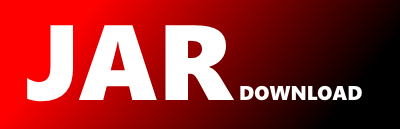
com.quotemedia.streamer.client.Stream Maven / Gradle / Ivy
Show all versions of streamerclient-java-core Show documentation
package com.quotemedia.streamer.client;
import com.quotemedia.streamer.messages.control.*;
/**
* A bidirectional connection to the streaming service server.
* Instances can be created using {@link com.quotemedia.streamer.client.StreamFactory}.
*
Opening a stream establishes a connection to the server. Once the stream is open data reception
* can be enabled/disabled by subscribing/unsubscribing. If the stream isn't used anymore it should be closed
* to ensure resources are released properly.
*
How to use streams see {@link com.quotemedia.streamer.client} description.
*
* @see com.quotemedia.streamer.client.StreamFactory
*/
public interface Stream {
/**
* Sets callback to handle received messages.
*
* @param callback called when a message is received.
*/
void onmessage(final OnMessage> callback);
/**
* Sets callback to handle errors.
*
* @param callback called when an error occurs during streaming
*/
void onerror(final OnError callback);
/**
* Sets the callback to be used when a reconnect response is received
* @param callback when reconnected
* */
void onReconnect(final OnReconnect callback);
/**
* Sets callback to handle received ctrl messages.
*
* @param callback called when a message is received.
*/
void onctrlmessage(final OnMessage> callback);
/**
* Opens a connection to the server.
*
* This call will block until either the stream could be opened or the attempt failed.
*
* @param cfg parameters specifying this streams configuration.
* @return response when stream is open. Response must be checked for eventual limitations.
* @throws StreamException if stream could not be opened
* @throws java.lang.IllegalStateException if stream cannot be opened in its current state
*/
ConnectResponse open(final StreamCfg cfg) throws StreamException;
/**
* Closes this stream.
*
* This call will return immediately. Callback will be called asynchronous once the stream is closed.
*
* @param callback called when this stream is closed
*/
void close(final OnClose callback);
/**
* Manually reopens a connection.
*
* This call will manually perform a reconnection regardless of the close. When this method is call, you will need to listen to
* - ConnectResponse: check that the connection was successfully establish
* - ReconnectResponse: if previous disconnected session had any subscriptions this message will be sent once all those symbols are
* resubscribed
* - MissedDataSent: If any of the missed data flags is on, this message will be sent once all the missed data is sent to the client
*
* @throws StreamException if any exception is thrown during reconnecting
* */
void performReconnect() throws StreamException;
/**
* Requests data about available and current numbers of symbols and connections.
*
* This call will return immediately. Callback will be called asynchronous once the response arrives
* or the request failed.
*
* @param callback called when stats response is received from server
* @throws java.lang.IllegalStateException if this stream cannot request stats in its current state
*/
void getSessionStats(final OnResponse callback);
/**
* Returns this streams state.
*
* @return the current state
*/
Stream.STATE state();
/**
* Subscribes to streaming data specified by request.
*
* This call will return immediately. Callback will be called asynchronous once the response arrives
* or the request failed.
*
* @param request subscribe request to send to the server
* @param callback called when subscribe response is received from server
* @throws java.lang.IllegalStateException if this stream cannot subscribe in its current state
*/
void subscribe(final Subscription request, final OnResponse callback);
/**
* Unsubscribes from streaming data specified by request.
*
* This call will return immediately. Callback will be called asynchronous once the response arrives
* or the request failed.
*
* @param request unsubscribe request to send to the server
* @param callback called when unsubscribe response is received from server
* @throws java.lang.IllegalStateException if this stream cannot unsubscribe in its current state
*/
void unsubscribe(final Subscription request, final OnResponse callback);
void subUnsubAlert(final AlertSubscription request, final OnResponse callback);
/**
* Subscribes to streaming data specified by request.
*
* This call will return immediately. Callback will be called asynchronous once the response arrives
* or the request failed.
*
* @param request subscribe request to send to the server
* @param callback called when subscribe response is received from server
* @throws java.lang.IllegalStateException if this stream cannot subscribe in its current state
*/
void subscribeExchange(final ExchangeSubscription request, final OnResponse callback);
/**
* Unsubscribes from streaming data specified by request.
*
* This call will return immediately. Callback will be called asynchronous once the response arrives
* or the request failed.
*
* @param request unsubscribe request to send to the server
* @param callback called when unsubscribe response is received from server
* @throws java.lang.IllegalStateException if this stream cannot unsubscribe in its current state
*/
void unsubscribeExchange(final ExchangeSubscription request, final OnResponse callback);
/**
* Subscribes to streaming data specified by request.
*
* This call will return immediately. Callback will be called asynchronous once the response arrives
* or the request failed.
*
* @param request subscribe request to send to the server
* @param callback called when subscribe response is received from server
* @throws java.lang.IllegalStateException if this stream cannot subscribe in its current state
*/
void subscribeCorporateEvent(final CorpEventSubscription request, final OnResponse callback);
/**
* Unsubscribes from streaming data specified by request.
*
* This call will return immediately. Callback will be called asynchronous once the response arrives
* or the request failed.
*
* @param request unsubscribe request to send to the server
* @param callback called when unsubscribe response is received from server
* @throws java.lang.IllegalStateException if this stream cannot unsubscribe in its current state
*/
void unsubscribeCorporateEvent(final CorpEventSubscription request, final OnResponse callback);
/**
* The transport determines how the underlying bidirectional connection between the client and the server is established.
*/
enum TRANSPORT {
/**
* Streaming connection is established using websockets.
* WebSocket
*/
WEBSOCKET,
/**
* Streaming connection is established using a 'persistent' http request to receive data from the server
* and a separate request for sending data to the server.
*/
STREAMING;
// LONG_POLLING not supported
// SSE covered by STREAMING
}
/**
* Possible stream states.
*/
enum STATE {
/**
* The stream is newly created and hasn't been opened yet.
*/
NEW,
/**
* The stream is in transition to be open, but not open yet.
*/
OPENING,
/**
* The stream is open.
*/
OPEN,
/**
* The stream is in transition to be reopen, but not open yet.
*/
REOPENING,
/**
* The stream is in transition to be closed, but not closed yet.
*/
CLOSING,
/**
* The stream is closed.
*/
CLOSED,
/**
* The stream is broken.
*
* A broken stream should be closed and disposed of.
*
* Under some circumstances it is possible to recover a broken stream by closing and reopening it.
*/
BROKEN
}
/**
* Available conflation rates.
*/
interface CONFLATION {
/**
* No conflation.
*/
Integer NONE = 0;
/**
* Default conflation rate as determined by server.
*/
Integer DEFAULT = null;
}
/**
* Available conflation rates.
*/
interface INTERVAL_PERIOD {
/**
* No conflation.
*/
Integer TEN_SECOND = 10000;
/**
* Default conflation rate as determined by server.
*/
Integer DEFAULT = 60000;
}
int CONSOLIDATED_SYMBOL_ENTITLEMENTS_COEFFICIENT = 14;
String CONSOLIDATED_SYMBOL_SUFFIX = ":CC";
}