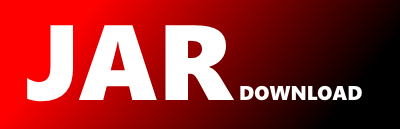
com.qozix.tileview.samples.SampleManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of TileView Show documentation
Show all versions of TileView Show documentation
The TileView widget is a subclass of ViewGroup that provides a mechanism to asynchronously display tile-based images, with additional functionality for 2D dragging, flinging, pinch or double-tap to zoom, adding overlaying Views (markers), built-in Hot Spot support, dynamic path drawing, multiple levels of detail, and support for any relative positioning or coordinate system.
The newest version!
package com.qozix.tileview.samples;
import android.content.Context;
import android.graphics.Bitmap;
import android.graphics.Canvas;
import android.graphics.Rect;
import android.view.View;
import com.qozix.tileview.detail.DetailLevel;
import com.qozix.tileview.detail.DetailLevelEventListener;
import com.qozix.tileview.detail.DetailManager;
import com.qozix.tileview.graphics.BitmapDecoder;
import com.qozix.tileview.graphics.BitmapDecoderAssets;
public class SampleManager extends View implements DetailLevelEventListener {
private DetailManager detailManager;
private BitmapDecoder decoder = new BitmapDecoderAssets();
private Rect area = new Rect(0, 0, 0, 0);
private Bitmap bitmap;
private String lastFileName;
public SampleManager( Context context, DetailManager dm ) {
super( context );
detailManager = dm;
detailManager.addDetailLevelEventListener( this );
update();
}
public void setDecoder( BitmapDecoder d ){
decoder = d;
}
public void clear(){
bitmap = null;
lastFileName = null;
}
public void update() {
DetailLevel detailLevel = detailManager.getCurrentDetailLevel();
if( detailLevel != null ) {
String fileName = detailLevel.getDownsample();
if( fileName != null ) {
if( !fileName.equals( lastFileName ) ) {
bitmap = decoder.decode( fileName, getContext() );
invalidate();
}
}
lastFileName = fileName;
}
}
@Override
public void onDetailLevelChanged() {
update();
}
@Override
public void onDetailScaleChanged( double s ) {
}
@Override
public void onDraw( Canvas canvas ) {
if( bitmap != null) {
area.right = getWidth();
area.bottom = getHeight();
canvas.drawBitmap( bitmap, null, area, null);
}
super.onDraw( canvas );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy