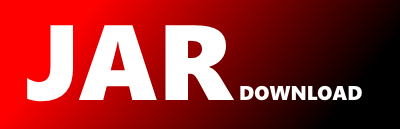
com.quamto.jira.data.time.dao.TeamIssuesDAO Maven / Gradle / Ivy
The newest version!
package com.quamto.jira.data.time.dao;
import java.sql.ResultSet;
import java.util.ArrayList;
import java.util.List;
import com.quamto.core.QException;
import com.quamto.core.QException.ExceptionType;
import com.quamto.core.Result;
import com.quamto.db.DbConnection;
import com.quamto.db.SQLParameter.SQLDataType;
import com.quamto.entity.BaseEntity;
import com.quamto.entity.BaseEntityDAO;
import com.quamto.jira.data.design.dao.ElementBaseDAO;
import com.quamto.jira.data.time.entity.TeamIssuesEntity;
public class TeamIssuesDAO extends BaseEntityDAO {
public TeamIssuesDAO(DbConnection dbConnection){
super(dbConnection, "qji_team_issues", "tis_id", TeamIssuesDAO.class);
}
/**
* Get an instance of the entity with data loaded according to Id provided
*/
@Override
public TeamIssuesEntity get(Long id) throws QException {
TeamIssuesEntity entity = null;
try {
entity = (TeamIssuesEntity)getEntityLoaded(id);
} catch (Exception e) {
throw new QException(e, ExceptionType.LoadData_err, subClassName + "-get");
}
return entity;
}
/**
* Get by issue
*/
public Boolean getByIssue(Integer idIssue, Long idTeam) throws QException {
Boolean hasChildren = false;
try {
hasChildren = dbOperator.existValueInTable(entityTableName, "tis_id_issue", idIssue, " tis_id_team=" + idTeam);
} catch (Exception e) {
throw e;
}
return hasChildren;
}
/**
* Gets list of pause types related to the owner in the database
* @return Return a list of pause types related to the owner in the database
*/
public List getAllByTeam(Long idTeam) throws QException {
ArrayList listTimeRegistrationTypes = new ArrayList();
try {
ResultSet rs = getResultSet();
String query = "SELECT * "
+ " FROM " + entityTableName
+ " WHERE tis_id_team = " + idTeam;
rs = dbOperator.getResultSet(query);
while(rs.next()){
listTimeRegistrationTypes.add(loadEntityAttributesFromRs(rs));
}
} catch (Exception e) {
throw new QException(e, ExceptionType.LoadData_err, subClassName + "-getAllByGroup");
}
return listTimeRegistrationTypes;
}
/**
* Gets list of pause types related to the owner in the database
* @param idUsers Owner identifier to be consulted
* @return Return a list of pause types related to the owner in the database
*/
public List getAllByUser(ArrayList idUsers, Long idOwner) throws QException {
ArrayList listTimeRegistrationTypes = new ArrayList();
try {
ResultSet rs = getResultSet();
String condition = "(";
for (int i = 0; i < idUsers.size(); i++) {
condition += "'"+idUsers.get(i)+"'";
if( i == (idUsers.size() - 1) ){
condition += ")";
}else{
condition += ",";
};
};
String query = " SELECT * "+
" FROM "+entityTableName+
" INNER JOIN qji_teams ON tis_id_team=tea_id" +
" WHERE tea_id_owner=" + idOwner.toString() +
" AND tis_id_user IN "+condition;
rs = dbOperator.getResultSet(query);
while(rs.next()){
listTimeRegistrationTypes.add(loadEntityAttributesFromRs(rs));
}
} catch (Exception e) {
throw new QException(e, ExceptionType.LoadData_err, subClassName + "-getAllByUser");
}
return listTimeRegistrationTypes;
}
/**
*
* @param id Deletes the Package with param identifier
* @return Operation result
*/
public Result deleteByIssue(Long id){
Result result = new Result();
try {
try(ElementBaseDAO elementDAO = new ElementBaseDAO(dbConnection)){
String sentenciaSQL = "DELETE FROM " + entityTableName
+ " WHERE tis_id_issue = " + id ;
result = dbOperator.executeQuery(sentenciaSQL);
}
} catch (Exception e) {
result.setException(new QException(e, ExceptionType.OperationFailed_err, subClassName + "-deletePackage"));
}
return result;
};
/**
* Make assignments of fields and values of entity to be used in
* insertion and update database operations
*/
@Override
public void loadQueryParameters(BaseEntity entity)
throws QException {
try {
TeamIssuesEntity ent = (TeamIssuesEntity) entity;
addQueryParameter("tis_id", ent.getID(), SQLDataType.Long_sdt);
addQueryParameter("tis_id_team", ent.getIdTeam(), SQLDataType.Long_sdt);
addQueryParameter("tis_id_user", ent.getIdUser(), SQLDataType.String_sdt);
addQueryParameter("tis_id_issue", ent.getIdIssue(), SQLDataType.Integer_sdt);
addQueryParameter("tis_start_date", ent.getStartDate(), SQLDataType.DateTime_sdt);
} catch (Exception e) {
throw new QException(e, ExceptionType.LoadData_err, subClassName + "-loadQueryParameters");
}
}
/**
* Load the values of ResultSet of table from entity in to returned object.
*/
@Override
public TeamIssuesEntity loadEntityAttributesFromRs(ResultSet rs) throws QException {
TeamIssuesEntity entity = new TeamIssuesEntity();
try {
entity.setID(rs.getLong("tis_id"));
entity.setIdTeam(rs.getLong("tis_id_team"));
entity.setIdUser(rs.getString("tis_id_user"));
entity.setIdIssue(rs.getInt("tis_id_issue"));
entity.setStartDate(rs.getTimestamp("tis_start_date"));
} catch (Exception e) {
throw new QException(e, ExceptionType.LoadData_err, subClassName + "-loadEntityAttributesFromRs");
}
return entity;
}
@Override
public void close() throws Exception {
}
@Override
public List> getAll() throws QException {
return null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy