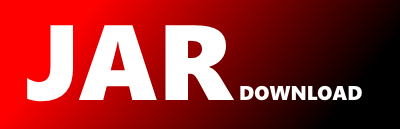
com.quamto.jira.data.design.dao.PackageDAO Maven / Gradle / Ivy
The newest version!
package com.quamto.jira.data.design.dao;
import java.sql.ResultSet;
import java.util.ArrayList;
import java.util.List;
import com.quamto.core.IUserMessages;
import com.quamto.core.QException;
import com.quamto.core.QException.ExceptionType;
import com.quamto.core.Result;
import com.quamto.db.DbConnection;
import com.quamto.db.SQLParameter.SQLDataType;
import com.quamto.entity.BaseEntity;
import com.quamto.entity.BaseEntityDAO;
import com.quamto.jira.data.common.UserMessages;
import com.quamto.jira.data.common.UserMessages.DesignMessages;
import com.quamto.jira.data.design.entity.PackageEntity;
public class PackageDAO extends BaseEntityDAO{
public PackageDAO(DbConnection conexionBD){
super(conexionBD, "qji_design_packages", "pak_id", PackageDAO.class);
}
/**
* Gets an object of type Package charged according to the record identifier
*/
@Override
public PackageEntity get(Long id) throws QException {
PackageEntity ent = null;
try {
ent = (PackageEntity)getEntityLoaded(id);
} catch (Exception e) {
throw new QException(e, ExceptionType.LoadData_err, subClassName + "-get");
}
return ent;
}
/**
* Returns a list of Package stored in the database
*/
@Override
public List getAll() throws QException {
ArrayList designPackagesList = new ArrayList();
try {
ResultSet rs = getResultSet();
while(rs.next()){
designPackagesList.add(loadEntityAttributesFromRs(rs));
}
} catch (Exception e) {
throw new QException(e, ExceptionType.LoadData_err, subClassName + "-getAll");
}
return designPackagesList;
}
/**
* Gets the list of PackageEntity based on an specific entity
*
* @param idProject Project Owner Identifier
* @return List of attachments associated with the entity ( without attachment only information attached)
* @throws QException Throws an exception if the operation did not achieve
*/
public List getAllByProject(Long idProject, Long idOwner) throws QException {
ResultSet rs = null;
ArrayList packageList= new ArrayList();
try {
String sentenceSQL = "SELECT * "+
" FROM qji_design_packages "+
" WHERE pak_id_project = " +idProject+
" AND pak_id_owner = "+idOwner;
rs = getResultSet(sentenceSQL);
while(rs.next()){
packageList.add(loadEntityAttributesFromRs(rs));
}
} catch (Exception e) {
throw new QException(e, ExceptionType.OperationFailed_err, subClassName + "-getAllByProject");
}
return packageList;
}
/**
* Make the assignment of the fields and values of the entity to be used in
* Operations insert and update data
*/
@Override
protected void loadQueryParameters(BaseEntity entity)
throws QException {
try {
PackageEntity ent = (PackageEntity)entity;
addQueryParameter("pak_id", ent.getID(), SQLDataType.Long_sdt);
addQueryParameter("pak_name", ent.getName(), SQLDataType.String_sdt);
addQueryParameter("pak_description", ent.getDescription(), SQLDataType.String_sdt);
addQueryParameter("pak_id_parent", ent.getIdParent(), SQLDataType.Long_sdt);
addQueryParameter("pak_id_project", ent.getIdProject(), SQLDataType.Long_sdt);
addQueryParameter("pak_id_owner", ent.getIdOwner(), SQLDataType.Long_sdt);
addQueryParameter("pak_id_user", ent.getIdUser(), SQLDataType.String_sdt);
} catch (Exception e) {
throw new QException(e, ExceptionType.LoadData_err, subClassName + "-loadQueryParameters");
}
}
/**
* result set load values corresponding to the table of the entity in the object returned
*/
@Override
protected PackageEntity loadEntityAttributesFromRs(ResultSet rs) throws QException {
PackageEntity ent = new PackageEntity();
try {
ent.setID(rs.getLong("pak_id"));
ent.setName(rs.getString("pak_name"));
ent.setDescription(rs.getString("pak_description"));
ent.setIdParent(rs.getLong("pak_id_parent"));
ent.setIdProject(rs.getLong("pak_id_project"));
ent.setIdOwner(rs.getLong("pak_id_owner"));
ent.setIdUser(rs.getString("pak_id_user"));
} catch (Exception e) {
throw new QException(e, ExceptionType.LoadData_err, subClassName + "-loadEntityAttributesFromRs");
}
return ent;
}
/**
*
* @param id Deletes the Package with param identifier
* @return Operation result
*/
public Result deletePackage(Long id){
Result result = new Result();
try {
try(ElementBaseDAO elementDAO = new ElementBaseDAO(dbConnection)){
Boolean hasChildren = elementDAO.existElementsByPackage(id);
if(!hasChildren){
ResultSet rsTable =
dbOperator.getResultSet("SELECT " + entityIdFieldName
+ " FROM " + entityTableName
+ " WHERE pak_id_parent=" + id);
while(rsTable.next()){
Long idChildPkg = rsTable.getLong(entityIdFieldName);
result = deletePackage(idChildPkg);
if(!result.isSuccessful()){
break;
}
}
}else{
IUserMessages userMessage = new UserMessages(DesignMessages.PackageWithElements);
result.setException(new QException(ExceptionType.OperationNotAllowed_err,
userMessage));
}
if(result.isSuccessful()){
result = delete(id);
}
}
} catch (Exception e) {
result.setException(new QException(e, ExceptionType.OperationFailed_err, subClassName + "-deletePackage"));
}
return result;
};
@Override
public void close() throws Exception {
try {
} catch (Exception e) {
throw e;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy