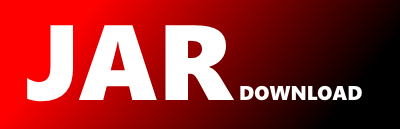
com.qozix.tileview.tiles.selector.TileSetSelectorByRange Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of TileView Show documentation
Show all versions of TileView Show documentation
The TileView widget is a subclass of ViewGroup that provides a mechanism to asynchronously display tile-based images, with additional functionality for 2D dragging, flinging, pinch or double-tap to zoom, adding overlaying Views (markers), built-in Hot Spot support, dynamic path drawing, multiple levels of detail, and support for any relative positioning or coordinate system.
The newest version!
package com.qozix.tileview.tiles.selector;
import java.util.ArrayList;
import java.util.List;
import com.qozix.tileview.detail.DetailLevel;
import com.qozix.tileview.detail.DetailLevelSet;
public class TileSetSelectorByRange implements TileSetSelector {
private List switchPoint = new ArrayList();
@Override
public DetailLevel find( double scale, DetailLevelSet levels ) {
int totalLevels = levels.size();
int totalSwitches = switchPoint.size();
// fast-fail
if ( totalLevels == 0 ) {
return null;
}
// sanity check the switchPoints with the levels
// switchPoints should be 1 less then the total levels
if ( totalLevels != ( totalSwitches + 1 ) ) {
return null;
}
// loop through and find a set where this scale fits
for ( int index = 0; index < totalSwitches; index++ ) {
double thisSwitchPoint = this.switchPoint.get( index );
// when we exceed the scale we take the previous
if ( scale < thisSwitchPoint ) {
return levels.get( index );
}
}
// take the last
return levels.get( totalLevels - 1 );
}
public void add( final double value ) {
switchPoint.add( value );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy