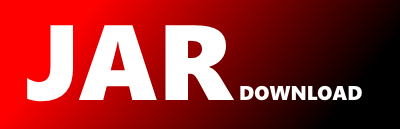
com.quotemedia.streamer.client.impl.FutureValue Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of streamerclient-java-core Show documentation
Show all versions of streamerclient-java-core Show documentation
Java streaming client that provides easy-to-use client APIs to connect and subscribe to QuoteMedia's market data streaming services. https://quotemedia.com/
The newest version!
package com.quotemedia.streamer.client.impl;
import java.util.concurrent.CountDownLatch;
import java.util.concurrent.ExecutionException;
import java.util.concurrent.Future;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.TimeoutException;
import java.util.concurrent.atomic.AtomicBoolean;
import java.util.concurrent.atomic.AtomicReference;
final class FutureValue implements Future {
private Object lock = new Object();
private final CountDownLatch oncomplete;
public FutureValue() {
this.oncomplete = new CountDownLatch(1);
}
// sync on lock object
private AtomicBoolean completed = new AtomicBoolean(false);
@Override
public final boolean isDone() {
return this.completed.get();
}
// sync on lock object
private final AtomicBoolean cancelled = new AtomicBoolean(false);
@Override
public final boolean isCancelled() {
return this.cancelled.get();
}
@Override
public final boolean cancel(final boolean interrupt) {
synchronized (this.lock) {
if (this.completed.get()) {
return false;
} else {
this.cancelled.set(true);
this.completed.set(true);
this.oncomplete.countDown();
return true;
}
}
}
// sync on lock object
private final AtomicReference value = new AtomicReference();
private final AtomicReference throwable = new AtomicReference();
@Override
public final V get() throws InterruptedException, ExecutionException {
this.oncomplete.await(); // do not hold lock when blocking
// implementation guarantees that state is immutable once latch triggered
return this.getresultorthrowexception();
}
@Override
public final V get(final long timeout, final TimeUnit unit)
throws InterruptedException, ExecutionException, TimeoutException {
final boolean timedout = !this.oncomplete.await(timeout, unit); // do not hold lock when blocking
if (timedout) {
throw new TimeoutException();
}
// implementation guarantees that state is immutable once latch triggered
return this.getresultorthrowexception();
}
private V getresultorthrowexception() throws ExecutionException {
if (this.failed.get()) {
throw new ExecutionException(this.throwable.get());
} else if (this.cancelled.get()) {
return null;
} else {
return this.value.get();
}
}
public final boolean complete(final V val) {
synchronized (this.lock) {
boolean success = false;
if (this.completed.get()) {
success = false;
} else {
this.value.set(val);
this.completed.set(true);
success = true;
}
this.oncomplete.countDown();
return success;
}
}
private final AtomicBoolean failed = new AtomicBoolean(false);
public final boolean fail(final Throwable t) {
synchronized (this.lock) {
boolean success = false;
if (this.completed.get()) {
success = false;
} else {
this.throwable.set(t);
this.failed.set(true);
this.completed.set(true);
success = true;
}
this.oncomplete.countDown();
return success;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy