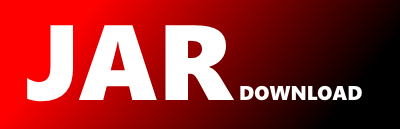
com.quotemedia.streamer.client.mapper.LastSaleMsgImpl Maven / Gradle / Ivy
package com.quotemedia.streamer.client.mapper;
import com.quotemedia.datacache.data.Price;
import com.quotemedia.datacache.data.QMLongDecimal;
import com.quotemedia.streamer.messages.market.DataMessage;
import java.util.Date;
public class LastSaleMsgImpl implements DataMessage {
private String symbol;
private int locateCode;
private Date timestamp;
private QMLongDecimal accumulatedVolume;
private Price change;
private Price last;
private Float percentChange;
private Price previousClose;
private Tick tick;
private String lastTradeExcode;
public String getSymbol() {
return symbol;
}
public void setSymbol(String symbol) {
this.symbol = symbol;
}
public Date getTimestamp() {
return timestamp;
}
public void setTimestamp(Date timestamp) {
this.timestamp = timestamp;
}
public QMLongDecimal getAccumulatedVolume() {
return accumulatedVolume;
}
public void setAccumulatedVolume(QMLongDecimal accumulatedVolume) {
this.accumulatedVolume = accumulatedVolume;
}
public int getLocateCode() {
return locateCode;
}
public void setLocateCode(int locateCode) {
this.locateCode = locateCode;
}
public Price getChange() {
return change;
}
public void setChange(Price change) {
this.change = change;
}
public Price getLast() {
return last;
}
public void setLast(Price last) {
this.last = last;
}
public Float getPercentChange() {
return percentChange;
}
public void setPercentChange(Float percentChange) {
this.percentChange = percentChange;
}
public Price getPreviousClose() {
return previousClose;
}
public void setPreviousClose(Price previousClose) {
this.previousClose = previousClose;
}
public Tick getTick() {
return tick;
}
public void setTick(Tick tick) {
this.tick = tick;
}
public String getLastTradeExcode() {
return lastTradeExcode;
}
public void setLastTradeExcode(String lastTradeExcode) {
this.lastTradeExcode = lastTradeExcode;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy