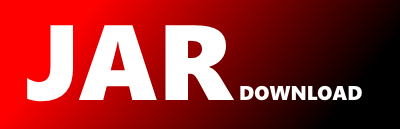
com.qozix.layouts.FixedLayout Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of TileView Show documentation
Show all versions of TileView Show documentation
The TileView widget is a subclass of ViewGroup that provides a mechanism to asynchronously display tile-based images, with additional functionality for 2D dragging, flinging, pinch or double-tap to zoom, adding overlaying Views (markers), built-in Hot Spot support, dynamic path drawing, multiple levels of detail, and support for any relative positioning or coordinate system.
The newest version!
package com.qozix.layouts;
import android.content.Context;
import android.view.View;
import android.view.ViewGroup;
public class FixedLayout extends ViewGroup {
public FixedLayout(Context context) {
super(context);
}
@Override
protected void onMeasure(int widthMeasureSpec, int heightMeasureSpec) {
measureChildren(widthMeasureSpec, heightMeasureSpec);
int w = 0;
int h = 0;
int count = getChildCount();
for (int i = 0; i < count; i++) {
View child = getChildAt(i);
if (child.getVisibility() != GONE) {
FixedLayout.LayoutParams lp = (FixedLayout.LayoutParams) child.getLayoutParams();
int right = lp.x + child.getMeasuredWidth();
int bottom = lp.y + child.getMeasuredHeight();
w = Math.max(w, right);
h = Math.max(h, bottom);
}
}
h = Math.max(h, getSuggestedMinimumHeight());
w = Math.max(w, getSuggestedMinimumWidth());
w = resolveSize(w, widthMeasureSpec);
h = resolveSize(h, heightMeasureSpec);
setMeasuredDimension(w, h);
}
@Override
protected ViewGroup.LayoutParams generateDefaultLayoutParams() {
return new LayoutParams(LayoutParams.WRAP_CONTENT, LayoutParams.WRAP_CONTENT, 0, 0);
}
@Override
protected void onLayout(boolean changed, int l, int t, int r, int b) {
int count = getChildCount();
for (int i = 0; i < count; i++) {
View child = getChildAt(i);
if (child.getVisibility() != GONE) {
FixedLayout.LayoutParams lp = (FixedLayout.LayoutParams) child.getLayoutParams();
child.layout(lp.x, lp.y, lp.x + child.getMeasuredWidth(), lp.y + child.getMeasuredHeight());
}
}
}
@Override
protected boolean checkLayoutParams(ViewGroup.LayoutParams p) {
return p instanceof FixedLayout.LayoutParams;
}
@Override
protected ViewGroup.LayoutParams generateLayoutParams(ViewGroup.LayoutParams p) {
return new LayoutParams(p);
}
public static class LayoutParams extends ViewGroup.LayoutParams {
public int x = 0;
public int y = 0;
public LayoutParams(int width, int height, int left, int top) {
super(width, height);
x = left;
y = top;
}
public LayoutParams(int width, int height){
super(width, height);
}
public LayoutParams(ViewGroup.LayoutParams source) {
super(source);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy