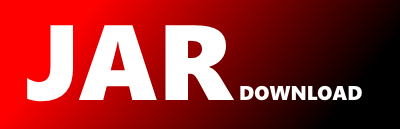
com.qualinsight.plugins.sonarqube.licensing.model.ProductVersionRange Maven / Gradle / Ivy
/*
* qualinsight-plugins-sonarqube-licensing
* Copyright (c) 2015-2016, QualInsight
* http://www.qualinsight.com/
*
* This program is free software: you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation, either
* version 3 of the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this program. If not, you can retrieve a copy
* from .
*/
package com.qualinsight.plugins.sonarqube.licensing.model;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import com.google.common.collect.BoundType;
import com.google.common.collect.Range;
/**
* Helper factory for {@link ProductVersion} {@link Range} instantiation.
*
* @author Michel Pawlak
*/
public final class ProductVersionRange {
private enum RangeType {
NORMAL("^(\\(|\\[)(\\d+\\.\\d+\\.\\d+)\\.\\.(\\d+\\.\\d+\\.\\d+)(\\)|\\])$",
new RangeFactory() {
@Override
Range build(final Matcher matcher) {
final String inclusiveLeftType = "[".equals(matcher.group(1)) ? "CLOSED" : "OPEN";
final String leftEndPoint = matcher.group(2);
final String rightEndPoint = matcher.group(3);
final String inclusiveRightType = "]".equals(matcher.group(4)) ? "CLOSED" : "OPEN";
return Range.range(new ProductVersion(leftEndPoint), BoundType.valueOf(inclusiveLeftType), new ProductVersion(rightEndPoint), BoundType.valueOf(inclusiveRightType));
}
}),
DOWN_TO("^(\\(|\\[)(\\d+\\.\\d+\\.\\d+)\\.\\.x\\)$",
new RangeFactory() {
@Override
Range build(final Matcher matcher) {
final String inclusiveLeftType = "[".equals(matcher.group(1)) ? "CLOSED" : "OPEN";
final String leftEndPoint = matcher.group(2);
return Range.downTo(new ProductVersion(leftEndPoint), BoundType.valueOf(inclusiveLeftType));
}
}),
UP_TO("^\\(x\\.\\.(\\d+\\.\\d+\\.\\d+)(\\)|\\])$",
new RangeFactory() {
@Override
Range build(final Matcher matcher) {
final String rightEndPoint = matcher.group(1);
final String inclusiveRightType = "]".equals(matcher.group(2)) ? "CLOSED" : "OPEN";
return Range.upTo(new ProductVersion(rightEndPoint), BoundType.valueOf(inclusiveRightType));
}
}),
ALL("^\\(x\\.\\.x\\)$",
new RangeFactory() {
@Override
Range build(final Matcher matcher) {
return Range.all();
}
});
Pattern pattern;
RangeFactory factory;
RangeType(final String regularExpression, final RangeFactory factory) {
this.pattern = Pattern.compile(regularExpression);
this.factory = factory;
}
private static abstract class RangeFactory {
abstract Range build(Matcher matcher);
}
}
private ProductVersionRange() {
}
/**
* Creates a {@link ProductVersion} {@link Range} from input {@link String}.
*
* @param rangeString input String representing a {@link ProductVersion} {@link Range}
* @return {@link ProductVersion} {@link Range}
*/
public static Range fromString(final String rangeString) {
for (final RangeType rangeType : RangeType.values()) {
final Matcher matcher = rangeType.pattern.matcher(rangeString);
if (matcher.matches()) {
return rangeType.factory.build(matcher);
}
}
throw new IllegalStateException("Invalid product version range: '" + rangeString + "'.");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy