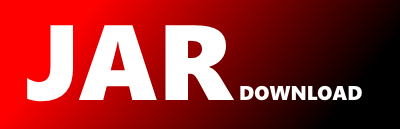
com.quhaodian.imake.fields.FormBeanConver Maven / Gradle / Ivy
package com.quhaodian.imake.fields;
import com.quhaodian.data.annotations.FormAnnotation;
import com.quhaodian.data.annotations.FormFieldAnnotation;
import org.apache.commons.lang3.StringUtils;
import java.lang.reflect.Field;
import java.util.List;
public class FormBeanConver {
public FormBean converClass(Class> object) {
FormBean formBean = new FormBean();
List beanList = formBean.getFields();
FormAnnotation anno = object.getAnnotation(FormAnnotation.class);
if (anno != null) {
formBean.setTitle(anno.title());
formBean.setAdd(anno.add());
formBean.setUpdate(anno.update());
formBean.setList(anno.list());
}
for (Class> clazz = object; clazz != Object.class; clazz = clazz.getSuperclass()) {
Field[] fs = clazz.getDeclaredFields();
for (Field f : fs) {
f.setAccessible(true);
if (f.getName().equals("id")) {
continue;
}
FormFieldAnnotation annotation = f.getAnnotation(FormFieldAnnotation.class);
FieldBean bean = new FieldBean();
if (annotation == null) {
continue;
}
if (annotation.ignore()) {
continue;
}
bean.setClassName(annotation.className());
bean.setId(annotation.id());
if (bean.getId() == null) {
bean.setId(f.getName());
}
bean.setType(annotation.type().name());
bean.setPlaceholder(annotation.placeholder());
Integer sortNum = getInteger(annotation);
bean.setSortNum(sortNum);
bean.setTitle(annotation.title());
bean.setCol(annotation.col()+"");
if (annotation.grid()) {
formBean.getGrids().add(bean);
}
if (StringUtils.isEmpty(bean.getTitle())) {
bean.setTitle(f.getName());
}
if (StringUtils.isEmpty(bean.getId())) {
bean.setId(f.getName());
}
if (StringUtils.isEmpty(bean.getPlaceholder())) {
bean.setPlaceholder(bean.getTitle());
}
if ("date".equals(bean.getType())) {
formBean.setHasDate(true);
formBean.getDates().add(bean);
}
if ("image".equals(bean.getType())) {
formBean.setHasImage(true);
formBean.getImages().add(bean);
}
beanList.add(bean);
}
}
return formBean;
}
public FormBean conver(Object object) {
return converClass(object.getClass());
}
private Integer getInteger(FormFieldAnnotation annotation) {
Integer result = 0;
String b = annotation.sortNum();
try {
result = Integer.parseInt(b);
} catch (Exception e) {
result = 0;
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy