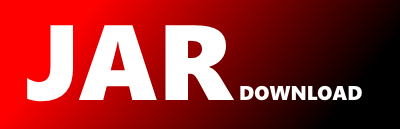
com.qualinsight.plugins.sonarqube.licensing.extension.ProductLicenseParser Maven / Gradle / Ivy
/*
* qualinsight-plugins-sonarqube-licensing
* Copyright (c) 2015-2016, QualInsight
* http://www.qualinsight.com/
*
* This program is free software: you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation, either
* version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this program. If not, you can retrieve a copy
* from .
*/
package com.qualinsight.plugins.sonarqube.licensing.extension;
import java.io.IOException;
import java.io.InputStream;
import com.verhas.licensor.License;
import org.apache.commons.lang.StringUtils;
import org.bouncycastle.openpgp.PGPException;
import org.joda.time.LocalDate;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.sonar.api.batch.BatchSide;
import org.sonar.api.server.ServerSide;
import com.qualinsight.plugins.sonarqube.licensing.model.Product;
import com.qualinsight.plugins.sonarqube.licensing.model.ProductEdition;
import com.qualinsight.plugins.sonarqube.licensing.model.ProductLicense;
import com.qualinsight.plugins.sonarqube.licensing.model.ProductVersionRange;
/**
* The {@link ProductLicenseParser} class is used in order to obtain a {@link ProductLicense} object for a {@link Product}. The {@link ProductLicense} is built based on a license key provided through the SonarQube UI.
*
* @author Michel Pawlak
*/
@BatchSide
@ServerSide
public final class ProductLicenseParser {
private static final Logger LOGGER = LoggerFactory.getLogger(ProductLicenseParser.class);
/**
* Public key ring digest used to ensure that the public key ring has not been replaced.
*/
private static final byte[] digest = new byte[] {
(byte) 0xD7,
(byte) 0x85,
(byte) 0x2F,
(byte) 0xB1,
(byte) 0xC8,
(byte) 0x57,
(byte) 0xAC,
(byte) 0x8A,
(byte) 0xD2,
(byte) 0x54,
(byte) 0xF6,
(byte) 0x84,
(byte) 0x4C,
(byte) 0xDD,
(byte) 0x8C,
(byte) 0xC1,
(byte) 0x0E,
(byte) 0x5B,
(byte) 0x01,
(byte) 0x6B,
(byte) 0xB8,
(byte) 0x13,
(byte) 0x31,
(byte) 0x89,
(byte) 0x08,
(byte) 0x10,
(byte) 0x7C,
(byte) 0xD2,
(byte) 0x65,
(byte) 0xB5,
(byte) 0x04,
(byte) 0xA9,
(byte) 0xD4,
(byte) 0xEE,
(byte) 0xBE,
(byte) 0xDC,
(byte) 0xCB,
(byte) 0xC3,
(byte) 0x32,
(byte) 0xF5,
(byte) 0xD7,
(byte) 0x7D,
(byte) 0xBD,
(byte) 0x68,
(byte) 0xED,
(byte) 0x24,
(byte) 0x1E,
(byte) 0xF1,
(byte) 0x80,
(byte) 0xF2,
(byte) 0x6E,
(byte) 0x84,
(byte) 0xA0,
(byte) 0x09,
(byte) 0x43,
(byte) 0x09,
(byte) 0x92,
(byte) 0x3F,
(byte) 0x37,
(byte) 0xF4,
(byte) 0x96,
(byte) 0xE9,
(byte) 0x7F,
(byte) 0xB3,
};
/**
* {@link ProductLicenseParser} IoC constructor.
*/
public ProductLicenseParser() {
}
/**
* Retrieves the {@link ProductLicense} matching the {@link Product}
*
* @param publicKeyInputStream {@link InputStream} to the public key to be used to decrypt the license key
* @param licenseKey license key string
* @return a {@link ProductLicense} corresponding to the registration type of the {@link Product}
*/
public ProductLicense parse(final InputStream publicKeyInputStream, final String licenseKey) {
final ProductLicense.Builder productLicenseBuilder = ProductLicense.Builder.instance();
if (StringUtils.isNotBlank(licenseKey)) {
final License license = new License();
try {
license.loadKeyRing(publicKeyInputStream, ProductLicenseParser.digest);
license.setLicenseEncoded(licenseKey);
productLicenseBuilder.withProductName(license.getFeature("product-name"));
productLicenseBuilder.withProductVersionRange(ProductVersionRange.fromString(license.getFeature("product-version")));
productLicenseBuilder.withServerId(license.getFeature("serverid"));
productLicenseBuilder.withProductEdition(ProductEdition.valueOf(license.getFeature("edition")));
productLicenseBuilder.withIssueDate(LocalDate.parse(license.getFeature("issue-date")));
productLicenseBuilder.withValidityDate(LocalDate.parse(license.getFeature("validity-date")));
} catch (final PGPException | IOException e) {
LOGGER.warn("A problem occurred while checking license validity.", e);
}
}
return productLicenseBuilder.build();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy