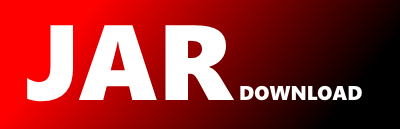
com.qspin.qtaste.javaguifx.server.TreeNodeSelector Maven / Gradle / Ivy
The newest version!
/*
Copyright 2007-2012 QSpin - www.qspin.be
This file is part of QTaste framework.
QTaste is free software: you can redistribute it and/or modify
it under the terms of the GNU Lesser General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
QTaste is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public License
along with QTaste. If not, see .
*/
package com.qspin.qtaste.javaguifx.server;
import java.awt.Component;
import java.awt.Label;
import java.util.regex.Pattern;
import javax.swing.JLabel;
import javax.swing.JTree;
import com.qspin.qtaste.testsuite.QTasteTestFailException;
class TreeNodeSelector extends UpdateComponentCommander {
/**
* Type of node selection
*/
public enum SelectorIdentifier {
SELECT_BY_STRING,
SELECT_BY_REGEX,
CLEAR_SELECTION
}
private SelectorIdentifier mSelectorIdentifier;
/**
* < type of node selection
*/
protected volatile Object[] mPath; /**< tree path built in prepareDoActions() to select the node */
/**
* Constructor.
*
* @param selectorIdentifier type of node selection
*/
public TreeNodeSelector(SelectorIdentifier selectorIdentifier) {
mSelectorIdentifier = selectorIdentifier;
}
/**
* Compare a node path element (provided as argument of the selectNode method) to a node name (from a JTree).
*
* @param nodePathElement the node path element to compare with the node name
* @param nodeName the node name
* @return true if both match, false otherwise.
*/
protected boolean compareNodeNames(String nodePathElement, String nodeName) {
boolean comparisonResult = false;
switch (mSelectorIdentifier) {
case SELECT_BY_REGEX:
comparisonResult = Pattern.matches(nodePathElement, nodeName);
break;
case SELECT_BY_STRING:
default:
comparisonResult = nodePathElement.equals(nodeName);
break;
}
return comparisonResult;
}
/**
* Build a tree path (an array of objects) from a node path string and a node path separator.
*
* @throws QTasteTestFailException
*/
protected void prepareActions() throws QTasteTestFailException {
if (mSelectorIdentifier == SelectorIdentifier.CLEAR_SELECTION) {
// nothing special to do for CLEAR_SELECTION action
return;
}
String nodePath = mData[0].toString();
String nodePathSeparator = mData[1].toString();
// Split node path into an array of node path elements
// Be careful that String.split() method takes a regex as argument.
// Here, the token 'nodePathSeparator' is escaped using the Pattern.quote() method.
String[] nodePathElements = nodePath.split(Pattern.quote(nodePathSeparator));
if (nodePathElements.length == 0) {
throw new QTasteTestFailException(
"Unable to split the node path in elements (nodePath: '" + nodePath + "' separator: '" + nodePathSeparator
+ "')");
}
LOGGER.trace("nodePath: " + nodePath + " separator: " + nodePathSeparator + " splitted in " + nodePathElements.length
+ " element(s).");
// if (component instanceof JTree) {
// JTree tree = (JTree) component;
// TreeModel treeModel = tree.getModel();
// List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy