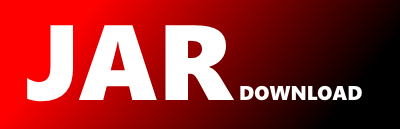
com.quotemedia.streamer.client.NewsSubscription Maven / Gradle / Ivy
Show all versions of streamerclient-java-core Show documentation
package com.quotemedia.streamer.client;
import com.quotemedia.streamer.messages.control.NewsFilter;
/**
* Subscription request for news data.
*
* Instances of this class can be created using {@link NewsSubscription.Builder}.
*
*/
public class NewsSubscription {
private NewsFilter[] newsFilters;
private Boolean skipHeavyInitialLoad;
public NewsFilter[] getNewsFilters() {
return newsFilters;
}
public boolean skipHeavyInitialLoad() {
return skipHeavyInitialLoad != null ? skipHeavyInitialLoad : false;
}
public static final class Builder {
private NewsFilter[] newsFilters;
private boolean skipHeavyInitialLoad = false;
/**
* Sets the news filters to subscribe for.
* .
*
* @param value the news filters
* @return a reference to this object
*/
public final NewsSubscription.Builder newsFilters(final NewsFilter... value) {
this.newsFilters = value;
return this;
}
/**
* Sets whether to skip heavy initial loads.
*
* @param value whether to skip heavy initial loads.
* @return a reference to this object
*/
public final NewsSubscription.Builder skipHeavyInitialLoad(final boolean value) {
this.skipHeavyInitialLoad = value;
return this;
}
public final NewsSubscription build() {
final NewsSubscription obj = new NewsSubscription();
obj.newsFilters = this.newsFilters;
obj.skipHeavyInitialLoad = this.skipHeavyInitialLoad;
return obj;
}
}
}