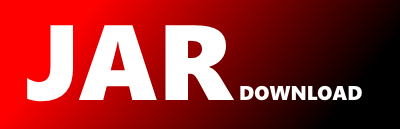
com.quotemedia.streamer.client.impl.TextPayloadDecoder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of streamerclient-java-core Show documentation
Show all versions of streamerclient-java-core Show documentation
Java streaming client that provides easy-to-use client APIs to connect and subscribe to QuoteMedia's market data streaming services. https://quotemedia.com/
The newest version!
package com.quotemedia.streamer.client.impl;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.quotemedia.streamer.messages.Encodings;
import com.quotemedia.streamer.messages.MimeTypes;
import com.quotemedia.streamer.messages.control.BaseResponse;
import com.quotemedia.streamer.messages.control.CtrlMessage;
import com.quotemedia.streamer.messages.qmci.QmciWireformat;
import com.quotemedia.streamer.messages.smessage.SMessage;
import org.apache.commons.codec.binary.Base64;
import java.io.IOException;
import java.io.UnsupportedEncodingException;
import java.nio.ByteBuffer;
import java.util.HashMap;
import java.util.Map;
import static com.google.common.base.Preconditions.checkNotNull;
public final class TextPayloadDecoder implements PayloadDecoder {
private final QmciMessageFactory qmci;
private final ObjectMapper json;
private final String textencoding;
private final Map decoders;
private final Map readers;
public TextPayloadDecoder(final QmciMessageFactory qmci, final ObjectMapper json) {
checkNotNull(qmci);
checkNotNull(json);
this.qmci = qmci;
this.json = json;
this.textencoding = "UTF-8"; // TODO make configurable
this.decoders = new HashMap<>();
this.decoders.put(Encodings.NONE_CHAR, new Decode() {
@Override
public byte[] decode(final String text) throws UnsupportedEncodingException {
return text != null ? text.getBytes(textencoding) : null;
}
});
this.decoders.put(Encodings.BASE64_CHAR, new Decode() {
@Override
public byte[] decode(final String text) throws UnsupportedEncodingException {
return text != null ? Base64.decodeBase64(text) : null;
}
});
this.readers = new HashMap<>();
this.readers.put(MimeTypes.JSON_CHAR, new Read() {
@Override
public Object read(final byte[] bytes) throws IOException {
return bytes != null ? json.readValue(bytes, CtrlMessage.class) : null;
}
});
this.readers.put(MimeTypes.QMCI_CHAR, new Read() {
@Override
public Object read(final byte[] bytes) throws IOException {
return bytes != null ? qmci.create(ByteBuffer.wrap(bytes).order(QmciWireformat.BYTE_ORDER)) : null;
}
});
}
/**
* Decodes message payload into an object. If no decoder for payload encoding, or reader for payload mime-type are
* available {@code null} is returned. This makes backward compatibility maintenance easier.
* Additional encodings or mime-types sent from server to client will simply be ignored.
*
* @param in message to decode
* @return the decoded object or {@code null} if encoding or mime-type is not supported
* @throws IOException if something goes wrong during decoding
*/
@Override
public final Object decode(final SMessage in) throws IOException {
if (in == null || in.payload() == null) {
return null;
}
final Decode d = this.decoders.get(in.encoding());
if (d == null) {
return null;
}
final Read read = this.readers.get(in.mimetype());
if (read == null) {
return null;
}
final Object decoded = read.read(d.decode(in.payload()));
if (decoded instanceof BaseResponse) { // @remove 1.0.6
((BaseResponse) decoded).setRequestId(in.id());
}
return decoded;
}
private interface Decode {
byte[] decode(final String payload) throws UnsupportedEncodingException;
}
private interface Read {
Object read(final byte[] bytes) throws IOException;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy