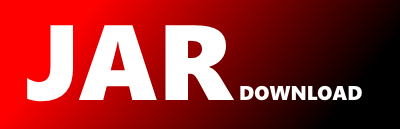
com.quamto.jira.data.design.entity.UseCaseScenarioEntity Maven / Gradle / Ivy
The newest version!
package com.quamto.jira.data.design.entity;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import com.quamto.entity.BaseEntity;
import com.quamto.jira.data.common.UserMessages;
import com.quamto.jira.data.common.UserMessages.ModuleMessages;
/**
* Class that contains the scenario entity definition
* @author jholguin
*
*/
public class UseCaseScenarioEntity extends BaseEntity {
private String name;
private ScenarioTypes scenarioType;
private long idElement;
private Integer principalStepJoin;
/**
* Contains the escenario types
*
* @author jholguin
* @since 03/06/2016
*
*/
public enum ScenarioTypes{
PrimaryRoute(0),
AlternateRoute(1),
Exception(2);
private final int valueInt;
private final String enumDescription;
private ScenarioTypes(int value){
UserMessages userMsg = new UserMessages(ModuleMessages.EnumeratorMessages);
this.valueInt = value;
this.enumDescription = userMsg.getEnumeratorDescription(ScenarioTypes.class.getSimpleName(), this.valueInt);
}
public int getInt(){
return valueInt;
}
public String getDescription(){
return enumDescription;
}
public ScenarioTypes getEnumValue(int valor){
ScenarioTypes enumValue = null;
switch (valor) {
case 0:
enumValue = ScenarioTypes.PrimaryRoute;
break;
case 1:
enumValue = ScenarioTypes.AlternateRoute;
break;
case 2:
enumValue = ScenarioTypes.Exception;
break;
}
return enumValue;
}
public Map getMapEnumValues(){
Map mapEnum = new HashMap();
for (ScenarioTypes tipoElm : ScenarioTypes.values()) {
mapEnum.put(tipoElm, tipoElm.getDescription());
}
return mapEnum;
}
public List> getListEnum(){
List> list = new ArrayList>();
for (ScenarioTypes tipoElm : ScenarioTypes.values()) {
HashMap mapEnum = new HashMap();
mapEnum.put("name", tipoElm.name() );
mapEnum.put("description", tipoElm.getDescription() );
list.add(mapEnum);
}
return list;
}
/**
* Gets a Hashmap of the enumeration with int values and each enum name
*
* @return Map with all of the int values and name defined for each enum
*/
public Map getMapIntValuesDescription(){
Map mapEnum = new HashMap();
for (ScenarioTypes type : ScenarioTypes.values()) {
mapEnum.put(type.getInt(), type.getDescription());
}
return mapEnum;
}
}
/**
* @return Name assigned for the scenario
*/
public String getName(){
return this.name;
}
/**
* @param value assigned for the scenario
*/
public void setName(String value){
this.name = value;
}
/**
* @return scenario type assigned
*/
public ScenarioTypes getScenarioType(){
return this.scenarioType;
}
/**
* @param value type assigned
*/
public void setScenarioType(ScenarioTypes value){
this.scenarioType = value;
}
public Integer getScenarioTypeInt(){
return this.scenarioType.getInt();
}
public String getScenarioTypeString(){
return this.scenarioType.getDescription();
}
/**
* @return Design element assigned to the scenario
*/
public long getIdElement(){
return this.idElement;
}
/**
* @param value Design element assigned to the scenario
*/
public void setIdElement(long value){
this.idElement = value;
}
public Integer getPrincipalStepJoin() {
return principalStepJoin;
}
public void setPrincipalStepJoin(Integer value) {
this.principalStepJoin = value;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy