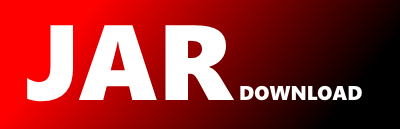
com.quotemedia.streamer.client.StreamCfg Maven / Gradle / Ivy
package com.quotemedia.streamer.client;
import com.quotemedia.streamer.messages.MimeTypes;
/**
* Parameters required when opening a stream.
*
* Instances of this class can be created using {@link StreamCfg.Builder}.
*
* @see Stream#open
*/
public final class StreamCfg {
private StreamCfg() {
}
private Auth auth;
/**
* Credentials used to authenticate client.
*
* @return the authentication parameters
*/
public final Auth auth() {
return this.auth;
}
private String uri;
/**
* Server uri to connect to.
*
* @return the streaming service uri
*/
public final String uri() {
return this.uri;
}
private Stream.TRANSPORT transport;
/**
* Transport to use for the stream.
*
* @return the transport to use
*/
public final Stream.TRANSPORT transport() {
return this.transport;
}
/**
* Default value for open timeout in seconds;
*/
public static Integer OPENTIMEOUT_S_DEFAULT = 5;
private Integer opentimeout_s;
/**
* Timeout to wait when opening a stream. This timeout ensures {@link Stream#open} won't block indefinitely.
*
* @return the timeout in seconds
*/
public final Integer opentimeout_s() {
return this.opentimeout_s;
}
/**
* Default value for reopen timeout in milliseconds;
*/
public static Integer REOPENTIMOUT_DEFAULT_MS = 5000;
private Integer reopentimeout_ms;
/**
* Timeout to wait when re-opening a stream.
* @return the timeout in milliseconds
*/
public final Integer reopentimeout_ms() {
return this.reopentimeout_ms;
}
public static Integer RECONNECT_DELAY_MS = 100;
private Integer reconnectdelay_ms;
public final Integer reconnectdelay_ms(){
return this.reconnectdelay_ms;
}
/**
* Default value for reopen timeout in milliseconds;
*/
public static Integer REOPEN_MAX_ATTEMPTS_DEFAULT = 3;
public static Integer MAX_PERMITTED_ATTEMPTS = 10;
private Integer reopenMaxAttempts;
/**
* Timeout to wait when re-opening a stream.
* @return the timeout in milliseconds
*/
public final Integer getReopenMaxAttempts() {
return this.reopenMaxAttempts;
}
public static boolean CHECK_SERVER_ON_RECONNECT_DEFAULT = false;
private boolean isCheckServerOnReconnect;
/**
* Checks if the server is up before attempting a reconnection.
* @return whether to check or not
* */
public boolean isCheckServerOnReconnect() {
return this.isCheckServerOnReconnect;
}
public static boolean ALWAYS_REOPEN_DEFAULT = false;
private boolean isAlwaysReopen;
/**
* Boolean value to never completely close a stream. If true, when a regular close is
* triggered, the reopen connection will still try to reconnect to the streaming service
* @return boolean value for always reopen
* **/
public final Boolean isAlwaysReopen(){
return this.isAlwaysReopen;
}
public static boolean RECEIVE_ALL_MISSED_DATA = false;
private boolean isReceiveAllMissedData;
/**
* Boolean value to receive missed all data once reconnected
* @return boolean value to receive all missed data
* **/
public final Boolean isReceiveAllMissedData(){
return this.isReceiveAllMissedData;
}
public static boolean RECEIVE_LATEST_MISSED_DATA = false;
private boolean isReceiveLatestMissedData;
/**
* Boolean value to receive few latest missed data once reconnected
* @return boolean value to receive latest missed data
* **/
public final Boolean isReceiveLatestMissedData(){
return this.isReceiveLatestMissedData;
}
public static String STOMP_WMID = "";
private String stompWmid;
/**
* String value wmid using for Stomp connection
* @return stompWmid
*/
public final String stompWmid() {
return this.stompWmid;
}
/**
* String value to store the where the connection is come from, we default it come from the external
* mainly header is design for QS2 to open and hold the Dividend messages connection.
*/
public static String CONNECTION_FROM_DEFAULT = "external";
private String connectionFrom;
public final String connectionFrom() {
return this.connectionFrom;
}
/**
* Default value for synchronous message processing flag;
*/
public static boolean IS_SYNCHRONOUS_MESSAGE_PROCESSING_DEFAULT = false;
private boolean isSynchronousMessageProcessing;
/**
* Value determines whether to process messages synchronously. When is set to false, insures {@link Stream#subscribe(Subscription, OnResponse)} won't be blocked
* waiting for response, when message processing was blocked.
*
* @return the value of the flag
*/
public final boolean isSynchronousMessageProcessing() {
return this.isSynchronousMessageProcessing;
}
public static boolean IS_RECONNECT_ACTIVE_DEFAULT = false;
private Boolean isReconnectActive;
public final Boolean isReconnectActive() {
return this.isReconnectActive;
}
/**
* Default queue capacity value for asynchronous message processing;
*/
public static int QUEUE_CAPACITY_DEFAULT = 200000;
private int queueCapacity;
/**
* Limits queue size for asynchronous message processing to protect client from having too many messages queued up for for processing
*
* @return the queue capacity value
*/
public final int queueCapacity() {
return this.queueCapacity;
}
private Integer conflation_ms;
/**
* Conflation rate in milliseconds.
*
* @return the conflation rate in milliseconds
* @see com.quotemedia.streamer.client.Stream.CONFLATION
*/
public final Integer conflation_ms() {
return this.conflation_ms;
}
private Integer interval_period_ms;
/**
* Interval period rate in milliseconds.
*
* @return the interval period rate in milliseconds
* @see com.quotemedia.streamer.client.Stream.INTERVAL_PERIOD
*/
public final Integer interval_period_ms() {
return this.interval_period_ms;
}
private Boolean rejectExcessiveConnection;
/**
* Returns whether to reject connections over the connection limit
*
* @return whether to reject connections over the connection limit.
*/
public Boolean rejectExcessiveConnection() {
return rejectExcessiveConnection;
}
/**
* Default streaming data format used.
*/
public static final String FORMAT_DEFAULT = MimeTypes.QMCI;
private String format;
/**
* Streaming data format.
*
* @return format identifier
* @see MimeTypes
*/
public final String format() {
return this.format;
}
/**
* Default receive buffer size in bytes
*/
public static final int RXBUFFERSIZE_DEFAULT = 4096;
private int rxbuffersize;
/**
* Receive buffer size in bytes.
*
* @return the receive buffer size in bytes
*/
public int rxbuffersize() {
return this.rxbuffersize;
}
/**
* Default send buffer size in bytes.
*/
public static final int TXBUFFERSIZE_DEFAULT = 4096;
private int txbuffersize;
/**
* Send buffer size in bytes.
*
* @return the send buffer size in bytes
*/
public int txbuffersize() {
return this.txbuffersize;
}
/**
* Default boolean to check server version on connection open.
*/
public static final boolean CHECK_VERSION_DEFAULT = true;
private boolean checkVersion;
/**
* Send buffer size in bytes.
*
* @return the send buffer size in bytes
*/
public boolean checkversion() {
return this.checkVersion;
}
/**
* Builder to create immutable instances of {@link StreamCfg} with fluent api.
*/
public static final class Builder {
private Auth auth;
/**
* Sets the authentication parameters.
*
* @param val the parameters
* @return a reference to this object
*/
public final Builder auth(final Auth val) {
this.auth = val;
return this;
}
private String uri;
/**
* Sets the server uri.
*
* @param val the server uri
* @return a reference to this object
*/
public final Builder uri(final String val) {
this.uri = val;
return this;
}
private Stream.TRANSPORT transport = Stream.TRANSPORT.WEBSOCKET;
/**
* Sets the stream transport.
*
* @param val the stream transport
* @return a reference to this object
*/
public final Builder transport(final Stream.TRANSPORT val) {
this.transport = val;
return this;
}
private Integer opentimeout_s = OPENTIMEOUT_S_DEFAULT;
/**
* Sets the open timeout.
*
* @param val the open timeout in seconds
* @return a reference to this object
*/
public final Builder opentimeout_s(final Integer val) {
this.opentimeout_s = val;
return this;
}
private Integer reopentimeout_ms = REOPENTIMOUT_DEFAULT_MS;
/**
* Sets the reopen timeout.
*
* @param val the reopen timeout in milliseconds
* @return a reference to this object
*/
public final Builder reopentimeout_ms(final Integer val) {
this.opentimeout_s = val;
return this;
}
private Integer reconnectdelay_ms = RECONNECT_DELAY_MS;
public final Builder reconnectdelay_ms(final Integer val){
this.reconnectdelay_ms = val;
return this;
}
private Integer reopenMaxAttempts = REOPEN_MAX_ATTEMPTS_DEFAULT;
/**
* Sets the max number of reconnect attempts.
*
* @param val the max number of attempts
* @return a reference to this object
*/
public final Builder reopenMaxAttempts(final Integer val) {
this.reopenMaxAttempts = val;
return this;
}
private Boolean checkServerOnReconnect = CHECK_SERVER_ON_RECONNECT_DEFAULT;
public final Builder checkServerOnReconnect(final Boolean val){
this.checkServerOnReconnect = val;
return this;
}
private Boolean isReconnectActive = IS_RECONNECT_ACTIVE_DEFAULT;
public final Builder isReconnectActive(final Boolean val){
this.isReconnectActive = val;
return this;
}
private Boolean isAlwaysReopen = ALWAYS_REOPEN_DEFAULT;
public final Builder isAlwaysReopen(final Boolean val){
this.isAlwaysReopen = val;
return this;
}
private Boolean isReceiveAllMissedData = RECEIVE_ALL_MISSED_DATA;
public final Builder isReceiveAllMissedData(final Boolean val){
this.isReceiveAllMissedData = val;
return this;
}
private Boolean isReceiveLatestMissedData = RECEIVE_LATEST_MISSED_DATA;
public final Builder isReceiveLatestMissedData(final Boolean val){
this.isReceiveLatestMissedData = val;
return this;
}
private String stompWmid = STOMP_WMID;
public final Builder stompWmid(final String val) {
this.stompWmid = val;
return this;
}
public String connectionFrom = CONNECTION_FROM_DEFAULT;
public final Builder connectionFrom(final String val) {
this.connectionFrom = val;
return this;
}
private boolean isSynchronousMessageProcessing = IS_SYNCHRONOUS_MESSAGE_PROCESSING_DEFAULT;
/**
* Sets value of synchronous message processing flag.
*
* @param val boolean value, which determines whether messages will be processed synchronously
* @return a reference to this object
*/
public final Builder isSynchronousMessageProcessing(final boolean val) {
this.isSynchronousMessageProcessing = val;
return this;
}
private int queueCapacity = QUEUE_CAPACITY_DEFAULT;
/**
* Sets the value of queue capacity for asynchronous message processing.
*
* @param val queue capacity
* @return a reference to this object
*/
public final Builder queueCapacity(final int val) {
this.queueCapacity = val;
return this;
}
private Integer conflation_ms = Stream.CONFLATION.DEFAULT;
/**
* Set the conflation rate in milliseconds.
*
* @param val the conflation rate in milliseconds
* @return a reference to this object
* @see com.quotemedia.streamer.client.Stream.CONFLATION
*/
public final Builder conflation_ms(final Integer val) {
this.conflation_ms = val;
return this;
}
private Integer interval_period_ms = Stream.INTERVAL_PERIOD.DEFAULT;
/**
* Set the interval period in milliseconds.
*
* @param val the interval period rate in milliseconds
* @return a reference to this object
* @see com.quotemedia.streamer.client.Stream.CONFLATION
*/
public final Builder interval_period_ms(final Integer val) {
this.interval_period_ms = val;
return this;
}
private Boolean rejectExcessiveConnection = false;
/**
* Sets whether to reject connections over the connection limit
*
* @param val determines whether to reject connections over the connection limit
* @return a reference to this object
*/
public final Builder rejectExcessiveConnection(final Boolean val) {
this.rejectExcessiveConnection = val;
return this;
}
private Boolean checkVersion = CHECK_VERSION_DEFAULT;
/**
* Sets whether to check server version on connection open
* @param val determines whether to check server version on connection open
* @return a reference to this object
*/
public final Builder checkVersion(final Boolean val) {
this.checkVersion = val;
return this;
}
private String format = StreamCfg.FORMAT_DEFAULT;
/**
* Set the streaming data format.
*
* @param val the format identifier
* @return a reference to this object
* @see MimeTypes
*/
public Builder format(final String val) {
this.format = val;
return this;
}
/**
* Creates a {@code StreamCfg} instance from set values.
*
* @return the crated instance
*/
public StreamCfg build() {
final StreamCfg obj = new StreamCfg();
obj.auth = this.auth;
obj.uri = this.uri;
obj.transport = this.transport;
obj.opentimeout_s = this.opentimeout_s;
obj.reopentimeout_ms = this.reopentimeout_ms;
obj.reconnectdelay_ms = this.reconnectdelay_ms;
obj.reopenMaxAttempts = this.reopenMaxAttempts;
obj.isCheckServerOnReconnect = this.checkServerOnReconnect;
obj.conflation_ms = this.conflation_ms;
obj.interval_period_ms = this.interval_period_ms;
obj.format = this.format;
obj.rxbuffersize = StreamCfg.RXBUFFERSIZE_DEFAULT;
obj.txbuffersize = StreamCfg.TXBUFFERSIZE_DEFAULT;
obj.checkVersion = this.checkVersion;
obj.rejectExcessiveConnection = this.rejectExcessiveConnection;
obj.isSynchronousMessageProcessing = this.isSynchronousMessageProcessing;
obj.isReconnectActive = this.isReconnectActive;
obj.queueCapacity = this.queueCapacity;
obj.isAlwaysReopen = this.isAlwaysReopen;
obj.isReceiveAllMissedData = this.isReceiveAllMissedData;
obj.isReceiveLatestMissedData = this.isReceiveLatestMissedData;
obj.stompWmid = this.stompWmid;
obj.connectionFrom = this.connectionFrom;
return obj;
}
}
/**
* Base class for authentication parameters.
*/
public static abstract class Auth {
}
/**
* Authentication parameters in the form of credentials.
*/
public static final class AuthCredentials extends Auth {
private final String webmasterid;
private final String username;
private final String password;
/**
* Creates authentication parameters with specified credentials.
*
* @param webmasterid the webmaster id used for authentication
* @param username the username used for authentication
* @param password the password used for authentication
*/
public AuthCredentials(final String webmasterid, final String username, final String password) {
this.webmasterid = webmasterid;
this.username = username;
this.password = password;
}
/**
* Returns the webmaster id.
*
* @return the webmaster id
*/
public final String webmasterid() {
return this.webmasterid;
}
/**
* Returns the username.
*
* @return the username
*/
public final String username() {
return this.username;
}
/**
* Returns the password.
*
* @return the password
*/
public final String password() {
return this.password;
}
@Override
public final String toString() {
final StringBuilder sb = new StringBuilder();
sb.append(this.getClass().getSimpleName());
sb.append("{");
sb.append("webmasterid: ").append(this.webmasterid);
sb.append(", username: ").append(this.username);
sb.append(", password: ").append(this.password);
sb.append("}");
return sb.toString();
}
}
/**
* Authentication parameters in the form of a session id.
* Authentication will only succeed if session id has been authenticated previously by other means.
* The intended use case for this authentication method is for clients handling authentication by them self.
*/
public static final class AuthSid extends Auth {
private final String sid;
/**
* Creates session id authentication parameter.
*
* @param sid session id
*/
public AuthSid(final String sid) {
this.sid = sid;
}
/**
* Returns the session id.
*
* @return the session id
*/
public final String sid() {
return this.sid;
}
@Override
public final String toString() {
final StringBuilder sb = new StringBuilder();
sb.append(this.getClass().getSimpleName());
sb.append("{");
sb.append("sid: ").append(this.sid);
sb.append("}");
return sb.toString();
}
}
/**
* Authentication parameters in the form of a webmaster id.
* Authentication will only succeed if webmaster id is valid and ip/domain the connection if made from has been
* registered for webmaster.
*/
public static final class AuthWmid extends Auth {
private final String wmid;
/**
* Creates a webmaster id authentication parameter.
*
* @param wmid the webmaster id
*/
public AuthWmid(final String wmid) {
this.wmid = wmid;
}
/**
* Returns the webmaster id.
*
* @return the webmaster id
*/
public final String wmid() {
return this.wmid;
}
@Override
public String toString() {
final StringBuilder str = new StringBuilder();
str.append(this.getClass().getSimpleName());
str.append("{");
str.append("wmid: ").append(this.wmid);
str.append("}");
return str.toString();
}
}
/**
* Authentication parameters in the form of a webmaster id.
* Authentication will only succeed if webmaster id is valid and ip/domain the connection if made from has been
* registered for webmaster.
*/
public static final class AuthEnterpriseToken extends Auth {
private final String token;
private final String wmid;
/**
* Creates a Enterprise token authentication parameter.
*
* @param token Enterprise token
* @param webmasterid also known as wmid, which is used for authentication
*/
public AuthEnterpriseToken(final String token, final String webmasterid) {
this.token = token;
this.wmid = webmasterid;
}
public final String wmid() {
return this.wmid;
}
/**
* Returns the token.
*
* @return the token
*/
public final String token() {
return this.token;
}
@Override
public String toString() {
final StringBuilder str = new StringBuilder();
str.append(this.getClass().getSimpleName());
str.append("{");
str.append("token: ").append(this.token);
str.append("}");
return str.toString();
}
}
}