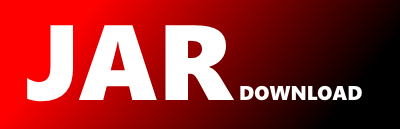
com.quotemedia.streamer.client.impl.StompPayloadDecoder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of streamerclient-java-core Show documentation
Show all versions of streamerclient-java-core Show documentation
Java streaming client that provides easy-to-use client APIs to connect and subscribe to QuoteMedia's market data streaming services. https://quotemedia.com/
The newest version!
package com.quotemedia.streamer.client.impl;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.quotemedia.streamer.messages.control.CtrlMessage;
import com.quotemedia.streamer.messages.market.DataMessage;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import static com.google.common.base.Preconditions.checkNotNull;
public class StompPayloadDecoder {
private final ObjectMapper json;
private final String textencoding;
public StompPayloadDecoder(final ObjectMapper json) {
checkNotNull(json);
this.json = json;
this.textencoding = "UTF-8"; // TODO make configurable
}
public Object decode(Object payload) throws IOException {
if (payload == null) {
return null;
}
Object decoded = read(decode(payload.toString()));
return decoded;
}
public byte[] decode(final String text) throws UnsupportedEncodingException {
return text != null ? text.getBytes(textencoding) : null;
}
public Object read(final byte[] bytes) throws IOException {
Object obj = null;
if (bytes != null) {
obj = json.readValue(bytes, CtrlMessage.class);
if (obj == null) {
obj = json.readValue(bytes, DataMessage.class);
}
}
return obj;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy