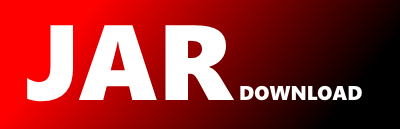
com.quamto.jira.data.common.JEnumerators Maven / Gradle / Ivy
The newest version!
package com.quamto.jira.data.common;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.HashMap;
/**
* Jhagi enumerations of the project
* @author jholguin
* @since 12/06/2014
*/
public abstract class JEnumerators {
/**
* Defines Boolean values
* @author Jhon-Aleck
*
*/
public enum YesNo{
No(0),Yes(1), Null(-1);
private final int value;
private YesNo(int value){
this.value = value;
}
public int getInt(){
return value;
}
/**
* Returns the enum value from integer value
* @param value Integer value
* @return Enumeration value
*/
public YesNo getEnumValue(int value){
YesNo enumValue = Null;
switch (value) {
case 0:
enumValue = No;
break;
case 1:
enumValue = Yes;
break;
case -1:
enumValue = Null;
break;
}
return enumValue;
}
}
/**
* Defines the complexities
* @author jholguin
*
*/
public enum Complexities{
High(0),
Medium(1),
Low(2);
private final int intValue;
private final String description;
private Complexities(int value){
this.intValue = value;
switch (value) {
case 0:
this.description = "High";
break;
case 1:
this.description = "Medium";
break;
case 2:
this.description = "Low";
break;
default:
this.description = "";
break;
}
}
public int getInt(){
return intValue;
}
public String getDescription(){
return description;
}
/**
* Returns the enum value from integer value
* @param value Integer value
* @return Enumeration value
*/
public Complexities getEnumValue(int value){
Complexities enumValue = High;
switch (value) {
case 0:
enumValue = High;
break;
case 1:
enumValue = Medium;
break;
case 2:
enumValue = Low;
break;
}
return enumValue;
}
/**
* Gets map with the enum values and description
*
*/
public Map getMapEnumValues(){
Map mapEnum = new HashMap();
for (Complexities type : Complexities.values()) {
mapEnum.put(type, type.getDescription());
}
return mapEnum;
}
/**
* Gets map with the integer values and description
*
*/
public Map getMapIntValues(){
Map mapEnum = new HashMap();
for (Complexities type : Complexities.values()) {
mapEnum.put(type.getInt(), type.getDescription());
}
return mapEnum;
}
}
/**
* Jhagi enumerations
* @author jholguin
*
*/
public enum PluginEntities{
Projects(0),
DesignElements(1),
DesignPackages(2),
TestCases(3),
Issues(4);
private final Integer intValue;
private PluginEntities(Integer value){
this.intValue = value;
}
/**
* Gets the integer value
*/
public Integer getInt(){
return this.intValue;
}
/**
* Returns the enum value from integer value
* @param value Integer value
* @return Enumeration value
*/
public PluginEntities getEnumValue(Integer value){
PluginEntities enumValue = null;
switch (value) {
case 0:
enumValue = Projects;
break;
case 1:
enumValue = DesignElements;
break;
case 2:
enumValue = DesignPackages;
break;
case 3:
enumValue = TestCases;
break;
case 4:
enumValue = Issues;
break;
}
return enumValue;
}
}
/**
* Jhagi enumerations
* @author lecheverry
*
*/
public enum NotesTypes{
Normal(0),
Checklist(1);
private final Integer intValue;
private NotesTypes(Integer value){
this.intValue = value;
}
/**
* Gets the integer value
*/
public Integer getInt(){
return this.intValue;
}
/**
* Returns the enum value from integer value
* @param value Integer value
* @return Enumeration value
*/
public NotesTypes getEnumValue(Integer value){
NotesTypes enumValue = null;
switch (value) {
case 0:
enumValue = Normal;
break;
case 1:
enumValue = Checklist;
break;
}
return enumValue;
}
}
/**
* Define the jhagi elements states
* @author jholguin
*
*/
public enum States{
Pending(0),
InProgress(1),
Finished(2),
Freeze(3),
Canceled(4);
private Integer intValue = 0;
private String enumDescription = "";
private States(Integer value){
intValue = value;
switch (value) {
case 0:
enumDescription = "Pending";
break;
case 1:
enumDescription = "In Progress";
break;
case 2:
enumDescription = "Finished";
break;
case 3:
enumDescription = "Stoped";
break;
case 4:
enumDescription = "Canceled";
break;
}
}
/**
* Gets the integer value from the enum value
* @return Integer value
*/
public Integer getInt(){
return intValue;
}
/**
* Gets the description from the enum value
* @return Enumeration item description
*/
public String getDescription(){
return enumDescription;
}
/**
* Get the enum value from integer value
*
* @param value Integer value to map
* @return Enum value mapped
*/
public States getEnumValue(Integer value){
States enumValue = Pending;
switch (value) {
case 0:
enumValue = Pending;
break;
case 1:
enumValue = InProgress;
break;
case 2:
enumValue = Finished;
break;
case 3:
enumValue = Freeze;
break;
case 4:
enumValue = Canceled;
break;
}
return enumValue;
}
/**
* Get a Hashmap from the enum with the ids and descriptions
*
* @return Hashmap
*/
public Map getMapEnumValues(){
Map mapEnum = new HashMap();
for (States item : States.values()) {
mapEnum.put(item, item.getDescription());
}
return mapEnum;
}
/**
* Get a Hashmap from the enum with the integer ids and descriptios
*
* @return Hashmap
*/
public Map getMapIntValues(){
Map mapEnum = new HashMap();
for (States item : States.values()) {
mapEnum.put(item.getInt(), item.getDescription());
}
return mapEnum;
}
}
/**
* Define the Jhagi Modules
*
* @author jholguin
*
*/
public enum Modules{
Projects(0),
Issues(1),
Design(2),
Test(3);
private final int intValue;
private final String description;
private Modules(int value){
this.intValue = value;
switch (value) {
case 0:
this.description = "Projects";
break;
case 1:
this.description = "Issues";
break;
case 2:
this.description = "Design";
break;
case 3:
this.description = "Test";
break;
default:
this.description = "";
break;
}
}
public int getInt(){
return intValue;
}
public String getDescription(){
return description;
}
/**
* Gets enum value mapped from integer value
* @param value Integer Value
* @return Enum value mapped
*/
public Modules getEnumValue(int value){
Modules enumValue = null;
switch (value) {
case 0:
enumValue = Projects;
break;
case 1:
enumValue = Issues;
break;
case 2:
enumValue = Design;
break;
case 3:
enumValue = Test;
break;
}
return enumValue;
}
/**
*
* @return Gets a Map with the enum values and descriptions
*/
public Map getMapEnumValues(){
Map mapEnum = new HashMap();
for (Modules type : Modules.values()) {
mapEnum.put(type, type.getDescription());
}
return mapEnum;
}
/**
*
* @return Gets a Map with the integer values and descriptions
*/
public Map getMapIntValues(){
Map mapEnum = new HashMap();
for (Modules type : Modules.values()) {
mapEnum.put(type.getInt(), type.getDescription());
}
return mapEnum;
}
/**
* @return Gets a Hashmap with the string values
*/
public List> getListEnum(){
List> list = new ArrayList>();
for (Modules moduleIdentifier : Modules.values()) {
HashMap mapEnum = new HashMap();
mapEnum.put("name", moduleIdentifier.name());
mapEnum.put("description", moduleIdentifier.getDescription());
list.add(mapEnum);
}
return list;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy