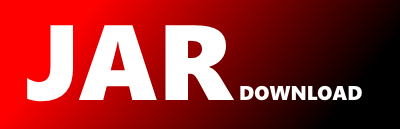
com.quartzdesk.api.common.encoding.BASE64Encoder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of quartzdesk-api Show documentation
Show all versions of quartzdesk-api Show documentation
QuartzDesk Public API library required for QuartzDesk Standard and Enterprise edition installations. This library must be placed on the classpath of the Quartz scheduler based application that is managed by QuartzDesk. It is important that this library is loaded by the same classloader that loads the Quartz scheduler API used by the application.
The newest version!
/*
* Copyright (c) 2013-2024 QuartzDesk.com. All Rights Reserved.
* QuartzDesk.com PROPRIETARY/CONFIDENTIAL. Use is subject to license terms.
*/
package com.quartzdesk.api.common.encoding;
/**
* This is a utility class which is used to encode a byte array into a MIME
* BASE64 encoded string. For further information about MIME BASE64 encoding,
* see RFC2045.
*
* @version $Id:$
*/
public final class BASE64Encoder
{
private static final char[] BASE64_ALPHABET =
{ 'A', 'B', 'C', 'D', 'E', 'F', 'G', 'H', 'I', 'J', 'K', 'L', 'M', 'N', 'O', 'P', 'Q', 'R',
'S', 'T', 'U', 'V', 'W', 'X', 'Y', 'Z', 'a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j',
'k', 'l', 'm', 'n', 'o', 'p', 'q', 'r', 's', 't', 'u', 'v', 'w', 'x', 'y', 'z', '0', '1',
'2', '3', '4', '5', '6', '7', '8', '9', '+', '/', '=' };
private static final int PAD_CHAR_INDEX = 64;
/**
* Private constructor of a utility class.
*/
private BASE64Encoder()
{
}
/**
* Encodes a byte array into MIME BASE64 encoded string.
*
* @param bytes the array of bytes to be encoded.
* @return the MIME BASE64 encoded string.
*/
public static String encode( byte[] bytes )
{
// CSOFF: MagicNumber
StringBuilder sb = new StringBuilder();
int first;
int second;
int third;
int i = 0;
if ( bytes == null ) return null;
while ( i < bytes.length )
{
first = bytes[i++];
if ( first < 0 ) first += 256;
sb.append( BASE64_ALPHABET[first >>> 2] );
if ( i < bytes.length )
{
second = bytes[i++];
if ( second < 0 ) second += 256;
sb.append( BASE64_ALPHABET[( second >>> 4 ) | ( ( first & 0x3 ) << 4 )] );
if ( i < bytes.length )
{
third = bytes[i++];
if ( third < 0 ) third += 256;
sb.append( BASE64_ALPHABET[( third >>> 6 ) | ( ( second & 0xf ) << 2 )] );
sb.append( BASE64_ALPHABET[( third & 0x3f )] );
}
else
{ // there is only the second byte of the triplet available
sb.append( BASE64_ALPHABET[( second & 0xf ) << 2] );
sb.append( BASE64_ALPHABET[PAD_CHAR_INDEX] );
}
}
else
{
// there is only the first byte of the triplet available
sb.append( BASE64_ALPHABET[( first & 0x3 ) << 4] );
sb.append( BASE64_ALPHABET[PAD_CHAR_INDEX] );
sb.append( BASE64_ALPHABET[PAD_CHAR_INDEX] );
}
}
return sb.toString();
// CSON: MagicNumber
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy