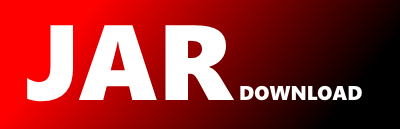
com.quhaodian.data.core.Finder Maven / Gradle / Ivy
package com.quhaodian.data.core;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import org.hibernate.Session;
import org.hibernate.query.Query;
import org.hibernate.type.Type;
/**
* HQL语句分页查询
*/
public class Finder {
protected Finder() {
hqlBuilder = new StringBuilder();
}
protected Finder(String hql) {
hqlBuilder = new StringBuilder(hql);
}
public static Finder create() {
return new Finder();
}
public static Finder create(String hql) {
return new Finder(hql);
}
public Finder append(String hql) {
hqlBuilder.append(hql);
return this;
}
/**
* 获得原始hql语句
*
* @return
*/
public String getOrigHql() {
return hqlBuilder.toString();
}
/**
* 获得查询数据库记录数的hql语句。
*
* @return
*/
public String getRowCountHql() {
String hql = hqlBuilder.toString();
int fromIndex = hql.toLowerCase().indexOf(FROM);
String projectionHql = hql.substring(0, fromIndex);
hql = hql.substring(fromIndex);
String rowCountHql = hql.replace(HQL_FETCH, "");
int index = rowCountHql.indexOf(ORDER_BY);
if (index > 0) {
rowCountHql = rowCountHql.substring(0, index);
}
return wrapProjection(projectionHql) + rowCountHql;
}
public int getFirstResult() {
return firstResult;
}
public void setFirstResult(int firstResult) {
this.firstResult = firstResult;
}
public int getMaxResults() {
return maxResults;
}
public void setMaxResults(int maxResults) {
this.maxResults = maxResults;
}
/**
* 是否使用查询缓存
*
* @return
*/
public boolean isCacheable() {
return cacheable;
}
/**
* 设置是否使用查询缓存
*
* @param cacheable
* @see Query#setCacheable(boolean)
*/
public void setCacheable(boolean cacheable) {
this.cacheable = cacheable;
}
/**
* 设置参数
*
* @param param
* @param value
* @return
* @see Query#setParameter(String, Object)
*/
public Finder setParam(String param, Object value) {
return setParam(param, value, null);
}
/**
* 设置参数。与hibernate的Query接口一致。
*
* @param param
* @param value
* @param type
* @return
* @see Query#setParameter(String, Object, Type)
*/
public Finder setParam(String param, Object value, Type type) {
getParams().add(param);
getValues().add(value);
getTypes().add(type);
return this;
}
/**
* 设置参数。与hibernate的Query接口一致。
*
* @param paramMap
* @return
* @see Query#setProperties(Map)
*/
public Finder setParams(Map paramMap) {
for (Map.Entry entry : paramMap.entrySet()) {
setParam(entry.getKey(), entry.getValue());
}
return this;
}
/**
* 设置参数。与hibernate的Query接口一致。
*
* @param name
* @param vals
* @param type
* @return
*/
public Finder setParamList(String name, Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy