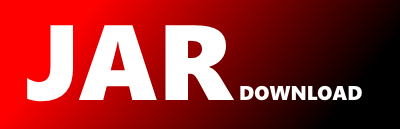
com.qozix.tileview.hotspots.HotSpotManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of TileView Show documentation
Show all versions of TileView Show documentation
The TileView widget is a subclass of ViewGroup that provides a mechanism to asynchronously display tile-based images, with additional functionality for 2D dragging, flinging, pinch or double-tap to zoom, adding overlaying Views (markers), built-in Hot Spot support, dynamic path drawing, multiple levels of detail, and support for any relative positioning or coordinate system.
The newest version!
package com.qozix.tileview.hotspots;
import java.util.ArrayList;
import java.util.LinkedList;
import com.qozix.tileview.detail.DetailLevelEventListener;
import com.qozix.tileview.detail.DetailManager;
import android.graphics.Point;
public class HotSpotManager implements DetailLevelEventListener {
private double scale = 1;
private ArrayList listeners = new ArrayList();
private ArrayList spots = new ArrayList();
public HotSpotManager( DetailManager detailManager ) {
detailManager.addDetailLevelEventListener( this );
}
public void addHotSpot( HotSpot hotSpot ){
spots.add( hotSpot );
}
public void removeHotSpot( HotSpot hotSpot ){
spots.remove( hotSpot );
}
public void addHotSpotEventListener( HotSpotEventListener listener ) {
listeners.add( listener );
}
public void removeHotSpotEventListener( HotSpotEventListener listener ) {
listeners.remove( listener );
}
public void clear(){
spots.clear();
}
// work from end of list - match the last one added (equivalant to z-index)
private HotSpot getMatch( Point point ){
Point scaledPoint = new Point();
scaledPoint.x = (int) ( point.x / scale );
scaledPoint.y = (int) ( point.y / scale );
for(int i = spots.size() - 1; i >= 0; i--){
HotSpot hotSpot = spots.get( i );
if(hotSpot.contains( scaledPoint.x, scaledPoint.y )){
return hotSpot;
}
}
return null;
}
public void processHit( Point point ){
// fast-fail if no listeners
if(listeners.isEmpty()){
return;
}
// is there a match?
HotSpot hotSpot = getMatch( point );
if( hotSpot != null){
HotSpotEventListener spotListener = hotSpot.getHotSpotEventListener();
if( spotListener != null ) {
spotListener.onHotSpotTap( hotSpot, point.x, point.y );
}
for( HotSpotEventListener listener : listeners ) {
listener.onHotSpotTap( hotSpot, point.x, point.y );
}
}
}
@Override
public void onDetailLevelChanged() {
}
@Override
public void onDetailScaleChanged( double s ) {
scale = s;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy