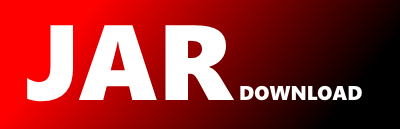
com.quinsoft.zeidon.standardoe.ObjectInstanceComparer Maven / Gradle / Ivy
The newest version!
/**
This file is part of the Zeidon Java Object Engine (Zeidon JOE).
Zeidon JOE is free software: you can redistribute it and/or modify
it under the terms of the GNU Lesser General Public License as published by
the Free Software Foundation, either version 3 of the License, or
(at your option) any later version.
Zeidon JOE is distributed in the hope that it will be useful,
but WITHOUT ANY WARRANTY; without even the implied warranty of
MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
GNU Lesser General Public License for more details.
You should have received a copy of the GNU Lesser General Public License
along with Zeidon JOE. If not, see .
Copyright 2009-2015 QuinSoft
*/
package com.quinsoft.zeidon.standardoe;
import java.io.PrintWriter;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.apache.commons.lang3.StringUtils;
import com.quinsoft.zeidon.Task;
import com.quinsoft.zeidon.ZeidonException;
import com.quinsoft.zeidon.objectdefinition.AttributeDef;
import difflib.Delta;
import difflib.Delta.TYPE;
import difflib.DiffUtils;
import difflib.Patch;
/**
* Compares two OIs and determines if they are the same.
*
* Some day this will create a "patch" type result that can be used to see what
* is different between the OIs.
*
* @author DG
*
*/
class ObjectInstanceComparer
{
private static final Map COLORS =
Collections.unmodifiableMap( new HashMap() {
private static final long serialVersionUID = 1L;
{
put( TYPE.CHANGE, "yellow" );
put( TYPE.DELETE, "red" );
put( TYPE.INSERT, "lightgreen" );
}} );
final private ObjectInstance oi1;
final private ObjectInstance oi2;
private List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy