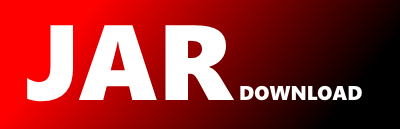
com.quinsoft.zeidon.View Maven / Gradle / Ivy
The newest version!
/**
This file is part of the Zeidon Java Object Engine (Zeidon JOE).
Zeidon JOE is free software: you can redistribute it and/or modify
it under the terms of the GNU Lesser General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
Zeidon JOE is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public License
along with Zeidon JOE. If not, see .
Copyright 2009-2015 QuinSoft
*/
package com.quinsoft.zeidon;
import java.io.Writer;
import java.util.Collection;
import java.util.EnumSet;
import java.util.Set;
import com.quinsoft.zeidon.objectdefinition.EntityDef;
import com.quinsoft.zeidon.objectdefinition.LodDef;
/**
* A View is the main interface for manipulating Zeidon data organized in Object Instances
* (OIs). A view is a set of EntityCursors, one for each entity specified in the LOD.
* The EntityCursor is used to scan through existing Entity Instances in an OI.
*
*/
public interface View extends TaskQualification
{
final static long DISPLAY_HIDDEN = 0x00000001;
final static long DISPLAY_EMPTY_ATTRIBS = 0x00000002;
/**
* Returns the application for this View. The logic is as follows:
*
* 1) Gets the application of the LodDef. If this application is not ZeidonSystem,
* then it returns the application.
* 2) If the LodDef belongs to ZeidonSystem, then the default application of the
* parent task is returned.
*/
@Override
Application getApplication();
/**
* Returns the LodDef for this View.
*
* @return the LodDef for this view.
*/
LodDef getLodDef();
/**
* Returns 'true' if this View is read-only. A read-only view can not be used
* to update the underlying OI. It is possible for a different view to update
* the same OI.
*
* @return true if this View is read-only.
*/
boolean isReadOnly();
/**
* Used to set the read-only flag for this view.
*
* @param readOnly if 'true' then this View will be read-only.
*/
void setReadOnly( boolean readOnly );
/**
* If true then allow cursors to refer to hidden entities without throwing
* an exception. Intended to be used by DBHandlers.
*
* @return true if the view can reference hidden (e.g. deleted) entities.
*/
boolean isAllowHiddenEntities();
/**
* If set to true then this view can reference hidden entities without throwing
* an exception. Intended to be used by DBHandlers.
*
* @param allowHiddenEntities
*
* @return the previous value of allowHiddenEntities.
*/
boolean setAllowHiddenEntities( boolean allowHiddenEntities );
/**
* Returns an ID for the view that is unique for the JVM.
*
* @return unique key.
*/
long getId();
/**
* Return an ID that is unique for this OI. Different views that reference the
* same OI will return the same OI ID.
*
* @return
*/
long getOiId();
/**
* Returns the EntityCursor for the entity specified by name.
*
* @param entityName name of the entity.
*
* @return the EntityCursor.
*/
EntityCursor cursor( String entityName );
/**
* Returns the EntityCursor for the entity specified by EntityDef.
*
* @param entityDef definition of the entity.
*
* @return the EntityCursor.
*/
EntityCursor cursor( EntityDef entityDef );
/**
* A convenience method to retrieve the cursor for the root entity.
*
* @return EntityCursor for the root entity.
*/
EntityCursor root();
/**
* Synonym for cursor( String entityName );
*
* @param entityName name of the entity.
*
* @return the EntityCursor.
*/
EntityCursor getCursor( String entityName );
/**
* Synonym for cursor( EntityDef entityDef ).
*
* @param entityDef definition of the entity.
* @return the EntityCursor.
*/
EntityCursor getCursor( EntityDef entityDef );
/**
* @return The default select set. If one doesn't exist then it will be created
* and stored in an internal hash set.
*/
SelectSet getSelectSet();
/**
* Returns the select set by index. If one doesn't exist then it will be created
* and stored in an internal hash set.
*
* @param index.
* @return
*/
SelectSet getSelectSet( Object index );
/**
* Returns a list of current select set names for this view.
*
* @return
*/
Set
© 2015 - 2024 Weber Informatics LLC | Privacy Policy