguration.RefreshTokenCrede" +
"ntialsH\000\022W\n\030access_token_credentials\030\002 \001" +
"(\01323.polyglot.OauthConfiguration.AccessT" +
"okenCredentialsH\000\032\213\001\n\027RefreshTokenCreden" +
"tials\022\032\n\022token_endpoint_url\030\001 \001(\t\0228\n\006cli" +
"ent\030\002 \001(\0132(.polyglot.OauthConfiguration." +
"OauthClient\022\032\n\022refresh_token_path\030\003 \001(\t\032" +
"3\n\026AccessTokenCredentials\022\031\n\021access_toke" +
"n_path\030\001 \001(\t\032)\n\013OauthClient\022\n\n\002id\030\001 \001(\t\022" +
"\016\n\006secret\030\002 \001(\tB\r\n\013credentials\"\226\001\n\023Outpu" +
"tConfiguration\022>\n\013destination\030\001 \001(\0162).po" +
"lyglot.OutputConfiguration.Destination\022\021" +
"\n\tfile_path\030\002 \001(\t\",\n\013Destination\022\n\n\006STDO" +
"UT\020\000\022\007\n\003LOG\020\001\022\010\n\004FILE\020\002\"a\n\022ProtoConfigur" +
"ation\022\034\n\024proto_discovery_root\030\001 \001(\t\022\025\n\ri" +
"nclude_paths\030\002 \003(\t\022\026\n\016use_reflection\030\003 \001" +
"(\010B\rB\013ConfigProtob\006proto3"
};
descriptor = com.google.protobuf.Descriptors.FileDescriptor
.internalBuildGeneratedFileFrom(descriptorData,
new com.google.protobuf.Descriptors.FileDescriptor[] {
});
internal_static_polyglot_ConfigurationSet_descriptor =
getDescriptor().getMessageTypes().get(0);
internal_static_polyglot_ConfigurationSet_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_polyglot_ConfigurationSet_descriptor,
new java.lang.String[] { "Configurations", });
internal_static_polyglot_Configuration_descriptor =
getDescriptor().getMessageTypes().get(1);
internal_static_polyglot_Configuration_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_polyglot_Configuration_descriptor,
new java.lang.String[] { "Name", "CallConfig", "ProtoConfig", "OutputConfig", });
internal_static_polyglot_CallConfiguration_descriptor =
getDescriptor().getMessageTypes().get(2);
internal_static_polyglot_CallConfiguration_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_polyglot_CallConfiguration_descriptor,
new java.lang.String[] { "DeadlineMs", "UseTls", "OauthConfig", "TlsCaCertPath", "TlsClientCertPath", "TlsClientKeyPath", "TlsClientOverrideAuthority", "Metadata", });
internal_static_polyglot_CallMetadataEntry_descriptor =
getDescriptor().getMessageTypes().get(3);
internal_static_polyglot_CallMetadataEntry_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_polyglot_CallMetadataEntry_descriptor,
new java.lang.String[] { "Name", "Value", });
internal_static_polyglot_OauthConfiguration_descriptor =
getDescriptor().getMessageTypes().get(4);
internal_static_polyglot_OauthConfiguration_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_polyglot_OauthConfiguration_descriptor,
new java.lang.String[] { "RefreshTokenCredentials", "AccessTokenCredentials", "Credentials", });
internal_static_polyglot_OauthConfiguration_RefreshTokenCredentials_descriptor =
internal_static_polyglot_OauthConfiguration_descriptor.getNestedTypes().get(0);
internal_static_polyglot_OauthConfiguration_RefreshTokenCredentials_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_polyglot_OauthConfiguration_RefreshTokenCredentials_descriptor,
new java.lang.String[] { "TokenEndpointUrl", "Client", "RefreshTokenPath", });
internal_static_polyglot_OauthConfiguration_AccessTokenCredentials_descriptor =
internal_static_polyglot_OauthConfiguration_descriptor.getNestedTypes().get(1);
internal_static_polyglot_OauthConfiguration_AccessTokenCredentials_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_polyglot_OauthConfiguration_AccessTokenCredentials_descriptor,
new java.lang.String[] { "AccessTokenPath", });
internal_static_polyglot_OauthConfiguration_OauthClient_descriptor =
internal_static_polyglot_OauthConfiguration_descriptor.getNestedTypes().get(2);
internal_static_polyglot_OauthConfiguration_OauthClient_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_polyglot_OauthConfiguration_OauthClient_descriptor,
new java.lang.String[] { "Id", "Secret", });
internal_static_polyglot_OutputConfiguration_descriptor =
getDescriptor().getMessageTypes().get(5);
internal_static_polyglot_OutputConfiguration_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_polyglot_OutputConfiguration_descriptor,
new java.lang.String[] { "Destination", "FilePath", });
internal_static_polyglot_ProtoConfiguration_descriptor =
getDescriptor().getMessageTypes().get(6);
internal_static_polyglot_ProtoConfiguration_fieldAccessorTable = new
com.google.protobuf.GeneratedMessageV3.FieldAccessorTable(
internal_static_polyglot_ProtoConfiguration_descriptor,
new java.lang.String[] { "ProtoDiscoveryRoot", "IncludePaths", "UseReflection", });
}
// @@protoc_insertion_point(outer_class_scope)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy