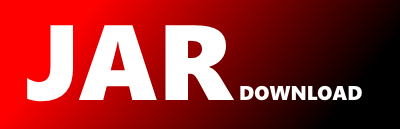
com.quamto.jira.data.design.entity.ElementBaseEntity Maven / Gradle / Ivy
The newest version!
package com.quamto.jira.data.design.entity;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.quamto.entity.BaseEntity;
import com.quamto.jira.data.common.JEnumerators.Complexities;
import com.quamto.jira.data.common.UserMessages;
import com.quamto.jira.data.common.UserMessages.ModuleMessages;
public class ElementBaseEntity extends BaseEntity {
private String name = null;
private String description = null;
private DesignElementTypes elementType;
private long idStereotype = 0;
private long idPackageOwner = 0;
private long idPriority = 0;
private Complexities complexity = Complexities.Low;
private long idParent = 0;
private Long idProject = null;
private String idUser = null;
public ElementBaseEntity(DesignElementTypes TypeElement){
this.elementType = TypeElement;
}
public ElementBaseEntity(){
}
/**
* @return Integer value from engine type enum value
*/
public Integer getComplexityInt(){
return this.complexity.getInt();
}
/**
* Enum that define the design elements types
*
* @author lardila / jholguin
* @since 16/07/2015
*
*/
public enum DesignElementTypes{
Generic(-1),
UseCase(0),
Classes(1),
Enumeration(2),
Table(3),
Actor(4),
Component(7),
Interface(8),
Node(9),
Device(10),
Environment(11);
private final int valueInt;
private final String enumDescription;
private DesignElementTypes(int value){
UserMessages userMsg = new UserMessages(ModuleMessages.EnumeratorMessages);
this.valueInt = value;
this.enumDescription = userMsg.getEnumeratorDescription(DesignElementTypes.class.getSimpleName(), this.valueInt);
}
public int getInt(){
return valueInt;
}
public String getDescription(){
return enumDescription;
}
/**
* Returns the enumeration value of the integer sent
* @param value Value to be mapped related to the enumeration
* @return enumValue value of the enum
*/
public DesignElementTypes getEnumValue(int value){
DesignElementTypes enumValue = null;
switch (value) {
case -1:
enumValue = DesignElementTypes.Generic;
break;
case 0:
enumValue = DesignElementTypes.UseCase;
break;
case 1:
enumValue = DesignElementTypes.Classes;
break;
case 2:
enumValue = DesignElementTypes.Enumeration;
break;
case 3:
enumValue = DesignElementTypes.Table;
break;
case 4:
enumValue = DesignElementTypes.Actor;
break;
case 7:
enumValue = DesignElementTypes.Component;
break;
case 8:
enumValue = DesignElementTypes.Interface;
break;
case 9:
enumValue = DesignElementTypes.Node;
break;
case 10:
enumValue = DesignElementTypes.Device;
break;
case 11:
enumValue = DesignElementTypes.Environment;
break;
}
return enumValue;
}
/**
* Gets an Enum Hashmap with values and description
*
* @return Values Map of the enum with its related description
*/
public Map getMapEnumValues(){
Map mapEnum = new HashMap();
for (DesignElementTypes elmType : DesignElementTypes.values()) {
mapEnum.put(elmType, elmType.getDescription());
}
return mapEnum;
}
/**
* Gets a Hashmap of the enumeration with int values and each enum name
*
* @return Map with all of the int values and name defined for each enum
*/
public Map getMapIntValuesNames(){
Map mapEnum = new HashMap();
for (DesignElementTypes type : DesignElementTypes.values()) {
mapEnum.put(type.getInt(), type.name());
}
return mapEnum;
}
/**
* Gets a Hashmap of the enumeration with int values and each enum name
*
* @return Map with all of the int values and name defined for each enum
*/
public Map getMapIntValuesDescription(){
Map mapEnum = new HashMap();
for (DesignElementTypes type : DesignElementTypes.values()) {
mapEnum.put(type.getInt(), type.getDescription());
}
return mapEnum;
}
/**
* Gets a list of hashmaps of the enumeration with each enum value, name and description.
* @return List with all of the enum values.
*/
public List> getListEnum(){
List> list = new ArrayList>();
for (DesignElementTypes elmType : DesignElementTypes.values()) {
HashMap mapEnum = new HashMap();
mapEnum.put("name", elmType.name() );
mapEnum.put("description", elmType.getDescription() );
list.add(mapEnum);
}
return list;
}
}
public enum ClassDataType{
NC_Dt(0),
String_Dt(1),
Decimal_Dt(2),
Blob_Dt(3),
Date_Dt(4),
Boolean_Dt(5),
Object_Dt(6);
private final int valueInt;
private final String enumDescription;
private ClassDataType(int value){
UserMessages userMsg = new UserMessages(ModuleMessages.EnumeratorMessages);
this.valueInt = value;
this.enumDescription = userMsg.getEnumeratorDescription(ClassDataType.class.getSimpleName(), this.valueInt);
}
public int getInt(){
return valueInt;
}
public String getDescription(){
return enumDescription;
}
/**
* Returns the value of the enum according to the int value received
* @param value Value to be mapped according to the enum
* @return Enum value
*/
public ClassDataType getEnumValue(int value){
ClassDataType enumValue = null;
switch (value) {
case 0:
enumValue = ClassDataType.NC_Dt;
break;
case 1:
enumValue = ClassDataType.String_Dt;
break;
case 2:
enumValue = ClassDataType.Decimal_Dt;
break;
case 3:
enumValue = ClassDataType.Blob_Dt;
break;
case 4:
enumValue = ClassDataType.Date_Dt;
break;
case 5:
enumValue = ClassDataType.Boolean_Dt;
break;
case 6:
enumValue = ClassDataType.Object_Dt;
break;
}
return enumValue;
}
/**
* Gets a Hashmap of the enumeration with int values and each description
*
* @return Map with all of the int values and description defined for each enum
*/
public Map getMapIntValues(){
Map mapEnum = new HashMap();
for (ClassDataType seqType : ClassDataType.values()) {
mapEnum.put(seqType.getInt(), seqType.getDescription());
}
return mapEnum;
}
public Map getMapEnumValues(){
Map mapEnum = new HashMap();
for (ClassDataType tipoCmp : ClassDataType.values()) {
mapEnum.put(tipoCmp, tipoCmp.getDescription());
}
return mapEnum;
}
public List> getListEnum(){
List> mapEnum = new ArrayList>();
for (ClassDataType tipoCmp : ClassDataType.values()) {
HashMap hashMap = new HashMap();
hashMap.put("name", tipoCmp.name());
hashMap.put("description", tipoCmp.getDescription());
mapEnum.add(hashMap);
}
return mapEnum;
}
}
/**
* @return Name of the design element
*/
public String getName(){
return this.name;
}
/**
* @param value Name of the design element
*/
public void setName(String value){
this.name = value;
}
/**
* @return Design element description
*/
public String getDescription(){
return this.description;
}
/**
* @param value Design element description
*/
public void setDescription(String value){
this.description = value;
}
/**
* @return Design element type assigned
*/
public DesignElementTypes getElementType(){
return this.elementType;
}
/**
* @param value Design element type assigned
*/
public void setElementType(DesignElementTypes value){
this.elementType = value;
}
/**
* @return Id Stereotype ID of the design element
*/
public long getIdStereotype(){
return this.idStereotype;
}
/**
* @param value Stereotype ID of the design element
*/
public void setIdStereotype(long value){
this.idStereotype = value;
}
/**
* @return Package owner of the design element
*/
public long getIdPackageOwner(){
return this.idPackageOwner;
}
/**
* @param value Package owner of the design element
*/
public void setIdPackageOwner(long value){
this.idPackageOwner = value;
}
/**
* @return Priority of the design element
*/
public long getIdPriority(){
return this.idPriority;
}
/**
* @param value Priority of the design element
*/
public void setIdPriority(long value){
this.idPriority = value;
}
/**
* @return Complexity of the design element
*/
public Complexities getComplexity(){
return this.complexity;
}
/**
* @param value Complexity of the design element
*/
public void setComplexity(Complexities value){
this.complexity = value;
}
/**
* @return Parent design element id
*/
public long getIdParent(){
return this.idParent;
}
/**
* @param value Parent design element id
*/
public void setIdParent(long value){
this.idParent = value;
}
/**
* @return Project identifier owner of the design element
*/
public Long getIdProject() {
return idProject;
}
/**
* @param idProject Project identifier owner of the design element
*/
public void setIdProject(Long idProject) {
this.idProject = idProject;
}
/**
* @return Gets the design element type description
*/
public String getDesignElementTypeDespcription(){
return this.elementType.getDescription();
}
/**
* @return Integer value from package type enum value
*/
public Integer getDesignElementTypeInt(){
return this.elementType.getInt();
}
/**
* @return the idUser that creates the element
*/
public String getIdUser() {
return idUser;
}
/**
* @param idUser that creates the element
*/
public void setIdUser(String idUser) {
this.idUser = idUser;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy