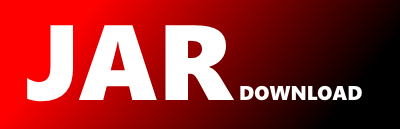
com.qualinsight.plugins.sonarqube.licensing.model.ProductVersion Maven / Gradle / Ivy
/*
* qualinsight-plugins-sonarqube-licensing
* Copyright (c) 2015-2016, QualInsight
* http://www.qualinsight.com/
*
* This program is free software: you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation, either
* version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this program. If not, you can retrieve a copy
* from .
*/
package com.qualinsight.plugins.sonarqube.licensing.model;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import org.apache.commons.lang.builder.EqualsBuilder;
import org.apache.commons.lang.builder.HashCodeBuilder;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.google.common.collect.ComparisonChain;
/**
* This class represents the version of a {@link Product}.
*
* @author Michel Pawlak
*/
public final class ProductVersion implements Comparable {
private static final Logger LOGGER = LoggerFactory.getLogger(ProductVersion.class);
/**
* Regular expression, version as String must match.
*/
private static final String VERSION_REGULAR_EXPRESSION = "^(\\d+)\\.(\\d+)\\.(\\d+)$";
private static final Pattern VERSION_PATTERN = Pattern.compile(VERSION_REGULAR_EXPRESSION);
private Integer majorVersion;
private Integer minorVersion;
private Integer incrementalVersion;
/**
* {@link ProductVersion} constructor.
*
* @param version {@link ProductVersion} as a String.
*/
public ProductVersion(final String version) {
final Matcher matcher = VERSION_PATTERN.matcher(version);
if (matcher.matches()) {
LOGGER.debug("Parsed product version: '{}.{}.{}'", matcher.group(1), matcher.group(2), matcher.group(3));
this.majorVersion = Integer.valueOf(matcher.group(1));
this.minorVersion = Integer.valueOf(matcher.group(2));
this.incrementalVersion = Integer.valueOf(matcher.group(3));
} else {
throw new IllegalStateException("Product version '" + version + "' is malformed.");
}
}
@Override
public int hashCode() {
return new HashCodeBuilder().append(this.majorVersion)
.append(this.minorVersion)
.append(this.incrementalVersion)
.hashCode();
}
@Override
public boolean equals(final Object other) {
if (other == null) {
return false;
}
if (other == this) {
return true;
}
if (other.getClass() != getClass()) {
return false;
}
final ProductVersion otherProductVersion = (ProductVersion) other;
return new EqualsBuilder().append(this.majorVersion, otherProductVersion.majorVersion)
.append(this.minorVersion, otherProductVersion.minorVersion)
.append(this.incrementalVersion, otherProductVersion.incrementalVersion)
.isEquals();
}
@Override
public int compareTo(final ProductVersion otherProductVersion) {
LOGGER.debug("this version: {}, other version: {}", this, otherProductVersion);
return ComparisonChain.start()
.compare(this.majorVersion, otherProductVersion.majorVersion)
.compare(this.minorVersion, otherProductVersion.minorVersion)
.compare(this.incrementalVersion, otherProductVersion.incrementalVersion)
.result();
}
@Override
public String toString() {
return new StringBuilder().append(this.majorVersion)
.append(".")
.append(this.minorVersion)
.append(".")
.append(this.incrementalVersion)
.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy