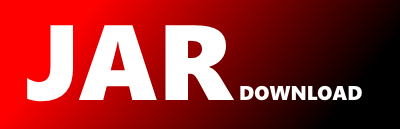
com.qualinsight.plugins.sonarqube.licensing.model.ProductLicense Maven / Gradle / Ivy
/*
* qualinsight-plugins-sonarqube-licensing
* Copyright (c) 2015-2016, QualInsight
* http://www.qualinsight.com/
*
* This program is free software: you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation, either
* version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this program. If not, you can retrieve a copy
* from .
*/
package com.qualinsight.plugins.sonarqube.licensing.model;
import com.google.common.collect.Range;
import org.joda.time.LocalDate;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import org.sonar.api.platform.Server;
import org.sonar.api.resources.Project;
/**
* Class that represents a product license.
*
* @author Michel Pawlak
*/
public final class ProductLicense {
private static final Logger LOGGER = LoggerFactory.getLogger(ProductLicense.class);
private String productName = null;
private Range productVersionRange = null;
private String serverId = null;
private ProductEdition productEdition = ProductEdition.UNKNOWN;
private LocalDate issueDate = null;
private LocalDate validityDate = null;
private ProductLicense() {
}
/**
* Checks if the {@link ProductLicense} allows the analysis of a specific SonarQube {@link Project}.
*
* @param server SonarQube {@link Server} instance
* @param runningProduct licensed {@link Product}
* @param validator execution context validator.
* @param context execution context used to improve logs
* @return true if the {@link ProductLicense} allows the analysis of the {@link Project}.
*/
public boolean allowsAnalysisOf(final Server server, final Product runningProduct, final ExecutionContextValidator validator, final String context) {
boolean checkable = true;
checkable = checkable && (this.productName != null);
checkable = checkable && (this.productVersionRange != null);
checkable = checkable && (this.serverId != null);
checkable = checkable && (this.issueDate != null);
checkable = checkable && (this.validityDate != null);
boolean analysisAllowed = false;
if (checkable) {
analysisAllowed = check(server, runningProduct, validator);
} else {
LOGGER.warn("{} - skipping {}: cannot validate license", runningProduct.name(), context);
}
return analysisAllowed;
}
private boolean check(final Server server, final Product runningProduct, final ExecutionContextValidator validator) {
boolean isValid = false;
if (checkRunningProduct(runningProduct) && checkServerId(server)) {
isValid = checkDates();
isValid = isValid && checkEdition(validator);
} else {
LOGGER.warn("{} - skipping analysis: license does not match running product !", runningProduct.name());
}
return isValid;
}
private boolean checkRunningProduct(final Product runningProduct) {
return this.productName.equals(runningProduct.name()) && this.productVersionRange.contains(runningProduct.version());
}
private boolean checkServerId(final Server server) {
return this.serverId.equals(server.getPermanentServerId());
}
private boolean checkDates() {
boolean isValid = false;
final LocalDate today = LocalDate.now();
if (this.issueDate.isBefore(today) || this.issueDate.isEqual(today)) {
if (today.isAfter(this.validityDate)) {
LOGGER.warn("{} - skipping analysis: product license has expired, please renew it !", this.productName);
} else {
isValid = true;
}
} else {
LOGGER.warn("{} - skipping analysis: system time was probably modified !", this.productName);
}
return isValid;
}
private boolean checkEdition(final ExecutionContextValidator validator) {
boolean isValid = false;
if (ProductEdition.FULL.equals(this.productEdition)) {
isValid = true;
LOGGER.debug("{} - running full analysis: product is registered.", this.productName);
} else if (ProductEdition.TRIAL.equals(this.productEdition)) {
isValid = validator.validate();
} else {
LOGGER.warn("{} - skipping analysis: product edition is unknown.", this.productName);
}
return isValid;
}
/**
* {@link ProductLicense} builder.
*
* @author Michel Pawlak
*/
public static class Builder {
private ProductLicense license;
private Builder() {
this.license = new ProductLicense();
}
/**
* Creates a {@link ProductLicense} builder.
*
* @return new builder instance
*/
public static final Builder instance() {
return new Builder();
}
/**
* Sets the product name.
*
* @param productName product name that is licensed.
* @return current builder
*/
public Builder withProductName(final String productName) {
this.license.productName = productName;
return this;
}
/**
* Sets the product version
*
* @param productVersionRange product version that is licensed.
* @return current builder
*/
public Builder withProductVersionRange(final Range productVersionRange) {
this.license.productVersionRange = productVersionRange;
return this;
}
/**
* Sets the serverId
*
* @param serverId SonarQube's serverId
* @return current builder
*/
public Builder withServerId(final String serverId) {
this.license.serverId = serverId;
return this;
}
/**
* Sets the product's productEdition
*
* @param edition product productEdition that is licensed
* @return current builder
*/
public Builder withProductEdition(final ProductEdition edition) {
this.license.productEdition = edition;
return this;
}
/**
* Sets the license's issue date
*
* @param issueDate date on which the license has been issued.
* @return current builder
*/
public Builder withIssueDate(final LocalDate issueDate) {
this.license.issueDate = issueDate;
return this;
}
/**
* Sets the license's validity date
*
* @param validityDate date until which the license is valid.
* @return current builder
*/
public Builder withValidityDate(final LocalDate validityDate) {
this.license.validityDate = validityDate;
return this;
}
/**
* Builds a {@link ProductLicense} with provided data.
*
* @return built {@link ProductLicense}
*/
public ProductLicense build() {
return this.license;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy