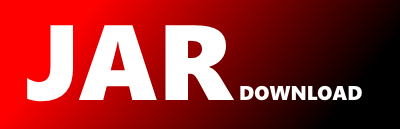
com.quotemedia.streamer.client.mapper.IntervalMsgImpl Maven / Gradle / Ivy
package com.quotemedia.streamer.client.mapper;
import com.quotemedia.datacache.data.Price;
import com.quotemedia.datacache.data.QMLongDecimal;
import com.quotemedia.streamer.messages.market.DataMessage;
import java.util.Date;
public class IntervalMsgImpl implements DataMessage {
private String symbol;
private int locateCode;
private Date timestamp;
private Price high;
private Price last;
private Long lastTime;
private Price low;
private Price open;
private Long openTime;
private Long startTime;
private Long tradeValue;
private QMLongDecimal volume;
private Price VWAP;
private Integer periodMs;
public String getSymbol() {
return symbol;
}
public void setSymbol(String symbol) {
this.symbol = symbol;
}
public int getLocateCode() {
return locateCode;
}
public void setLocateCode(int locateCode) {
this.locateCode = locateCode;
}
public Date getTimestamp() {
return timestamp;
}
public void setTimestamp(Date timestamp) {
this.timestamp = timestamp;
}
public Price getHigh() {
return high;
}
public void setHigh(Price high) {
this.high = high;
}
public Price getLast() {
return last;
}
public void setLast(Price last) {
this.last = last;
}
public Long getLastTime() {
return lastTime;
}
public void setLastTime(Long lastTime) {
this.lastTime = lastTime;
}
public Price getLow() {
return low;
}
public void setLow(Price low) {
this.low = low;
}
public Price getOpen() {
return open;
}
public void setOpen(Price open) {
this.open = open;
}
public Long getOpenTime() {
return openTime;
}
public void setOpenTime(Long openTime) {
this.openTime = openTime;
}
public Long getStartTime() {
return startTime;
}
public void setStartTime(Long startTime) {
this.startTime = startTime;
}
public Long getTradeValue() {
return tradeValue;
}
public void setTradeValue(Long tradeValue) {
this.tradeValue = tradeValue;
}
public QMLongDecimal getVolume() {
return volume;
}
public void setVolume(QMLongDecimal volume) {
this.volume = volume;
}
public Price getVWAP() {
return VWAP;
}
public void setVWAP(Price VWAP) {
this.VWAP = VWAP;
}
public Integer getPeriodMs() {
return periodMs;
}
public void setPeriodMs(Integer periodMs) {
this.periodMs = periodMs;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy